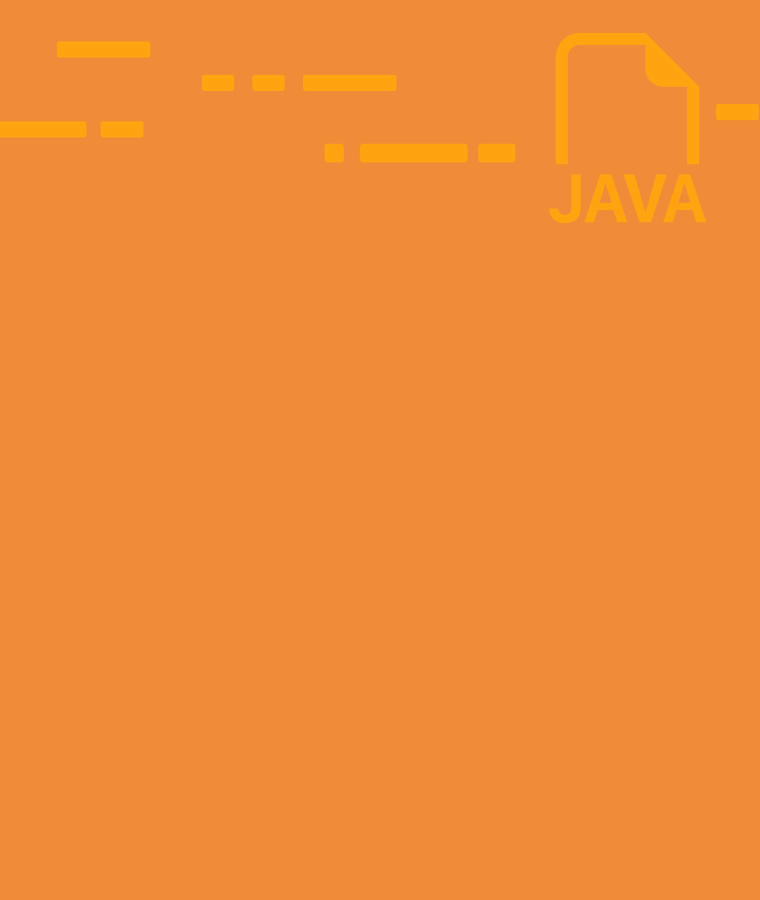
Java Data Types: The Complete Guide To Get You Started
Java provides a variety of data types for the developers to choose from. It enables flexible and easy data manipulation in a program. A data type of a variable tells the compiler the type of value allowed to be stored in it and also defines the size in the memory location.
Defining the data type of a variable has a lot of technical benefits. It becomes convenient for the developer to allocate possible values and perform allowed operations on it, which in turn results in less programming errors and bugs.
For example, a variable created with numeric data type cannot store any alphabetic value. In this case, the developer is restricted to store a numeric value in the variable. If the developer mistakenly tries to store an alphabetic value to a numeric data type, the compiler will not allow it to do so. It will ensure an error free program execution at the end.
If you want to learn about the introduction, you can visit the following URL
www.sololearn.com/learn/courses/java-introduction
Data Types
There are two categories of data types in Java, called primitive and non – primitive.
Primitive
The primitive category provides very basic data types for the variables. There are eight types available in Java.
- int
It is used to store whole numbers ranging from -128 to 127. For example, if we want to store a whole number 5, the following line of code will do the trick
int x = 5;
The size of this variable in memory will be 4 bytes.
- float
It is used to store fractional numbers up to 7 decimal digits, ranging from 1.40129846432481707e-45 to 3.40282346638528860e+38 (positive or negative). A float value can be stored as follows
float x = 5.5555555f;
The size in the memory will be 4 bytes.
- double
It stores fractional numbers up to 15 decimal digits, ranging from 4.94065645841246544e-324d to 1.79769313486231570e+308d (positive or negative).
double x = 55.555;
The size in the memory will be 8 bytes.
- short
It used to store whole numbers and the range is -32,768 to 32,767.
short x = 555;
The size in the memory will be 2 bytes.
- long
It is used to store whole numbers and the range is -9,223,372,036,854,775,808 to 9,223,372,036,854,775,807.
long x = 5555;
The size in the memory will be 8 bytes.
- char
It is used to store alphabetic character.
char x = a;
The size in the memory will be 2 bytes.
- byte
Used to store whole numbers ranging from -128 to 127
byte x = 11;
The size in the memory will be 1 byte.
- boolean
It is used to store true or false value.
boolean x = true;
The size in the memory will be 1 bit.
Let us start with a simple example of adding two numbers and showing their result.
int firstNumber = 2; int secondNumber = 3;
In the above lines, we created two variables firstNumber and secondNumber containing integer values 2 and 3 respectively.
