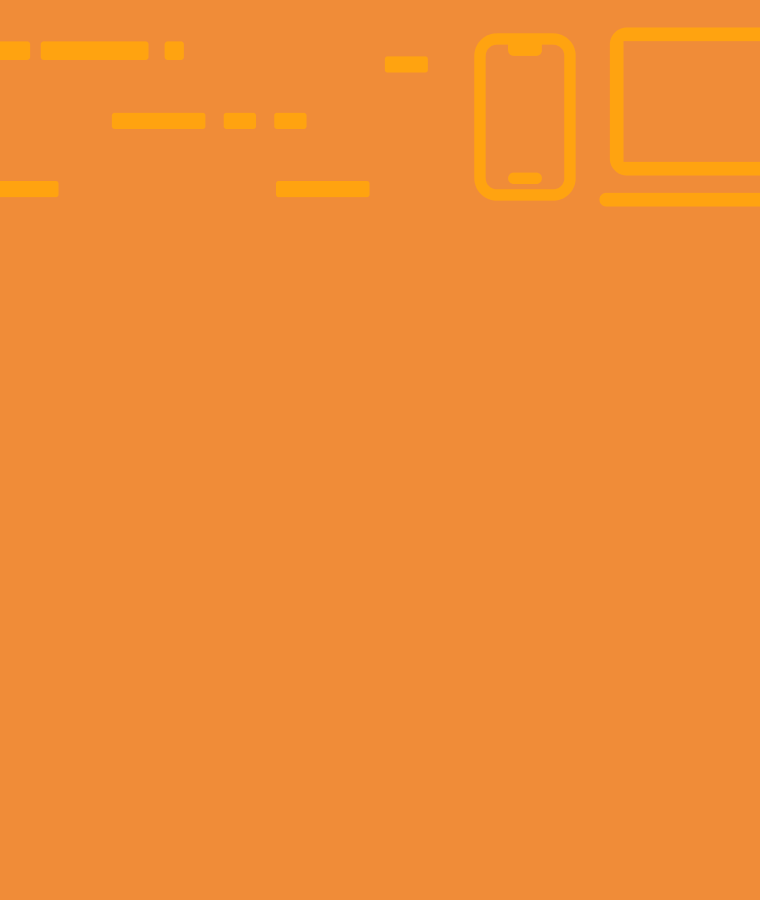
What Is Responsive Web Design?
In this article, we’ll look at responsive web design (RWD) in detail. By the end of the article, you’ll be able to make a website that your target audience can access on all screen sizes.
Definition
Responsive web design is the practice of designing and developing websites that respond to the user’s behavior on all screen sizes. These screen sizes in question include but are not limited to desktop, laptop, iPad, tablet, and mobile phone.
Why Responsive Design Matters
In the early times of the internet, people used to surf the net on desktop computers only. Then came mobile phones with the capability to view websites the way desktop and laptop computers can.
During those early times of the internet, web developers made one version of the website for computers and mobile phones. That was because internet users surf the net on computers (laptops and desktops). So there was no need to bother about responsive web design.
Decades later, beginning in 2010, responsive web design was born due to two reasons:
- people started accessing the internet from mobile phones and iPad more than computers
- the screen of a mobile phone is not as wide as that of desktop and laptop computers
Popular websites started making mobile versions and desktop versions of their websites. Then came the thought: why not create one website that would be accessible on both desktop/laptop computers and mobile phones?
That’s how responsive web design started. By 2015, responsive web design became necessary for every website.
Custom Layout Structure
For a better user experience, making a website’s layout respond to several screen sizes is important.
On desktop and laptop computers, some parts of the website would be on the sides, and some would stack on top of one another. On mobile phones, every part of the website has to stack on top of one another. To achieve this, developers can use different CSS files. But these days, developers use CSS media queries for this purpose.
If you are wondering what CSS media query is, it is a CSS technique with which you can modify the layout of your website in response to different screen sizes and prints.
The code below represents a layout structure that stacks on mobile phones and has some parts beside one another on a desktop.
