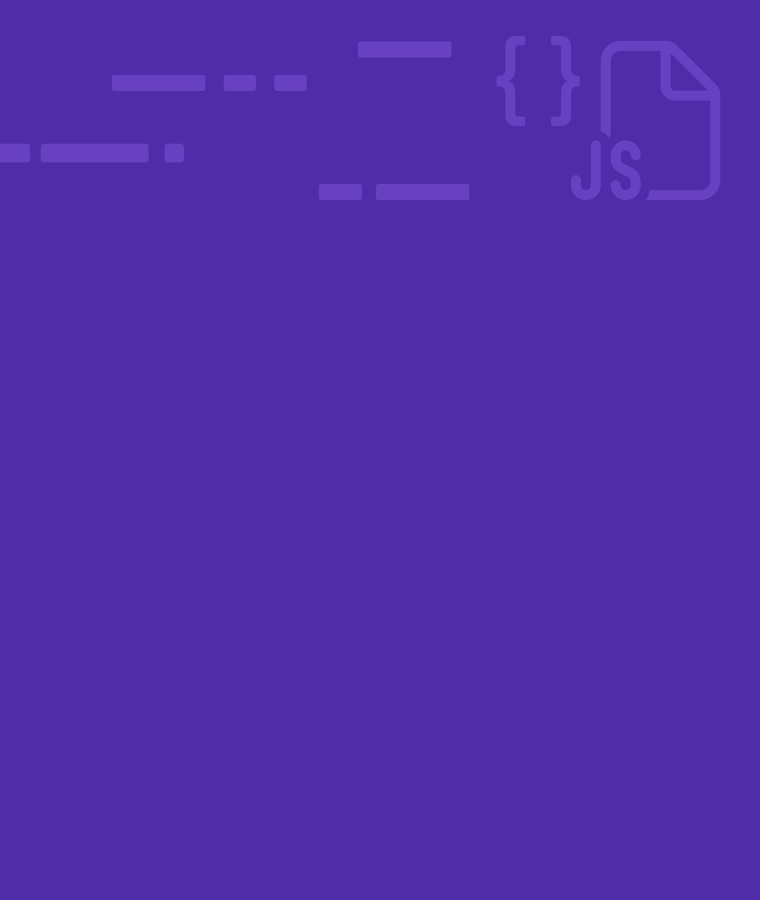
What is a Function in JavaScript?
Learn what a function is, why you need it, and how to create and use a function in JavaScript.
A function is a part of your code that does exactly one thing. It’s a logical unit of code that can carry out its task efficiently every time you call it. So you can call a function and it will execute what it was set to do.
In a nutshell, programming means giving instructions to computers or digital devices. In programming, these instructions are called statements. A function contains one or more programming statements. As such, when you call a function, the computer executes the statements inside this function, one after another.
A function takes an input(s) that it will use to carry out its task. An argument is an input you give a function when you want to call it. So functions can take arguments and use them to perform their specific jobs.
A function has a point of reference (or a name). A function’s name describes what it does. In most cases, the function’s name starts with a verb. For example, if you have a function that displays the sum of two numbers, its name will be addTwoNumbers.
To call a function, write its name followed by its arguments inside parentheses ( ) . Something like the following:
addTwoNumbers(1, 2) // 3
In the above call to the addTwoNumbers function, “addTwoNumbers” is the name of the function while 1 and 2 are its arguments (inside parentheses).
Note: Arguments are optional. Some functions may not need an argument(s). In that case, you don’t put anything inside the parentheses when you call such functions. E.g. To display pi, you can have a displayPi function that doesn’t need any arguments:
displayPi() // 3.14159...
Why do you need a Function?
- Functions make code shorter and snappier
DRY is a programming technique that involves making your code shorter where possible. The smaller the code, the better it is — given that it still does the same thing. DRY is an acronym for Don’t Repeat Yourself.
When coding, some statements repeat themselves frequently. So instead of continuously repeating those statements, you can group them into a single unit — a function. In other words, functions help to make your code DRY.
- Functions arrange the code
Programming is used to solve problems. As a programmer, you are a problem solver. When you want to solve a problem, you first think out the various steps involved in the solution. Then you use programming languages like JavaScript to solve these problems.
Given a well-defined solution, you can use functions to break down this solution into specific tasks. Hence, functions help arrange the code.
Let’s say you are building a personal assistant website. When the website loads, you want it to fetch all schedules, sort them, and then display them on a calendar. You can use various functions for each of these tasks. You could have the following JavaScript code for this website:
fetchAllSchedules() sortSchedules() displayOnCalendar()
How to Create a Function in JavaScript
To declare a function means to create it. When creating a function, you specify its name. If that function will be called with any arguments, you indicate the arguments when creating the function.
