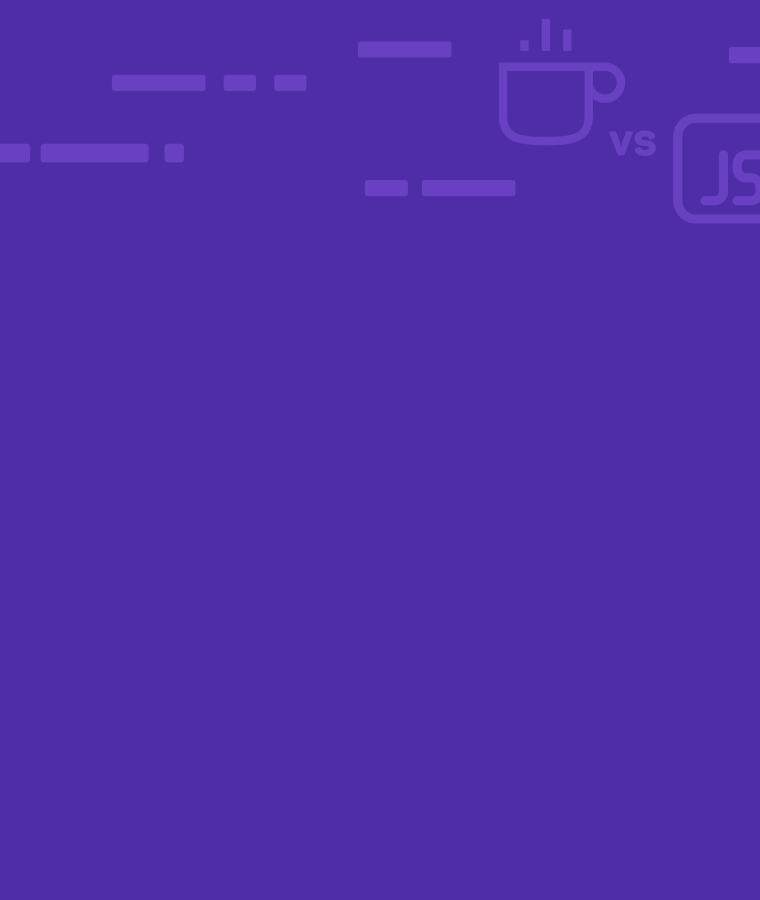
Java vs JavaScript for newbies
Java and JavaScript resemble in their names. Yet, they have plenty of striking differences between them. They are both modern programming languages that you can use to build great tools and land good-paying jobs.
In this article, you will know what Java is and what JavaScript is. You will see how they resemble and why their names are similar. You will also learn the many differences between both programming languages.
What is Java?
Java is a programming language for:
- Building desktop applications.
- Building mobile applications.
- Building servers.
For desktop applications, you can use Java to build software that a user can install on either Windows, Linux, or Mac Operating Systems. For mobile applications, you use Java to make Android apps. For servers, you can use Java to build the backend of a website.
What is JavaScript?
JavaScript is a programming language for building websites. JavaScript makes websites dynamic.
For example:
- When the user clicks a button, you can set JavaScript to react to the click appropriately.
- When the user searches a word, you can set JavaScript to look for matching phrases and display them.
- When the user signs out of their account, you can write JavaScript code to show the login page.
You can also use JavaScript to build the backend of a website.
Are Java and JavaScript the same language?
No. Java and JavaScript are not the same programming languages.
You can use Java to build desktop applications whereas you use JavaScript for building websites.
Why do Java and JavaScript have similar names?
To understand why they resemble, let’s go back into their history. Java and JavaScript were both released in 1995. However, Java came first.
As of then, browsers lacked standards. Java had a tool to use to add interactivity to websites. This tool is no longer in use and its name is an applet. As of that time, web developers sent Java applets to the frontend to add interactivity to the web.
