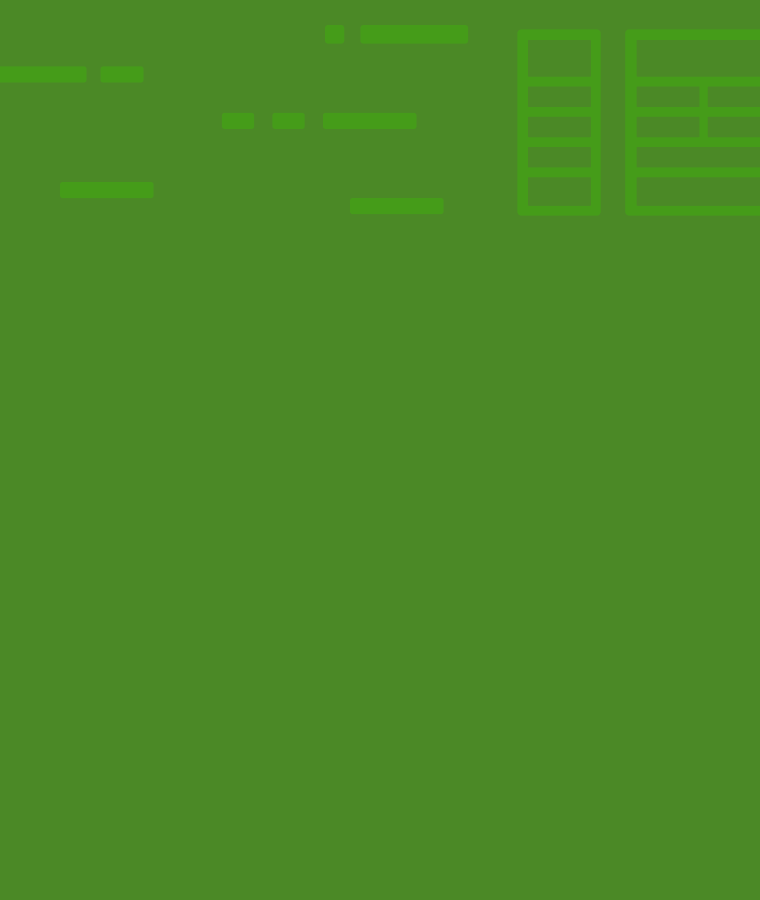
How To Improve User Experience With Responsive Web Pages (Using CSS Media Queries)
We use so many different devices to access the web that static websites are a thing of the past (like the technical version of dinosaurs and mammoths).
These days, webpage content needs to fit on any device screen.
With this CSS technique (media query), you can create responsive web pages which will fit any screen, from mobile to desktop.
With CSS media queries, you can check for the following:
- Width and height of viewport (i.e., the user’s visible area of the web page)
- Width and height of the device
- Device orientation (is the device in landscape or portrait mode)
- Resolution
Media Query Syntax
The media query is declared using the @media rule, which informs the browser that we’ve specified a media query.
The media query consists of a media type and media feature.
Media type allows you to specify the device you want to attach the media feature to. The media feature can be one or more expressions based on what you want to achieve.
The media expression resolves to either true or false depending on the specified media type.
If the specified media type doesn’t match the type of the device the page is viewed on, that means the expression will resolve to false.
If the media type matches the type of the device the page is viewed on:
- The results are true.
- All expressions in the media query are valid, and the corresponding codes are applied when the media query is true.
Media Types
The media types define the class of devices for which the media query applies. It includes;
- All: For all media type devices.
- Print: For printers.
- Screen: This is used to set the screen size of the media query, and the size of the screen can be adjusted using min – width, max – width, and orientation. It is used for computer screens, tablets, androids, i-phones, etc.
- Speech: Screen readers that read pages out loud.
Declaration of Media Query And Media Type
/* when you use @media without specifying all or any media type, it defaults to all. For instance, */ @media and (features) { HTML { /* you will write your style here */ } } /* To specify media type use the code below */ @media media type and (features) { HTML { /* you will write your style here */ } } /*for instance, if the media type is "screen," you will use the code below*/ @media screen and (features) { HTML { /* you will write your code here */ } }
Logical Operators In Media Queries
In media queries, you can use logical operators to bridge two or more conditions.
Examples of some logical operators are:
- And
- Not
- OR
The And Operator
The and-operator is used for joining two or more conditions to the media query declaration. It’s written as shown below:
/* The first example will show how the and operator is used to combine a single feature with media query declaration. */ @media media type and (feature) { HTML { /* Your style here */ } } /* The following example will show you how the "and operator" is used to combine multiple features with media query declaration. The media type and features we'll use in the example below are for the explanation. You'll need to specify your own media type and features to suit your needs.*/ @media screen and (min-width: 480px) and (orientation: landscape) { HTML { /* Your style here */ } }
The Not Operator
The not operator nullifies media queries. The not operator is used for media types that do not support the result.
That is, if the result is false, it returns true.
For instance: if a printer can only show one color (monochromatic) and you want to remove the body’s background color.
Here is the code illustration
@media print and (not (color)) { Body { background-color: none; } }
Note!
The Not operator negates everything after it in the media query. It will only nullify the media query applied if it is used in a comma-separated list.