A parameter is a variable that a function uses to perform its task. When creating a function, parameters are the arguments of that function. So when declaring a function, you provide parameters — variables that the function will use. But when calling functions, you provide arguments — values of the parameters.
When you declare a function you provide the function’s body. This body contains all the statements that the computer will run line by line when you use that function.
When declaring a function, surround the function body with left and right curly braces { } . These braces (and hence the function body) come after the parameter list (the parentheses ( ) ).
There are two ways to declare a function in JavaScript. They are as follows:
- Using the function keyword.
- Using the arrow syntax.
- Declare JS Function with function keyword
To declare a function using the function keyword, carry out the following steps:
- Type the function keyword followed by the desired function’s name.
- Type open and close parentheses ( ) . Add the function’s parameters inside these parentheses (i.e. if the function needs parameters).
- Type left curly brace { .
- Type the statements of the function (the function’s body).
- Type right curly brace } .
For example, using the function keyword method, you will declare the addTwoNumbers function in the following way:
function addTwoNumbers(a, b) { const sum = a + b console.log(sum) } addTwoNumbers(1, 2) // 3
Where the parameters of the addTwoNumbers function are a and b.
- Declare JS Function with arrow syntax
To declare a function with the arrow syntax, carry out the following steps:
- Type the const keyword, then the desired function’s name and an equals sign = .
- Type open and close parentheses ( ) . Add the function’s parameters inside these parentheses (i.e. if the function needs parameters).
- Type an equals sign joined with a greater sign together => .This is the arrow syntax.
- Type left curly brace { .
- Type the statements of the function (the function’s body).
- Type right curly brace } .
For example, using the arrow syntax method, you will declare the addTwoNumbers function in the following way:
const addTwoNumbers = (a, b) => { const sum = a + b console.log(sum) } addTwoNumbers(1, 2) // 3
Should you use function keywords and arrow syntax to declare functions?
What is similar?
Both ways set the function name, its parameters, and its body.
What is different?
- One way uses the function keyword while the other uses the arrow (equals and greater than signs) => .
- Also, in the first way, the function keyword comes first while in the other, the arrow => comes in between the parentheses and curly braces.
- Another difference is that you must assign the function to a variable with the arrow syntax way. In other words, const with the function name, followed by the equals sign, has to precede the parentheses.
Which should you use?
The arrow syntax method is the recommended way to declare functions. It is more modern than its function keyword counterpart. It is recommended because you can use it as a one-liner (coming later).
What are Void and Value Functions?
The above example addTwoNumbers function is a void function. A void function is a function that does not return a value. Returning a value means that there will be a return keyword with the result of some computation.
A void function simply does some computation and ends there. It carries out one or more statements. On the other hand, a value function does computations and returns a value. So, at the end of a value function, you will see the return keyword with some value or variable.
Because value functions can return a value, you can call them anywhere you will insert a variable. Let’s use the above addTwoNumbers function. To convert it from a void to a value function, return the computed sum. Then you can use the return value as you wish. Something like the following:
const addTwoNumbers = (a, b) => { const sum = a + b return sum } console.log(addTwoNumbers(1, 2)) // 3
Value functions are useful when you need to use the return value. You could also use them when you want to perform another computation with that return value.
Let’s say you want to display the double of the returned sum. You will create another displayDouble that doubles its argument and displays the double. Something like the following:
const displayDouble = (n) => { console.log(n * 2) } displayDouble(addTwoNumbers(1, 2)) // 3
One-liner JavaScript Functions
The above displayDouble function has only one statement. Anytime a function has only one statement, you can write its body (the single statement), without the enclosing braces. These kinds of functions are called one-liners.
Rewriting the above displayDouble function to be a one-liner, we have the following:
const displayDouble = (n) => console.log(n * 2) displayDouble(2) // 4
One-liners are elegant. They are short and easy to read. You can only use one-liners, once there is only one statement in a given function. If the function has more than one statement, remember to put its body inside curly braces.
Congrats! You’ve now know what JS functions are
A function is a group of codes that do the same thing when you call it. It has a name, parameters, and body. You can supply arguments (if need be) when you call a given function. A function can return a value. This return value can be used for other computations or assigned to a variable.
Generally, use functions when you are in either of the following scenarios:
- When you need to repetitively carry out a given task.
- When you need to break down a huge task into smaller specific tasks.
Functions are a great part of JavaScript, but the language also has other important parts like conditionals, loops, operators, etc. But learning them doesn’t have to be hard. Just check out our bite-sized lessons and try writing some JavaScript code of your own on Sololearn. Learn JavaScript for FREE on Sololearn here.