Java was becoming popular about the time JavaScript was created. As a result, JavaScript’s Author made JavaScript’s syntax to resemble Java’s own. Here, syntax means “how you write” a programming language.
Programming languages aimed at manipulating existing systems are called scripting languages. JavaScript was created to manipulate different parts of a website (the HTML and CSS). So, it’s a scripting language.
The above Java factors and being a scripting language made JavaScript have its name.
What do Java and JavaScript have in common?
They both resemble in terms of their syntax (how you write them). The syntax of a programming language is like its grammatical rules. See it as the dos and don’ts. The syntax specifies where and where not to put peculiar symbols and names.
To illustrate this syntax similarity, let’s try building a printSum function. This function will print the sum of two numbers. In Java, the function will look like the following:
void printSum(int a, int b) { System.out.println(a + b); }
In JavaScript, the same function will look like the following:
function printSum(a, b) { console.log(a + b); }
Both code snippets use comma, parentheses, curly braces, dot, plus sign, and semicolon in the same place. This indicates the syntax similarity between Java and JavaScript. If you want to create another function, you will still use these symbols in the same place.
Of course, there are some differences in keywords. The Java snippet has void and int. The JavaScript snippet has function. These keywords are part of the syntax of each language. The System.out.println line in Java and the console.log line in JavaScript each print out the sum result to the console.
What are the differences between Java and JavaScript?
Aside from the above differences in keywords and syntax, these languages have more significant differences.
1. Compiled vs Interpreted
There are two ways to consume video content: movies or live streaming. With movies, the film makers record the video, process it, then make the movie available. Movie watchers can then see those movies after they have been bundled into one.
With live streaming, we consume the videos in real time. It could be sports or a social media live stream. Either ways, as the recorder is producing the video, we get to see it. The recorder doesn’t get to pre-process or bundle the video before we watch it.
Across the above two ways, there is video production and consumption. But with movies, the consumption is after a bundling or editing step. This editing step doesn’t exist with live stream. You can liken these two ways to the process in which computers understand code.
As a programmer, you write code for the computer to understand. If you write with a compiled programming language, the computer will first convert your code before it will execute it. If you write code with an interpreted programming language, the computer will execute your code in real-time as it goes through the code. It doesn’t need to first compile it.
Java is a compiled programming language.
This means that it comes with a compiler. A compiler is a tool that you install to run Java program files. The compiler reads through the entire Java code and converts it into a software. The computer will then run this compiled software.
Java files usually have a main method (i.e. the starting point of the compiled software). Let’s create a Hello World program in Java. This program outputs “Hello World” to the console.
class Hello { public static void main(String[] args) { System.out.println('Hello World'); } }
The above program starts with the class keyword (we’ll explain it later).
Let’s focus on the public static void main(String[] args) line. If the Java compiler doesn’t see this line, it won’t run. The way you see it is how Java asks us to always specify the main method.
Because of compilation, the computer cannot run the compiled code line by line. This is because the compilation step converts the code entirely. This is why the main method is important. The main method is where the computer starts running compile code from. It is necessary in compiled programming languages.
JavaScript is an interpreted language.
It comes with an interpreter (a tool that runs code line by line). It doesn’t first bundle the code into software as is with the compiler. This is why JavaScript is a scripting language. It manipulates the website in real-time.
You don’t need a compiler for JavaScript. JavaScript doesn’t have two steps to run code (compile then run) like is with Java. Let’s create a Hello World program in JavaScript. It also outputs “Hello World” to the console.
console.log('Hello World');
It takes just that line. The interpreter will read and execute that line immediately. It will print “Hello World” to the console. As you can see, here you don’t need a main method.
2. JDK vs Browsers and NodeJS
JDK stand for Java Development Kit. It’s software that contains the tools to compile and run Java code. Before you can run Java, you need to download and install the JDK. The JDK is available for all operating systems so Java code can work on pretty much every platform.