For instance:
@media not all and (max-width: 700px) { HTML { /* Your style here */ } } /* the query is analyzed as follows */ @media not (all and (max-width: 700px));
/* In a comma-separated list */ @media not screen and (color), print and (color) { HTML{ /* Your style here */ } } /* the query is analyzed as follows */ @media (not (screen and(color))), print and (color)
The Or Operator
The Or operator is known as the comma-separated. You don’t write or when you’re using this operator. Instead, you use a comma in between the features.
If any of the features separated by a comma is true, the style will be applied. That means each condition in the list will be treated separately.
For instance
/* if any of the features match the device, the style will be applied */ @media (min-width: 500px), (max-width: 700px) { HTML { /* your style */ } }
Min and Max Width
When creating media queries for various screen sizes, keep the max-width and min-width properties in mind.
Specifying a max–width value without specifying a min-width value in a media query allows CSS to give the min-width a value of 0px.
All the styles written in the media query are applied to any device with a screen size ranging from 0px to the max–width specified.
The value you’ve given to the min-width is applied to the styles within the media rule until it reaches the maximum width.
For Instance @media screen and (min-width: 576px) { HTML { /* all styles written here will be applied from this minimum width of 567px */ } }
The styles in the media query above would only apply to devices with minimum specified width.
To Define Width Range
Setting the width range gives you some flexibility when creating responsive layouts covering all device widths.
Setting a specific range is the same as using min and max width.
The main difference from what we discussed earlier is that both the min and max values will be specified. For instance,
@media screen and (min-width: 300px) and (max-width: 700px) { HTML { /* all styles written here will be applied from this width range of 300-700px */ } }
The media query above will only work for the device with the specified screen size.
It uses the min-width: 300px provided as the start value and the max-width: 700px as the end value.
The Media Breakpoints
A breakpoint is a limit defined by developers for determining when to change the layout and apply the new rules within the media queries. For instance
@media screen and (min-width: 300px) { HTML { /* styles here */ } } @media screen and (max-width: 700px) { HTML { /* styles here */ } }
In the above examples, the breakpoints are the points specified in the code. Which are, min-width: 300px and max-width: 700px
Setting media breakpoints prevents web content from falling out or moving out of position on different screen sizes.
The responsive design media breakpoints can be specified based on
- Device
- Content
- Min – width and Max – width
Is There a Common Breakpoint?
One of the most frequently asked questions is, “Can I use a specific breakpoint for all devices?”
We cannot use fixed breakpoints for all devices because the screen sizes of all devices are different.
You can also use the breakpoints of a CSS framework (such as Bootstrap, Bulma, or others).
Viewport VS Media Query: The Differences
You might think that the viewport and media query are interchangeable. NO! They are to be used together because they perform different functions.
Here are some differences between the media query and the viewport, which also show why they must be used together.
- The media query allows you to specify the media type and create layouts for different device screen sizes. At the same time, the viewport tells the browser that the user’s visible area should be equal to the device width you are using.
For example, suppose your device’s screen size is 400px. With media query, you can change the background color or even the navbar position at that size.
The viewport allows text to be readable according to your device’s screen width.
- Viewport syntax is written as follows in HTML and CSS.
/* HTML */ <meta name = ''viewport'' content = "width = device-width, initial-scale =1"> /* CSS */ @-ms-viewport { width: device-width; }
Screen Vs. Only Screen: When To Use The Only Keyword
The screen is a media type used to set the screen size of a media query.
@media only screen and (min-width: 360px) and (max-width: 768px) { HTML { /* Your style here */ } }
In the example written above, you will notice that the screen media type is written with the only keyword. This keyword prevents older browsers that don’t support media queries from applying the style.
Media Query Resolution
Resolution refers to the number of pixels that make up a screen. The resolution media query can test the output device’s pixel density.
You can also set minimum and maximum values using the min – resolution and max – resolution keywords.
Check out the illustrations below.
/* minimum resolution */ @media (min-resolution: 10dpi) { P { color: orange; } } /* maximum resolution */ @media (max-resolution: 300dpi) { Body { background-color: green; } }
From the illustration above style:
If the min-resolution of the output device is 10dpi, the output paragraph is orange. Also, if the max-resolution is 300doi, the background color will be green.
Getting Started With Responsive Web Design
- This lesson isn’t for beginners but for people with previous knowledge of CSS.
- If you are new to CSS, you can visit our website to take a very detailed lesson on the fundamentals of CSS.
- You can try your hands on different exercises, which you can find in the code playground on Sololearn’s website.
- You can also join a community of developers to interact with professionals and get answers to your questions.
Conclusion
Learning CSS and responsive web design on your own can be tricky. But with well-planned courses on Sololearn, you can learn at your pace from the comfort of your bed.
Do you want to learn more about responsive web design? Get access to Sololearn’s responsive web design course.
References
- (2022, May 20). How to use CSS Breakpoints and Media Breakpoints. What are CSS Breakpoints and Media Query Breakpoints. Retrieved August 1. 2022, from
- Webplatform. Height. height · WebPlatform Docs. Retrieved August 1, 2022, from https://webplatform.github.io/docs/css/media_queries/height/