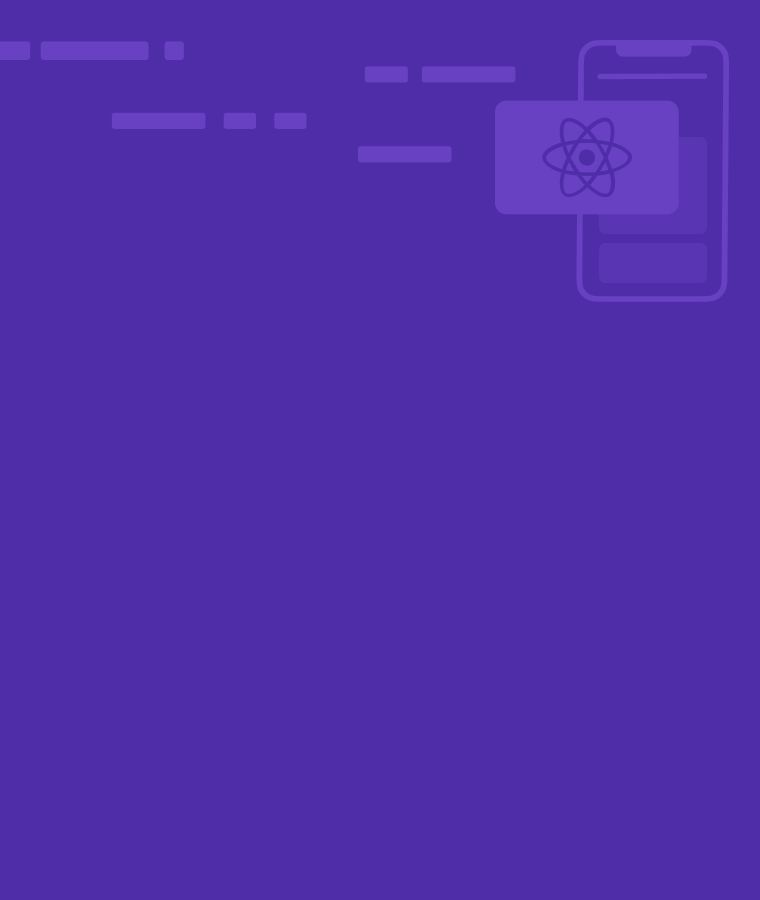
How to style your React app?
If you want to add style to React components, your creativity is your limit. Styling in React is like styling an HTML file, but you have the chance to get a lot more dynamic.
Apart from the conventional ways of styling such as adding classes and ids, direct styling in a CSS file, and others, you can add styles with the native JavaScript objects. You can also use CSS modules without any complex setup.
In this article, you’ll learn about four different ways you can style in React. By the end of the article, you’ll be confident in styling React components and get to know how React styles work.
This is what we’ll be styling in this article:
import Logo from "./sololearn-logo.png"; function App() { return ( <div> <img src={Logo} alt='Sololearn logo' /> <h1>Welcome to Sololearn</h1> <h2>The best platform for coding beginners</h2> <p> At Sololearn, you get to learn programming languages like JavaScript, Python, C++, Java, and many more. </p> <p> We also have learning resources for frameworks like Angular, Django, React, and many more. </p> <a href='sololearn.com'>Start Learning</a> </div> ); } export default App;
And this’s how it looks in the browser:

Add CSS Classes to Components
Just like the way you can add classes to HTML elements, you can also add CSS classes you’ve written to your JSX elements. The only difference is that “class” is not called “class” in React, it is called “className”. That’s because “class” is a reserved keyword in JavaScript. And in React, you’re always writing JavaScript.
I want to center every content of our JSX. So, I’m going to add a “className” I call “layout” to the top-level `div`. I’ll then write out the CSS for that `className` in the `index.css`.
The index.html file:
* { margin: 0; padding: 0; box-sizing: border-box; background-color: #f1f1f1; } .layout { display: flex; align-items: center; justify-content: center; flex-direction: column; height: 100vh; }
The JSX:
<div className='layout'> ... </div>
Now, everything is centered:

**N.B**: I didn’t have to import the CSS file because any style you put in the index.css file in React is available to your components. You must import the style file if you have a particular style file for a component. Otherwise, it won’t work.
Add Inline CSS to Components
Remember you can always use inline styles in your HTML. The same thing applies to JSX, but the process differs. It follows the JavaScript object’s syntax.
To pass inline styles to your JSX, here are the processes you need to follow:
- you need to bring in the `style` attribute first
- after that, you have to put the properties and values in an object right inside the style attribute. This means you have to use double curly braces.
- the values you pass to the properties must be string values even if they are numbers
- if you have many properties you want to apply, you have to separate them with commas
- if you’re using properties that have more than one word, you have to write them in camel case. So, “background-color” becomes “backgroundColor”.
In the simple web page, we are styling, I’m going to reduce the width and height of the Sololearn logo with inline styles:
<img src={Logo} alt='Sololearn logo' style={{ width: "180px", height: "180px" }} />
The Sololearn logo is now smaller:

Use JS Objects to Style
You can use the native JavaScript object for adding styles to your React components. To do this, you need to write the object outside of your JSX and pass it in as an inline style in a style attribute. With this approach, you don’t need to use double curly braces. That’s because the curly braces of the object is already one.
To show you how to use objects to style your React components, I’m going to style both the h1 and h2 texts of our simple web page with objects.
These are the objects I wrote:
const headingOneStyles = { margin: "10px", backgroundColor: "#EA589F", borderRadius: "4px", padding: "4px", }; const headingTwoStyles = { margin: "7px", backgroundColor: "#34C1F6", borderRadius: "4px", padding: "4px", };
This is how I passed the objects into the h1 and h2 texts:
<h1 style={headingOneStyles}>Welcome to Sololearn</h1> <h2 style={headingTwoStyles}>The best platform for coding beginners</h2>
And this is how they look in the browser:

CSS Modules
CSS modules is one of the coolest ways to style a React application. With CSS modules, you get to write your styles as CSS and consume them as JavaScript objects in your JSX.
To use CSS modules, here are some rules you need to follow:
- you need to create a CSS file with the extension `.module.css`. So, the name follows the syntax `cssfilename.module.css`
- write your CSS properties and values as classes
- import the CSS module file
- consume the styles inside the styles as an object inside a “className”
To show you how CSS modules work in practice, I’m going to style the paragraph and the button on the web page we’ve been using as an example. As you can see below, the paragraphs and button don’t look good enough:
So, I’ll create a file named styles.module.css and put the styles in it:
/*styles.modules.css file*/ .text { padding: 5px; } .button { text-decoration: none; margin-top: 10px; color: #fff; background-color: #ffa310; padding: 10px; border-radius: 4px; } .button:hover { background-color: #c07908; }
I’ll import the CSS module this way:
import styles from "./styles.module.css";
To consume the CSS modules, I’ll pass it in as an object inside a `className` attribute:
<p className={styles.text}> At Sololearn, you get to learn programming languages like JavaScript, Python, C++, Java, and many more. </p> <p className={styles.text}> We also have learning resources for frameworks like Angular, Django, React, and many more. </p> <a href='sololearn.com' className={styles.button}> Start Learning </a>
Notice that I use the exact classes I used inside the CSS module (.text and .button)
Now the paragraphs and button look better:

Conclusion
This article showed you 4 different ways you can style your React applications.
We looked at:
- how you can add classes to your components by using classes (className in React)
- how you can add inline CSS in the syntax of a JavaScript object
- how to pass in native JavaScript objects as styles
- and how you can use CSS modules.
If you want to learn more about React and how to style React components, you should check out our React course for beginners.
Thank you for reading.