Major browsers contain a JavaScript interpreter so they can run JavaScript code. NodeJS is a JavaScript engine outside the browser. You download and install it just as you will download the JDK. You can then use it to run JavaScript code on your computer without needing a browser. Then, you can use JavaScript to build servers for your website.
3. Statically Typed vs Dynamically Typed
Typing in programming is telling what kind of value is in a variable. A type can be
- A boolean type (value for either true or false)
- A number e.g. 1, 2, 3, …
- A string type (just text that remains as it is). Always in quotes e.g. ‘Sololearn’
- Any custom type that you or other programmers create
In a statically typed programming language:
- A variable’s type tells the value it holds.
- If a variable is a boolean type, it can either hold true or false.
- If a variable’s type is an int, it can hold any integer (positive or negative whole numbers).
In such languages, you must specify the type of every variable.
Java is a strongly typed language. If you try to assign an integer value to a boolean variable, the compiler will return an error. Look at the printSum function in Java once again.
void printSum(int a, int b) { System.out.println(a + b); }
The function parameters a and b each specify their type int. So if we try to use a non-integer value when calling this function, the compiler will return an error, and the program won’t execute.
In a dynamically typed programming language, these rules don’t exist. You are free to switch the value of a given variable. You don’t specify the type of a variable when creating it.
JavaScript is a dynamically typed programming language and it’s flexible. That’s why in JavaScript’s printSum function:
function printSum(a, b) { console.log(a + b); }
We do not need to specify the variable types of the function parameters a and b.
4. Object-Oriented Programming vs Functional Programming
Object-Oriented Programming (OOP), as its name suggests, is a programming concept that focuses on objects. Here, an object means any data that the code will work with. OOP is a coding method where you think more of the data the code will change rather than the code itself.
In OOP, each object has its own type. To create an object’s type, use the class keyword. Both Java and JavaScript are Object Oriented Programming languages. The difference is in the strictness. OOP is compulsory in Java. In Java, you must do everything within a class. The simple Hello World program must be inside a class in Java.
OOP exists in JavaScript but it is not very tight as is with Java. You can use classes to create objects in JavaScript. But you don’t need a class for everything in JavaScript. This is what makes the difference. In fact, JavaScript is a functional programming language.
A functional programming language deals more with executing functions. It focuses on the logic it runs. JavaScript is more of a functional programming language. You can write functions directly to manipulate the HTML and CSS contents of a website. However, in Java, you would have to do this inside classes, which might not be the best fit.
5. Other Differences
There are more differences between Java and JavaScript. With regards to what it takes to learn either language, Java is quite demanding. Java has so many features and it’s relatively more verbose compared to JavaScript. Verbose here means it has a lot of code for the same functionality. You’ll notice this with the simple Hello World program in both languages.
What else? Well:
JavaScript is easier to learn and understand. It’s the most used programming language and it powers all websites today. JavaScript also used less computation memory compared to Java. A Java file ends in .java extension while a JavaScript file ends in .js extension.
Which should you learn?
It depends on what you want to achieve. If you want to build desktop applications or you want to work in companies that use Java, learn Java. If you want to build websites, either the frontend or the backend, learn JavaScript.
Despite their names, Java and JavaScript serve different purposes and are different from a programmer’s point of view. The following is a summary in tabular form.
aspect | Java | JavaScript | |
1. | Usage Mode | Compiler | Interpreter |
2. | Tool | JDK | Browsers and NodeJS |
3. | Variable Types | Static Typing | Dynamic Typing |
4. | Programming | Object Oriented | Functional |
5. | Learning | More complex to learn | Easier to learn |
6. | Memory | Uses more memory | Uses less memory |
7. | File extension | .java | .js |
What Next?
It is time to learn. And that’s what we do at Sololearn.
Now you’ve known the differences between Java and JavaScript, which do you prefer? Do you want to learn Java? Then you can start your first Java lesson here. Or are you more curious about JavaScript? Start your first JavaScript lesson here.
Do you want to learn both? Start Java here and start JavaScript here.