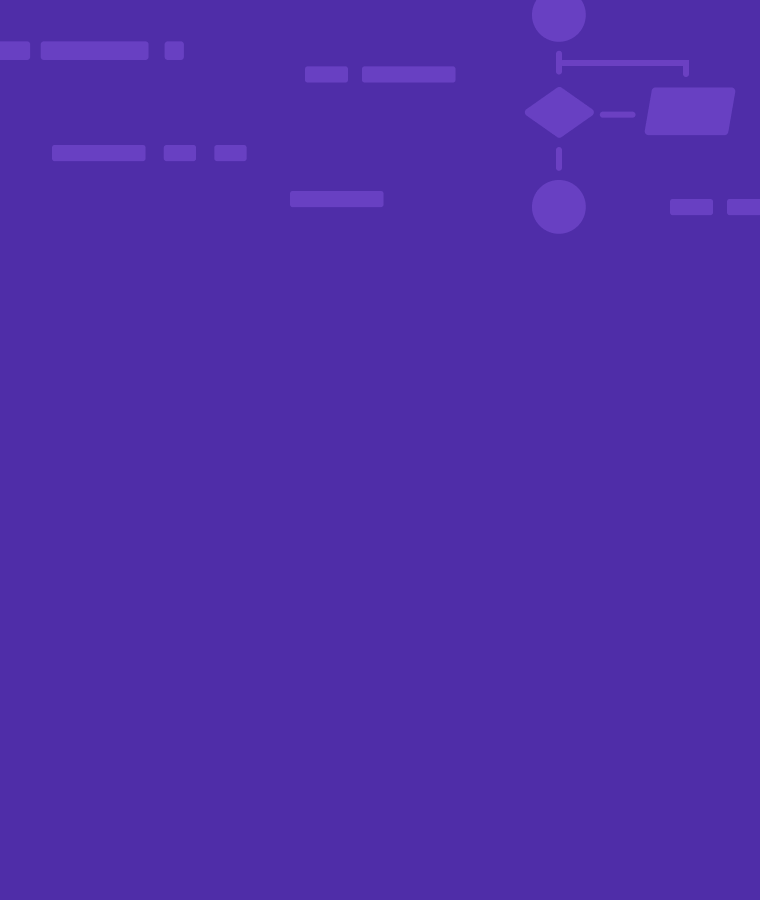
How to use JavaScript Loops?
Programming is like creating a manual for computers and digital devices. In turn, the manual is like the software that computers will run.
When creating a manual, you write out instructions in a step-by-step manner. Let’s say you are creating a manual on how to change a light bulb. The manual instructions could look like the following:
- Place the screw-like end of the light bulb on the receiving hole.
- Rotate the bulb 180 degrees clockwise to screw it inwards.
- Keep rotating the bulb until it is entirely fastened and screwed into its hole.
The above instructions include a loop.
In the case of our example manual, our target condition was to have the light bulb fastened to the wall. The instruction in the loop was to rotate the bulb.
Loops involve repeating an instruction over and over until a given condition is met. To loop is another name for repeating something. Loops usually have a target condition and when that happens, they end.
In this article, you will learn different types of JavaScript loops, and when and how to use them. You will also see practical use-cases of JavaScript loops.
Types of JavaScript Loops
JavaScript is a programming language that browsers can understand. With JavaScript, you can tell the browser what to do on a given website.
When programming in JavaScript, you will identify some steps that need to repeat till some condition is met. Once you identify such repeating steps, use a JavaScript loop.
JavaScript loops continuously run a given block of code until some terminating condition happens. This condition is usually a comparison check that returns either true or false.
In this article, we cover these types of JavaScript loops:
- while loop
- for loop
- for in loop
1.JavaScript while loop
Use a “while loop” to keep running a block of code while a condition is true. The moment a condition becomes false, the JavaScript while loop will stop executing.
This type of loop starts with a while statement followed by the condition in brackets. After the brackets, you place the repeating statements inside braces.