Here’s the HTML:
<body> <div class="wrapper"> <header> <h1>Header</h1> </header> <aside class="sidebar sidebar1"> <h1>Sidebar 1</h1> </aside> <article class="main"> <h1>Main Content</h1> <p> Lorem ipsum dolor sit amet consectetu adipisicing elit. Accusamus, dolores. </p> </article> <aside class="sidebar sidebar2"> <h1>Sidebar 2</h1> </aside> <footer> <h1>Footer</h1> </footer> </div> </body>
Here’s the CSS:
* { margin: 0; padding: 0; box-sizing: border-box; font-family: "Gill Sans", "Gill Sans MT", Calibri, "Trebuchet MS", sans-serif; } .wrapper { display: flex; flex-flow: row wrap; } .wrapper > * { flex: 1 100%; } header { background-color: #2ecc71; height: 120px; display: flex; align-items: center; justify-content: center; } footer { background-color: #333; height: 120px; display: flex; align-items: center; justify-content: center; } .main { text-align: left; background-color: crimson; height: 555px; display: flex; align-items: center; justify-content: center; flex-direction: column; } .sidebar1, .sidebar2 { background-color: #3498db; display: flex; align-items: center; justify-content: center; } @media all and (min-width: 800px) { .main { flex: 3; } .sidebar1 { order: 1; } .sidebar2 { order: 2; } .main { order: 2; } footer { order: 4; } } @media all and (min-width: 600px) { .sidebar { flex: 1; } }
And here’s how it looks in the browser:

Multicol
Multicol is an experimental HTML tag (<muticol>) that was used to create multiple layouts right inside the HTML file. It is now deprecated (it is still available but not recommended for use anymore).
Multicol got deprecated because you can now use the columns property of CSS to do the same thing.
This is how you can use the column property of CSS to layout your website:
The HTML:
<div class="container"> <p> Lorem ipsum dolor sit amet consectetur adipisicing elit. Totam, amet ad reiciendis dolores laborum repellendus quae molestiae magnam incidunt tempora. </p> <p> Lorem ipsum dolor sit amet consectetur adipisicing elit. Totam, amet ad reiciendis dolores laborum repellendus quae molestiae magnam incidunt tempora. </p> <p> Lorem ipsum dolor sit amet consectetur adipisicing elit. Totam, amet ad reiciendis dolores laborum repellendus quae molestiae magnam incidunt tempora. </p> </div>
The CSS:
.container { columns: 3; }
Flexbox
Flexbox was introduced in the CSS release of version 3 to ease the way developers lay out content on a website. It is a unidirectional CSS layout technique with which you can lay out the content of a website in either row or column.
The introduction of flexbox was a game changer because developers had to use floats for the same purpose. With floats, it was really hard to lay out content.
The Steps to Take in Using CSS Flexbox
- put your items in a container div element and bring in flexbox as a value of the display property:
.container { display: flex; }
- specify the direction you want – it should be row or colum:
.container { display: flex; flex-direction: row; }
The default flex-direction is row, so you might not need to use the flex-direction property if you want a direction of row.
To make the items responsive on a mobile phone and other smaller devices, write your media queries and specify a direction of column:
@media screen and (max-width: 700px) { .container { flex-direction: column; } }
This would make everything stack on any device with a maximum screen size of 700px.
CSS Grid
CSS grid is a technology introduced in CSS 3. It lets you lay out your website as grids in rows and columns. So, CSS grid is bidirectional. This means it works in two directions. This is what makes it more advanced than flexbox, which is unidirectional.
CSS grid is a real game changer in laying out the content of a website. It is instrumental in responsive web design.
The Steps to Take in Using CSS Grid
- put your items in a container div element and bring in grid as a value of the display property:
.container { display: grid; }
- the next thing you have to do is to specify the columns and rows you want with two properties – grid-template-columns and grid-template-rows:
.container { display: grid; grid-template-columns: 1fr 1fr 1fr; grid-template-rows: 1fr 1fr 1fr; }
This would give the grid system 3 rows and 3 columns each of 1 fractional unit on a wide screen of desktop and laptop computers.
When you want to make the grid system responsive on mobile phones, you can write the media queries and specify a template column of just 1:
@media screen and (max-width: 700px) { .container { grid-template-columns: 1fr; } }
This would make every row and column stack on top of one another on any device with a maximum width of 700px.
You can represent the template columns and rows as fractional units as I did above, or you can use pixels. For example, 100px.
Flexible Images
Images are a very important aspect of responsive web development. Some images might need to scale down on smaller screens, and some would be okay as they are initially presented.