Now we will do manipulation on our two variables. We will add them.
int sum = firstNumber + secondNumber;
We added the variables and stored the result in a new variable sum having the same data type int. In this case, the sum will contain 5.
public class Program { public static void main(String[] args) { int firstNumber = 2; int secondNumber = 3; int sum = firstNumber + secondNumber; System.out.println(sum); } }
You can do coding by yourself to understand the concept. The following URL provides an online compiler.
Non – primitive
These data types are created by the developers. Unlike primitive ones, these are not pre-defined in Java. A non – primitive data type is also called a reference type. The reason being it always refers to an object.
Now what is an object? An object is a collection of multiple primitive data types and some useful functions or methods. These functions are capable of performing computations. For example, we can say that a car is an object. We can map its features, price and number of seats as primitive data types and driving as a useful function.
Now let us discuss the memory location of this reference data type. Let us take the example of the car. When a developer creates an object car in the application, the program creates two things in the memory. One is the reference and the other is the object car to which this reference points to. The reference is created in the heap memory. The object car is created in stack memory.
- String
A string data type contains a sequence of characters surrounded by double quotes. It is used to store names and alphabetic values. For example, to store username and password in a login application, the developer can create the following strings
String username = “new user”; String password = “user password”;
- Array
It is a collection of a same data type. Each member of the array can be accessed using the index technique. Suppose a developer wants to store a list of odd numbers. He or she will create an array as follows
int[] oddNumbers = { 1, 3, 5, 7, 9, 11 };
Each member of the array is called an element. The first element of the array can be accessed by the following line of code.
System.out.println(oddNumbers[0]);
In the similar way, a developer can access any element of the array using the index technique. The output of the above line of code on the console will be 1.
If a developer wishes to know about the length of the array, he or she can do so by the following line of code.
System.out.println(oddNumbers.length);
The output on the console will be 6 for the following code for instance:
public class Program { public static void main(String[] args) { int[] oddNumbers = { 1, 3, 5, 7, 9, 11 }; System.out.println(oddNumbers[0]); System.out.println(oddNumbers.length); } }
- Class
A class in Java provides all the necessary details required to create an object. A class contains data members and functions/methods. It serves as a blueprint for the object.
For example, a developer is creating an application for car leasing. He or she will create a class containing the data members car model, company name and car price. The methods or functions of the class can be driving, monthly installment and insurance plan.
The car model and company name will be string data type, whereas the price will be float. In the driving function, the developer can write code for implementing different driving rules for the car. The monthly installment plan function can include code for calculating monthly installment the customer has to pay. The insurance plan function will include calculations for the insurance of the car.
The following lines of code will create a class car having all the above discussed details
public class car { String companyName; String modelName; float price; public void driving() { // lines of code } public void calculateMonthlyInstalment() { //lines of code } public void calculateInsurance() { //lines of code } }
- Interface
It is similar to a class. It also contains data members and methods. One difference is that the methods or functions do not contain any coding logic. The logic of these functions can be implemented by other classes.
Let us take the previous example of the car. Suppose a developer wants to create a method that builds different model names for different car companies. Some companies might like to include date of manufacturing in the model name, others might like to include a specific code in the model name. So in this case, the class car can contain a method called buildModelName() which will not contain any logic.
interface car { String modelName; float price; public void buildModelName(); }
This interface can be implemented by other classes.
class xyz implements car { public void buildModelName() { // logic for building model name including date of manufacturing } } class abc implements car { public void buildModelName() { // logic for building model name including specific code } }
In this way, interface car is providing abstraction.
Type of variable (Primitive and Non – Primitive)
Sometimes in a program, there comes many scenarios when a developer needs to check the type of a variable. For example, suppose a program checks input given by the end-user (end-user is the person who uses the software). Suppose a program checks whether the input type is numeric or alphabetic. In this case, a developer has to code to identify the type.
There are two functions a developer can use to identify the type of a variable
getClass() this method is of class Object in Java. It fetches the details of the class of the variable.
getSimpleName() it fetches the name of the class for which I is being called.
To check the type of a primitive variable, the following line of code will do the trick
long a; System.out.println(((Object)a).getClass().getSimpleName());
A developer has to cast the variable a to Object because this method is written for reference type variables.
For non – primitive, the following code will be used
car c = new car(); // object of class car from our above example
System.out.println(c.getClass().getSimpleName());
If you wish to learn Java at the intermediate level, you can check the following URL
www.sololearn.com/learn/courses/java-intermediate
Conclusion
In this blog, we have learnt about the main data types in Java. Primitive data types are pre – defined in the language whereas non – primitive are created by the developers. These are actually reference types and they refer to an object. An object is a collection of data members and computational functions. A developer can make comprehensive and useful programs and applications using an object or more than one object. Multiple objects can interact with each other and it will result in a comprehensive and scalable application.