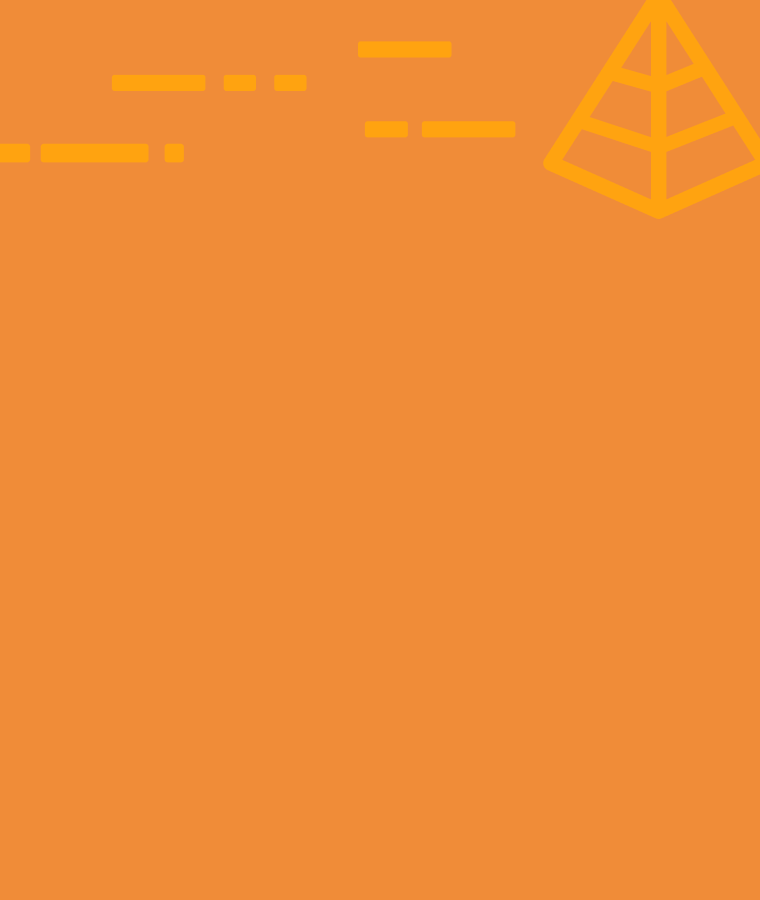
What is Unit Testing? Its Importance and Techniques
Software testing plays a crucial role in the development process. It helps to identify and fix bugs, reduces costs, and enhances the quality and performance of the software. Several testing methods are commonly used during the software development cycle to ensure the quality of the final product. One of the well-known types of functional software testing is unit testing.
Developers use this testing method to find and fix functional errors in the code by dividing it into small logical sections. Unit testing offers benefits like less development cost, proper documentation, and an agile coding process.
In this guide to unit testing, you’ll learn what unit testing is and how it works. You’ll find out the benefits and limitations of unit testing along with its various techniques, tools, and best practices.
What Is Unit Testing?
Unit testing is the software testing method. Software code is split into individual logically isolated units such as function, method, object, or module. Each source code section is then tested to detect and fix issues.
Developers carry out unit testing during the development phase. Unit testing validates that every unit of the code performs as intended and is functional. It helps refine the code too.
Uses of Unit Testing
- Unit testing applies to any software, including web, mobile, desktop applications, and backend services.
- It is a common practice in agile software development methodologies, focusing on frequently delivering small, incremental updates to the codebase.
- You can also find applications of unit testing in extreme programming. In extreme programming, unit tests are created for test-driven development (TDD) purposes.
Importance and Advantages of Unit Testing
Unit testing offers quick insights into the correctness of the code and has the following benefits.
- Unit testing helps identify bugs in the early stages of the development cycle.
- It saves time and money during the later testing phases, such as system and integration testing.
- Making corrections and changes in the code is quick with unit testing.
- It helps in project documentation and code reuse.
- Unit testing offers ease of migrating a software application to a new platform or environment by providing a way to identify and fix potential issues before they become major problems.
- It is easy to onboard new team members in the development as they can also understand the code base with less effort. Moreover, any damage to the code becomes less worrisome as it can be quickly identified.
- Unit testing facilitates changes to the code by providing a way to quickly and easily verify that changes to the code do not break existing functionality. It’s especially important when working on large or complex projects, where changes to one part of the code may have unintended consequences on other parts of the code.
- Unit testing simplifies integration by allowing developers to confidently merge different units or components of the code without worrying about whether the integration will cause issues.
- You can proceed with unit testing without waiting for all the code to be finished. With unit testing, you can test the standalone parts of the project.
Limitations of Unit Testing
Unit testing has the following limitations.
- Writing unit test cases is a time-consuming and costly process.
- Unit testing aims to check the code functionality. Thus, non-functionality parameters are not covered.
- Issues like integration or system-level errors might go unchecked.
- Unit testing is not suited to identifying user interface errors.
- Modifying or introducing new code can lead to navigation difficulties and prolonged time before integration testing begins.
How Does Unit Testing Work?