You can set a reset in your CSS that gives images a maximum width of 100% on any screen size. With this method, you convert the images to block elements and assign a max-width of 100%:
img { display: block; max-width: 100%; }
HTML also has built-in functionality to make images responsive. You get access to it by using the picture tag, so you may not need media queries to make images responsive:
<picture> <source media="(max-width: 1100px)" srcset="ring-tailed-lemur-large.jpg" /> <source media="(max-width: 900px)" srcset="ring-tailed-lemur-medium.jpg" /> <source media="(max-width: 760px)" srcset="ring-tailed-lemur-small.jpg" /> <img src="ring-tailed-lemur-large.jpg" alt="ring-tailed-lemur" style="max-width: 100%" /> </picture>
This results into 3 separate images available to the browser:
- the large image shows on a screen size between 1100px and 900px
- the medium image shows on a screen size between 900px and 760px
- the small image shows on a screen size below 760px
Responsive Text Size
In responsive web development, the size of texts need to scale down on mobile phones. You can achieve this by writing media queries or using relative units like rem (relative ephemeral unit) and em (ephemeral unit) instead of absolute units like px (pixels).
In the code below, the font size would be 3rem on large screens and 1.5rem on any device with a screen less than 700px:
body { font-size: 3rem; } @media screen and (max-width: 700px) { body { font-size: 1.5rem; } }
Showing or Hiding Content
There might be the need to show or hide some content on a website for the purpose of responsive web development.
For instance, if you have two pieces of content that are almost the same but one is larger than the other, you might need to hide the large one on smaller devices like mobile phones.
To get this done, you need to use the display property of CSS. You can set the value to hidden or none. It is recommended to set the value to none instead of hidden. That’s because if you set the value to hidden, the content would still take its place, it just won’t show. But if you set it to none, everything the content, and its space is totally taken off.
In the code below, the large text shows on large devices but is hidden on smaller devices like mobile phones:
.large-text { font-size: 4rem; } .small-text { font-size: 2rem; } .mormal-text { font-size: 1rem; } @media screen and (max-width: 700px) { .large-text { display: none; } }
How to Choose Breakpoints
As already pointed out in this article, media queries is an essential part of responsive web design. Whenever you want to write your media queries, you need to choose the screen breakpoints you want to make the changes on.
This is either a “min-width” or “max-width”. If you are designing in a mobile-first approach, then you need to use min-width to target large screen sizes. But if you are designing with a desktop-first approach, then you should target smaller screen sizes with max-width.
The table below represents different breakpoints you can choose for different screen sizes:
Device | Breakpoint | Examples |
Mobile phones | 320px, 375px, 415px | Small iPhones |
Mobile phones | <700px and >420px | Bigger Android phones |
Tablets | >768px and < 835px | iPad mini |
Tablets | >1024px and <1114px | iPad Pro |
Laptops | >1200px and <1450px | Laptops |
Desktop and TV Screens | >1600px | Desktop computers, large monitors and TVs |
CSS Units and Values for Responsive Design
Choosing the right units is a very important aspect of responsive design. In CSS, there are absolute and relative units. Examples of absolute units are centimeters (cm) and pixels (px) and examples of relative units are rem and em.
Absolute units remain the same on all screen sizes, while relative units would adjust according to the screen size and the user’s preferential settings. This is why it is recommended to use relative units instead of absolute units.
You’d see many developers use pixels (px). It’s not a bad thing to use them in development, but when you are ready to put your website on the internet, you should change them to rem or em.
Instead of using an absolute unit like pixels (px), here are the relative units you can use:
- % — percentage unit. Relative to the parent element. If the parent is 100% and you specify 50% for the child element, the child element takes 50% of the parent element.
- em — ephemeral unit. It is relative to the font-size of the current element. 2em means X2 of the size of the current element.
- rem — relative ephemeral unit. It is relative to the font-size of the root element. 1rem = 16px because 16px is the font-size of the root element unless you reset it.
- vw — relative to 1% of the screen width. 50% vw means 50% of the screen width.
- vh — relative to 1% of the screen height. 50% vh means 50% of the screen height.
Wrapping Up
This article took you through everything you need to know to get yourself familiarized with responsive web development.
The foundation of responsive web design is HTML and CSS. That’s why we at Sololearn created courses to help you learn both HTML and CSS. We also have a course dedicated to responsive web development. In addition, if you want to write your HTML and CSS, you can use our code playground.
The most fundamental thing you need to know is that the content can go side by side on large screens like desktop and laptop computers. But on smaller devices like mobile phones, the content of the web page must be on top of one another on small screens. Flexbox and grid make it possible to achieve that.
Other items on a web page such as texts and images must also conform to responsive web design principles. For texts, you can target different breakpoints with media queries or use relative units such as rem and em. For images, you can convert them to a block display and give them a maximum width of 100%.
Once you know these things about responsive web design, then you are on the pathway to making websites that respond to different screen sizes.