For a simple example, let’s try to write a JavaScript program for fastening the light bulb’s switch. We would have something like the following:
For a simple example, let’s try to write a JavaScript program for screwing a light bulb. We would have something like the following:
while (bulbIsNotFastened) { rotateBulbClockwise(); }
It’s that simple.
If you can’t tell the exact number of times a repetition would take place, use “while loops”. While loops are commonly used in games. Games have some continuous activity that users have to do until something happens.
For example, in shooter games, players are free to move and can be shot. However, the game ends when the player’s life finishes. Let’s say you are building a shooter game. You can use a “while loop” to stop the game when the player’s life finishes. You could use the following:
while (playerStillHasLives) { playGame(); }
The moment the player no longer has any lives — and playerStillHasLives becomes false — the game will stop.
You can have something similar in games where players take turns. For a dealing card game like Crazy Eights, you can have a JavaScript while loop that will keep checking if all players’ hands are not empty.
// in Crazy Eights while (noPlayersHandIsEmpty) { permitNextPlayerToTakeTurn(); }
For chess, you can have a JavaScript while loop that will keep checking if a checkmate happens. Checkmate is when one player wins the game. You want to stop the chess game once checkmate happens (or when checkmate is true). So you can have a while loop that will permit the next player’s turn if the game is not in checkmate.
// in Chess while (!isCheckmate) { permitNextPlayerToTakeTurn(); }
Note: Placing an exclamation mark (!) in front of a boolean (true or false) value, negates that value. So if the value is true, placing ! in front of it will make it false, and vice versa. This is why we used !isCheckmate for the while loop in chess. We only want to permit game play, while checkmate is not true.
do-while loops
JavaScript while loops have another version that rather starts with the do keyword. This variant requires you to place the braces — with the repeating statements inside — before the while keyword.
Use do-while loops when you first need to run the repeating statement before continuously checking for the terminating condition.
For example, when a shooter game starts, the player should have all their lives. So we can’t start playing the game before checking if the playerStillHasLives with the do-while loop. Something like the following:
do { playGame(); } while (playerStillHasLives)
This loop behaves just like the previous ones. The difference is that the first terminating condition check is done after the repeating statement(s) has run once.
2.JavaScript for loop
for loops start with the for keyword.
The similarity between for and while loops is that they both will stop when some target condition takes place. On the other hand, there are two differences between for and while loops.
The first difference is that you use a while loop when you can’t tell the number of times the repetitions will take place. However, you use for loops when you know the number of repetitions ahead of time.
The second difference between them is with contents of their brackets. The brackets of while loops contain only the target condition. In contrast, the brackets of for loops contain 3 items as follows:
- an initializer,
- the target condition itself,
- and an after-iteration.
When writing a for loop, use semi-colons (;) to separate these three items inside the brackets. Something like the following:
for (initializer; condition; after - iteration) { // repeating statements }
The initializer is code that will be run just once, at the beginning of the loop. The after-iteration is a special code that the for loop will run after running the repeating statements (or after looping). This after-iteration is run before the next condition check.
Altogether, the initializer, condition, and after-iteration of for loops use a loop variable. They use this loop variable to configure the loop’s current repetition. This is why you use for loops when you know the number of loop repetitions ahead of time.
Bearing the loop variable in mind, the structure of for loops is usually as follows:
- The initializer will be setting a loop variable to an initial value. E.g. let i = 3 (creates a new variable for the loop and sets its value to 3).
- The condition is usually checking the loop variable against a particular value. E.g. i > 0 (checks if the i variable is greater than 0)
- The after-iteration will change the loop variable (i) to a different value to prepare for the next iteration. E.g. i– (setting i to its current value minus 1)
A good example is when building a countdown. Let’s say we have a website for showing congratulations. And that we want to countdown from 3 to 2, to 1, before popping “Congratulations”. We can use the following JavaScript for loop to achieve that:
for (let i = 3; i > 0; i--) { console.log(i); } console.log('Congratulations'); // 3 // 2 // 1 // Congratulations
for of loops
The for of loop is a variant of the for loop with simpler brackets’ contents.
