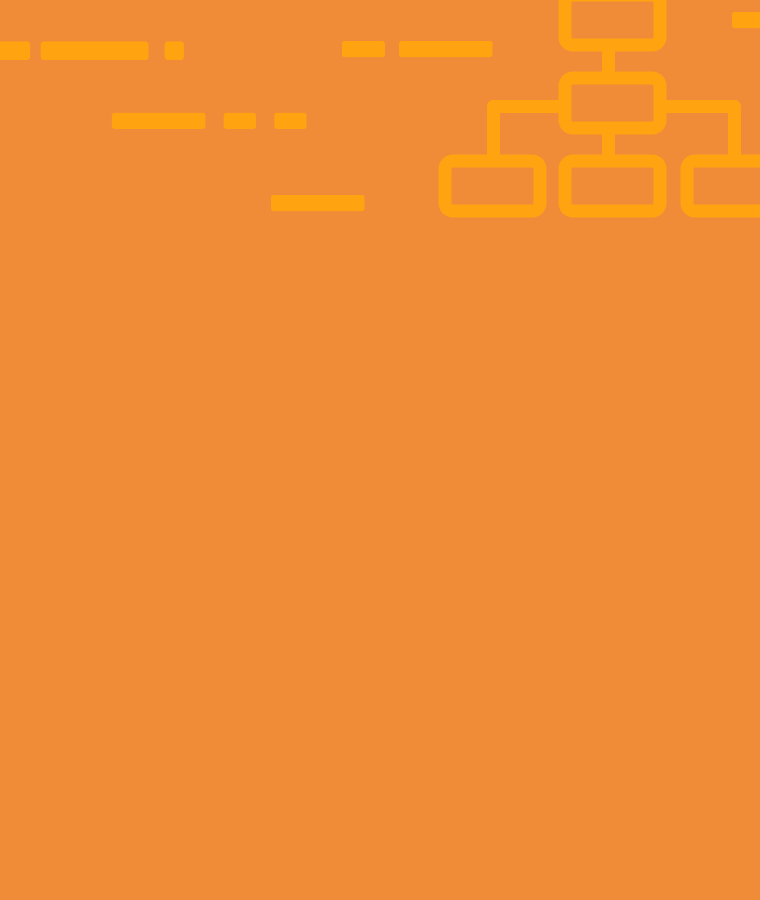
What is DOM?
In the early days of your web development career, you’ll hear many technical terms. You may not understand most of them. For example, you may have heard about DOM. But do you know what DOM is?
It is one of the most important concepts for web developers. Most importantly, it is the key structure that everything is based on. In this article, you can learn everything about DOM. So, without any further ado, let’s dive in.
What is DOM?
DOM abbreviates Document Object Model. DOM is the thing that decides the internal representation of a web page. Also, it decides how elements like headings, paragraphs, etc relate to each other.
Now, let’s understand each word of the abbreviation.
Document
A simple document is a collection of structured information. For example articles, books, or insurance papers are all documents. However, for web developers, a web page is a document. And the DOM is a model that structures the web page.
Do you know? DOM considers all the stuff on a web page as objects.
Object
An object is anything that is displayed on the web page. But developers normally refer to objects as elements or nodes. So, don’t get confused if you hear these terms.
Some of the objects you may use on a web page are:
Content
Videos, images, or even words are part of content objects. And of course, you’ll use at least one of them on your web page.
Structural elements
These objects are not displayed on the page. But they play a vital role in giving shape to your website. Some of the structural elements are divs, containers, and sections.
Attributes
Attributes are part of the DOM. But they’re not treated like content and structural elements, because all elements have attributes like style or size.
Model
A model is a plan for the representation of something like a building or vehicle. It helps us understand how we’ll put it together. You can find models for many things that are under planning. For example, blueprints, floor plans, and IKEA instructions are all models. They have enough details about the object that we’re easily able to recreate them.
The same goes for the model in the DOM, as it acts as a set of instructions for web browsers.
How Does the DOM Treat HTML?
DOM sees any HTML document as a tree of nodes. Where node is an HTML element like a heading or paragraph.
The main HTML file is called the root node which further contains a child node <html>. And within the <html> there are two more child nodes: <head> and <body>.
Keep in mind: The actual work begins in the head and body tag. But we must respect the general document structure to get perfect results.
The head and body tags/elements can contain their own multiple elements. And to access them from the website we can use JavaScript. Let’s find out how you can do that.
Selecting Elements in the DOM
You can access elements from your HTML document by using the following methods:
- getElementById()
- querySelector()
- querySelectorAll()
getElementById()
Ids are unique identifiers for your HTML elements. This means that you cannot give the same id name to two different elements.
For instance, you may have two paragraphs, but you want them to have two different colors. What will you do? You’ll assign them two different id names. It will allow you to style both paragraphs differently.
Let’s understand id with code.
This would be incorrect:
<p id="para">I’m writing a paragraph.</p> <p id="para">I’m writing another paragraph.</p>
Here’s your correct code:
<p id="para1">I’m writing a paragraph.</p> <p id="para2">I’m writing another paragraph.</p>
Now, let’s try to grab ids using JavaScript.
To link JavaScript with an id, use the following code:
document.getElementById("id name goes here")
This code tells the computer to get the <p> element with the id of para1 and print the element to the console.
The following code will get the paragraph with the id “para1” and print it to the console.
