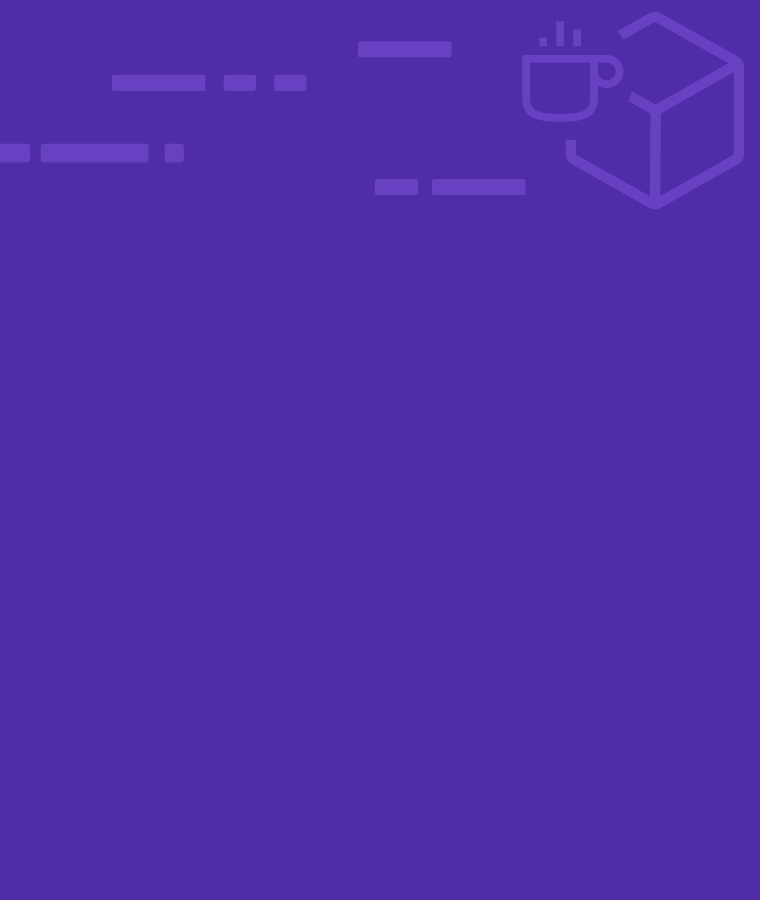
How To Initialize Arrays In Java?
When learning a new programming language, at some point you’ll come across variables. They are used to store data types like the int for integers, float and double for floating point (decimals) numbers, boolean for true or false values, and so on.
But what if you want to store your variables in a group? You can do this with a special data structure known as an array.
Arrays in Java are used to store a collection of variables with similar data types. You can think of this as a container for storing identical entities; like putting a set of blue cups in a box. This means that an array of integers can only have a set of integers stored in it.
Here’s a quick example to show how arrays work:
String language1 = "Java"; String language2 = "Python"; String language3 = "JavaScript";
In the code above, we created three variables to store three programming languages.
With an array, you can do this:
String[] languages = { "Java", "Python", "JavaScript" };
Now we’ve stored all the languages in a single variable called languages.
In this tutorial, you’ll learn how to declare, initialize and access items in an array. Let’s dive in!
What’s an array declaration?
An array declaration in Java means to create an array. To declare one, you have to specify the data type followed by a square bracket and the name of the array.
Here’s an example:
int[] arrInt;
The array above has a data type of int. The name of the array is arrInt.
At the moment, the array has no items in it. In the next section, you’ll see how to initialize/add items to the array.
What does array initialization mean?
To initialize an array means to add items to the array. So to initialize the int array we declared in the last section, we do this:
int[] arrInt = { 2, 4, 6, 8 };
In the code above, we added four integers to the int array — 2, 4, 6, 8. Items stored in an array are nested in curly brackets, and each item is separated from the next using commas.
Now that we’ve added items to the array, we can access each item using its index number. Under the hood, each item in an array has a number attached to it depending on its position.
The first item in an array has an index of 0, the second has an index of 1, the third has an index of 2, and so on. To show that using our int array, you’d have something like this:
Index 0 => 2
Index 1 => 4
Index 2 => 6
Index 3 => 8
Here’s a code example:
int[] arrInt = { 2, 4, 6, 8 }; System.out.println(arrInt[0]); // 2 System.out.println(arrInt[1]); // 4 System.out.println(arrInt[2]); // 6 System.out.println(arrInt[3]); // 8
To access an item in an array, you start by writing the array’s name followed by the index of the item in square brackets.
To access the first item in the arrInt array, we did this: arrInt[0]. The System.out.println() function was used to print out the value to the console.
In the same manner, you can change or change the value of an item in an array using its index. Here’s an example that shows how to change the third item in our array:
int[] arrInt = { 2, 4, 6, 8 }; arrInt[2] = 10; System.out.println(arrInt[2]); // 10
Using the index arrInt[2], we assigned a value of 10 to the third item.
So far, we have been printing each value individually. If you want to print or do something with all the items in an array, you can use a for loop. Here’s an exam:
int[] arrInt = { 2, 4, 6, 8 }; for (int i = 0; i < arrInt.length; i++) { System.out.println(arrInt[i]); // 2 // 4 // 6 // 8 }
Conclusion
At this point, you know how to create, add, access, and change items in a Java array.
Want to learn about more interesting Java topics? Check out Sololearn’s Java course.
Happy coding!