The difference between for and for of loops is that for loops have 3 different parts whereas for of loops have only one. Also, for of loops have the of keyword and you use for of loops with arrays.
In JavaScript, an array is a list of items. An array is a collection of different elements. To create an array, place its members inside square brackets and separate them with commas. Something like the following:
const countdownSeconds = [3, 2, 1];
That was a simple array containing the countdown seconds: 3, 2, and 1. Now we can use this coundownSeconds array in a for of loop. The for of loop will loop through each member of the array and apply any instructions to each member.
Rebuilding the countdown loop with a for-of loop, we could have the following:
const countdownSeconds = [3, 2, 1]; for (let i of countdownSeconds) { console.log(i); } console.log('Congratulations'); // 3 // 2 // 1 // Congratulations
This for of variant is more elegant than the plain for loop. You don’t need to worry about the loop variable. You simply use an array having in mind that the loop will use each array member.
for of loops are useful with unsorted arrays or a custom list of items. With such custom lists, you are not worried about the order of items — as with the case of the countdown. You rather want all the members of this custom list to be used up.
An example will be to use or list out members of an array. Let’s use the 3 mentioned types of JavaScript loops.
const javaScriptLoops = ['while', 'for', 'for in']; for (let type of javaScriptLoops) { console.log(type); } // while // for // for in
3. JavaScript for in loops
for in loops are special. You use them just as with for of loops but they have two differences.
The first obvious difference is in the keyword. You will have to use in instead of of. Something like:
for (let i in array) { // use i }
instead of
for (let i of array) { // use i }
The second difference is with what the loop “loops” through. for of loops loop through the members of the array while for in loops loop through the positions of those members.
The position of an array member is called its index. The index of an array member tells its current position in the array. The index starts counting from zero, i.e. The first member of an array has index 0 and not 1.
So anytime you want positions or indices (plural for index) of array members, use for in loop. For example, to output the index of each array member, you can use the following:
const javaScriptLoops = ['while', 'for', 'for in']; for (let i in javaScriptLoops) { console.log(i); } // 0 // 1 // 2
Now you have the index of a given array member, how do you obtain that member from that index? Use the square brackets notation. The square brackets notation is a technique for obtaining an array member through 2 steps:
- Place the index of that member inside square brackets.
- Place the square brackets after the array name.
The following code outputs the second member of the array (with index 1).
const javaScriptLoops = ['while', 'for', 'for in']; console.log(javaScriptLoops[1]); // for
Now you have the index and the array member, why would use them together? Sometimes, you might want to list out the array members with numbers attached to them. So instead of attaching the numbers to the array members, you use their indices to obtain their positions.Inside a for in loop, use the square brackets notation to access a given array member with its index. Something like the following:
const javaScriptLoops = ['while', 'for', 'for in']; for (let i in javaScriptLoops) { console.log(i + '. ' + javaScriptLoops[i]); } // 0. while // 1. for // 2. for in
But the index of arrays start counting from 0 and not 1. What do you do if you want to start the list from 1? Display the sum of i and 1. Something like the following:
const javaScriptLoops = ['while', 'for', 'for in']; for (let i in javaScriptLoops) { console.log(++1 + '. ' + javaScriptLoops[i]); } // 1. while // 2. for // 3. for in
Note: Placing double addition sign (++) in front of a variable is the same as adding 1 to its value.
Summary
- Looping is repeating an instruction over and over until a given condition is met.
- Use loops once you identify repeating code and a terminating condition.
- JavaScript loops include
- JavaScript while
- JavaScript for
- JavaScript for-in loops.
- JavaScript while loops for when the number of iterations is not known ahead of time.
- JavaScript for loops for when you know how many times the repetitions should take place.
- JavaScript for in loops for when you want to iterate over the keys of a JavaScript object.
Conclusion
Loops are a great part of JavaScript, but the language also has other important parts like conditionals, functions, operators, etc. Learning is fun when you can learn and play with others. On Sololearn, you do just that. Learn JavaScript for FREE on Sololearn here.