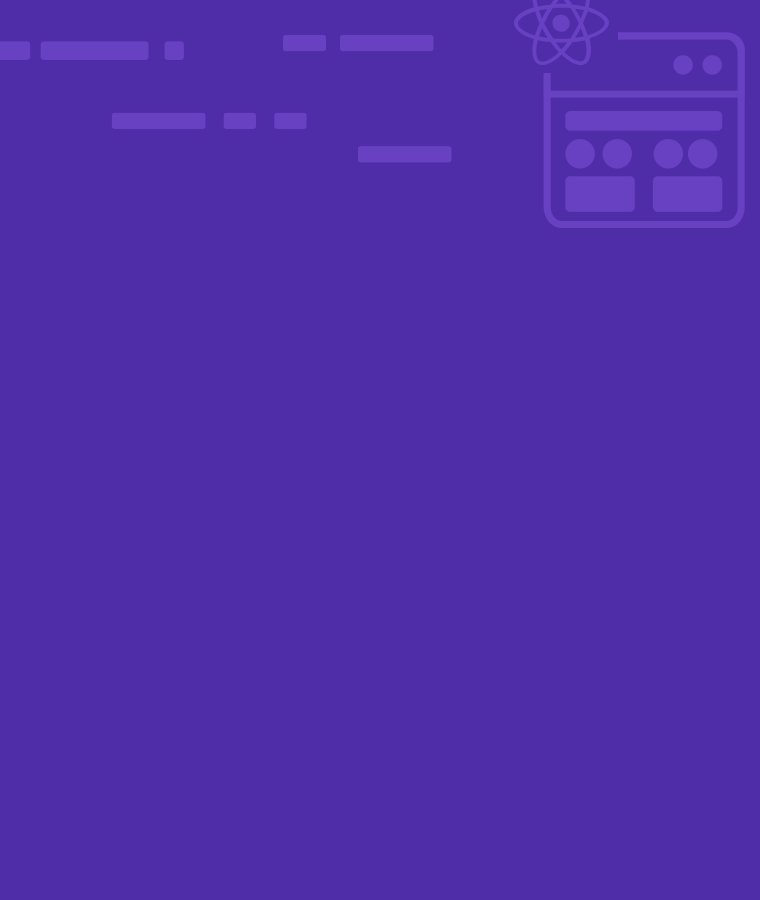
React components: what are they, what are they for, and how to use them?
A component is to Reactjs what battery is to a laptop. React is all about components. So, if you want to learn React in the nearest future, be aware that you’ll be writing a lot of components. And if you are already making web applications with React, then you are writing a lot of components.
In this article, you’ll learn what React components are in a way you can understand. You will learn:
- what components are in relation to states
- functional and class-based components in ReactJS
- how to render a component in ReactJS
- and how to pass arguments into components in ReactJS in form of props (properties).
What is a Component in React?
Components are the basic building block of a React application. In JavaScript, you write functions you can reuse over and over again and import into another code. That’s what components do in React. Components in Reactjs is an independent and reusable pieces of code. So, components can exist alone and can be brought in into another component.
In React, you can do the same thing in a lot of ways. So, to write components, you can do it in at least two ways – you can write it in form of a JavaScript function or class. In the next two sections of this article, we’ll look at functional and class-based components.
A React component in can be stateless or statefull. A stateless component is a component that has no state and a statefull component is a component that has one or more states. In the next section, I will show you what stateless and statefull components are.
If you are wondering what state is in React, take a look at it as React’s way of writing variables. By definition, it is an object that stores data or information in a React component.
Stateless vs statefull components
The main difference between stateless and statefull components is that one has a state and the other doesn’t.
A stateless component in Reactjs is a component that has no state. A stateless component is also called a dumb component. This means it’s not keeping track of data or information.
The code below represents a stateless component:
export default function App() { return ( < div className = "App" > < h1 > Hello Sololearners < /h1> < h2 > Welcome to < a href = "sololearn.com" > Sololearn < /a></h2 > < /div> ); }
On the other hand, a statefull component is a component that has one or more states. This means it’s keeping track of data or information. It is also called a smart component.
To show you how statefull component looks in code, I have prepared the code below:
import { useState } from "react"; export default function App() { const [name, setName] = useState(""); return ( < div className = "App" > < h1 > Hello Sololearners < /h1> < h2 > Welcome to < a href = "sololearn.com" > Sololearn < /a> < /h2> < p > Enter your name to start coding: < /p> < input type = "text" value = { name } onChange = { (e) => setName(e.target.value) } /> < p > Welcome, { name }. < /p> < /div> ); }
Functional components
A React functional component is a pure JavaScript function that returns one or more JSX elements. JSX is the way you write HTML in React, so JSX elements are also HTML elements.
A functional component is the new way of writing components in React. You can write a functional component as a JavaScript arrow function or pure function.
The example below represents a basic functional component:
const FunctionalComponent = () => { return ( < div > < h1 > Sololearn < /h1> < p > You can write codes in any programming language on Sololearn < /p> < /div> ); }; export default FunctionalComponent;
Class components
As the name says, a React class component is a JavaScript class. To make sure that the class inherits everything from React, it has to extend React. To return JSX in a functional component, you use the render method.
To understand class-based components better, you should checkout this code:
import React from "react"; class ClassComponent extends React.Component { render() { return ( < div > < h1 > Sololearn < /h1> < p > This is a class component < /p> < p > You can write codes in any programming language on Sololearn < /p> < /div> ); } } export default ClassComponent;
Rendering components
To make sure the components you have written show in the UI, you have to render them. And to render a component, you pass them into another component or the app component as a self-closing tag. That is, a tag that has no closing tag.
For example, I named the functional component as FunctionalComponent.jsx and the class-based component as ClassComponent.jsx. To render them in the app component I need to import them and pass them in as self-closing tags this way:
<FunctionalComponent /> <ClassComponent />
The full code looks like this:
import { useState } from "react"; import ClassComponent from "../ClassComponent"; import FunctionalComponent from "../FuncionalComponent"; export default function App() { const [name, setName] = useState(""); return ( < div className = "App" > < h1 > Hello Sololearners < /h1> < h2 > Welcome to < a href = "sololearn.com" > Sololearn < /a> < /h2> < p > Enter your name to start coding: < /p> < input type = "text" value = { name } onChange = { (e) => setName(e.target.value) } /> < p > Welcome, { name }. < /p> < FunctionalComponent / > < ClassComponent / > < /div> ); }
And this is what you get in the browser if you run the code:

Components in components
It is possible to have another component right inside another component in React. That’s what React developers call component in component.
Look in the code below to see an example of a component in component:
const FunctionalComponent = () => { // Greet component inside another component function Greet() { return < p > Hi there, how are you today ? < /p>; } return ( < div > // rendering the greet component < Greet / > < h1 > Sololearn < /h1> < p > This is a functional component < /p> < p > You can write codes in any programming language on Sololearn < /p> < /div> ); }; export default FunctionalComponent;
In the code above:
- the FunctionalComponent component is the main component
- the Greet component is the “component in component”.
Components in File
Components in file is the standard and the accepted way of creating components in ReactJS. This means you create a JavaScript or JSX file and you write your component in it.
In React, you should have one component per file. So, it is not a good practice to have a component in component. What you should do instead is to put your components in their respective files.
Conclusion
This article showed you everything you need to get started with components in Reactjs.
We looked at stateless and statefull components. Next, we took another look at the two ways you can write a component with functional and class-based components in ReactJS.
If you want to learn more about components in ReactJS and React in general, we have a beginner-centered React course on our learning platform.
Thank you for reading.