Note: A console is a tool in the browser that logs information like network requests and errors. You can find the console by pressing “ctrl+shift+I”.
const p1 = document.getElementById("para1"); console.log(p1);
This code will print the whole element. But if you just wish to see the text then you can use the textContent property in console.log().
const p1 = document.getElementById("para1"); console.log(p1.textContent);
Fun fact: The console is like a playground for developers. They test and solve most of their problems using the console.
querySelector()
In the querySelector() you give the name of an HTML element or CSS property (class or id). According to the given name, it will store the value of the first matching element.
In terms of ids, you’re going to get only one answer, since we don’t use multiple ids. But if you’re using multiple <p> tags or the same class name, you’ll get only the first answer using querySelector().
Let me clear up any confusion with this example:
<h1>Favorite TV shows</h1> <ul class="list"> <li>Big Bang Theory</li> <li>Friends</li> <li>Mind your language</li> <li>The Crown</li> </ul>
If you wish to find h1 from the above code you can just throw the tag name inside the querySelector. And you can also print it using the console.log() method.
const h1Element = document.querySelector("h1"); console.log(h1Element);
Even if you place multiple h1s in the code, you’ll only get the first one.
Remember: You cannot use any HTML element names as variables.
Now, again look at the HTML code. You’ll see that I have declared a class=”list” inside the <ul>. It means you can access it either using the class or the tag. You can practice the tag by yourself, but here we’ll play with the class.
const list = document.querySelector(".list"); console.log(list);
Your confusion is valid if you’re thinking about my variable name. We cannot use HTML element names as variables. But if we’re using something named by us like id or class then there is no issue using the same name for a variable.
This technique will help you a lot when your code grows larger.
querySelectorAll()
querySelectorAll() is similar to querySelector(). The only difference is that querySelectorAll() shows all the matching answers.
For example, let’s try printing list items from the same example.
const myItems = document.querySelectorAll("ul > li"); console.log(myItems);
But there is a small problem here, the above code only displays ul as an object with a number of its elements. It doesn’t display the list names.
So, if you want to see the exact content of the list items then you can use the forEach loop. Here’s how it works:
const myItems = document.querySelectorAll("ul > li"); myItems.forEach((item) => { console.log(item); });
How to Add New Elements in DOM?
So far we were just editing elements using JavaScript. But now we’re going to create some new elements in the DOM using JS.
For creating new elements you can use the document.createElement().
Let’s write some code to further understand the createElement().
<h1>Web development is fun</h1>
This is the only line of my document right now. Now we will write some more HTML lines using JavaScript.
Let’s first create an unordered list and assign it to a variable.
let unorderedList = document.createElement("ul");
The above line will not have any impact on the document. Unless you add the element in the document using the appendChild() method.
appendChild() is a method that will add a child node to a parent element.
As the body is the parent element and unorder list is a child, our code will look like this:
document.body.appendChild(unorderedList);
The next step is to create a list of items, add text to items and add them to our document.
Code for creating <li>:
let listItem1 = document.createElement("li"); let listItem2 = document.createElement("li");
Do you remember the textContent property that we used to get the text of an element? We can also use it to add text to the element. Here’s how you can do that:
listItem1.textContent = "It makes me crazy"; listItem2.textContent = "It’s like an adventure";
Now simply use the appendChild() method to add these list items in your <ul>.
unorderedList.appendChild(listItem1); unorderedList.appendChild(listItem2);
This is what the code looks like all together.
let unorderedList = document.createElement("ul"); document.body.appendChild(unorderedList); let listItem1 = document.createElement("li"); listItem1.textContent = "It makes me crazy"; unorderedList.appendChild(listItem1); let listItem2 = document.createElement("li"); listItem2.textContent = "It’s like an adventure"; unorderedList.appendChild(listItem2);
How to Change the Style Property?
The style property allows us to change the styling of HTML documents.
To demonstrate an example, I’m going to change the color of an h1 using the style property.
Here’s our HTML heading:
<h1>Let’s change my color</h1>
Use the querySelector() to grab the tag in JavaScript.
const heading1 = document.querySelector("h1");
We then use heading1.style.color to change the text from black to green.
heading1.style.color = "blue";
You can use the style property to change more inline styles like background-color, border-style, or font-size.
How to Use addEventListener()?
Editing the styles or elements is fun. But do you know what is more fun? Handling the events. An event can be anything that requires a reaction from your website. Like clicking a submit button.
addEventListener() is a method that detects the event and responds accordingly. Let’s see how it works.
Here I have an HTML button with “btn” as the id.
<button id="btn">Click me</button>
Now we’ll target this id by using the getElementById() method and assign it to a variable.
const button = document.getElementById("btn");
The addEventListener() takes in an event type and a function. In our scenario, we’re taking a click as the event and the function is an alert message.
button.addEventListener("click", () => { alert("Thank you for clicking me"); });
Final words
DOM is an independent interface. It allows us to create, change or delete elements from our HTML document. You can also add events using addEventListener() to make your page more dynamic.
Keep in mind that for selecting elements we use methods like:
- getElementById()
- querySelector()
- querySelectorAll()
And for adding new elements we use the createElement() method.
Also, you can use the style property to edit inline CSS.
Now that you are pretty much aware of how DOM works, and how to control different parts of the DOM, the next step is to practice. Practice as much as you can to become an expert web developer.