There are three stages to executing unit testing. You start with planning, creating the unit test, and reviewing it. In the next step, you write the test cases and make scripts. And lastly, you test the code. You must test each case independently to ensure there aren’t any unnecessary dependencies in the code.
The unit testing workflow comprises four stages: create, review, baseline, and execute test cases.
After testing each code unit, developers can further evaluate the larger sections of the code using integration testing. You can perform unit testing in two ways, manual and automated.
1. Manual Unit Testing
Manual testing requires an instruction manual containing a detailed step-by-step process.
The testing begins with identifying the code unit or component that requires testing. It can be a function, method, or class in your application. Next, you write a set of test cases, set up a test environment of test data, and run the test cases to verify the output of the unit. The test cases should include both valid and invalid input and edge cases.
Manual testing allows you to identify problems from the perspective of users. This method enables you to discover errors that may be encountered by individuals unfamiliar with the code and structure, as well as identify any issues related to the program’s usability.
If any test case fails, you debug the unit or component to identify the cause of the failure and fix the issue. You continue to apply these steps to each unit in your application to ensure it is thoroughly tested.
It is important to note that manually performing unit testing can be time-consuming. And it may not be practical for more extensive applications with many code units. As a result, it is often more efficient to use automated testing to help manage and execute the test cases.
2. Automated Unit Testing
To initiate automated testing you write the test code in the software application and remove it once testing is complete. However, a more thorough approach is to isolate a code section to run it through a testing environment using every type of data the code might encounter in real-world use.
This type of unit testing uses a testing framework or tool to develop test cases. You code the criteria into the test to verify the code using the automation framework.
The automation framework records the failed test cases. It also flags the failed test cases with a summary report. It might stop the further execution of the unit testing if a severe failure is encountered. An automated testing tool makes performing unit testing for repetitive test cases easier.
The 4 Most Used Techniques of Unit Testing
Unit testing techniques have four main categories, based on the code aspects they target to test.
- Black box testing covers testing the user interface and input/output parts. It focuses on checking how the software behaves. The testers perform this testing without the knowledge of the code’s internal structure.
- White box testing focuses on testing the internal structure and behavior of a software application. Usually, the developers and engineers who work on code and know its internal structure perform this testing. It is also known as clear, glass, and transparent box testing.
- Gray box testing tests both the presentation layer and the code part of an application. This technique tests the code from the user’s perspective and aims to identify issues that may result from incorrect code or improper structure. Matrix, regression, and pattern testing are types of gray box testing.
- Code coverage technique measures how much of the program source code is executed during testing. It can guide the testing process to ensure that all parts of the code are covered. Some code coverage techniques include statement, condition, finite, and decision coverage techniques.
5 Unit Testing Best Practices
The following best practices can help you run the unit testing process efficiently.
- Test one use case at a time for a clear and focused insight into where the error is coming from.
- Make sure tests are not complex. The more complex a testing code is, the more likely it is to contain bugs. If one of your goals is to keep the app development code simple, the unit testing code should be the same. A good practice is to keep the cyclomatic complexity of the testing code low.
- Tests perform best when code unit outcome does not depend on external influences. Thus, aim to reduce the test dependencies.
- A test whose results change without any code change is unreliable (non-deterministic test). Thus, write deterministic tests that produce the same result no matter the input (without any code change). You can do this by limiting external dependencies and environmental values.
- Create unit tests that are fast, as slow tests are inefficient for frequent use. Although the speed of a test varies depending on what is being tested, generally, tests that take more than an hour and 15 minutes are considered slow.
Top 5 Tools and Frameworks Used for Unit Testing
Below is a list of unit testing software that helps in automated unit testing.
- Jtest is an automated and integrated Java testing tool. It allows you to generate and execute the unit test cases. Jtest offers a wide range of testing capabilities that can be used to test the various aspects of a software application, including its functionality, performance, and security. Large companies like Cisco Systems use this tool. It also offers static analysis.
- JUnit is a free, open-source Java unit testing framework. Its features include annotations, assertions (to identify test methods), and test runners. You can run tests automatically with easily interpretable results. JUnit 5 is the latest version, more flexible and modular than previous versions of JUnit.
- NUnit is an open-source tool for testing .NET languages. It offers manual script writing and data-driven tests that can run in parallel. NUnit version 3 introduces several new features and improvements over previous versions, such as support for .NET Core and .NET Standard, the ability to run tests in parallel, and the ability to create custom test runners.
- PHPUnit is a developer-oriented PHP unit testing tool. It has predefined assertions and has application test helpers to test complex pieces.
- EMMA is another open-source unit testing tool used for Java coding. It has no dependency on external libraries and claims to be fast with small overhead runtime. One of its key features is that it enables efficient development of large-scale enterprise software while allowing individual developers to work quickly and iteratively.
Upskill Your Programming Expertise With Sololearn
Whether you are a beginner wanting to kick-start your career or a professional looking to sharpen your coding skills, Sololearn meets all your needs. With a resource-rich library, you can find beginner, intermediate, and expert-level courses on various programming languages.
Explore the Sololearn courses, and learn on the go with the iOS and Android mobile applications.