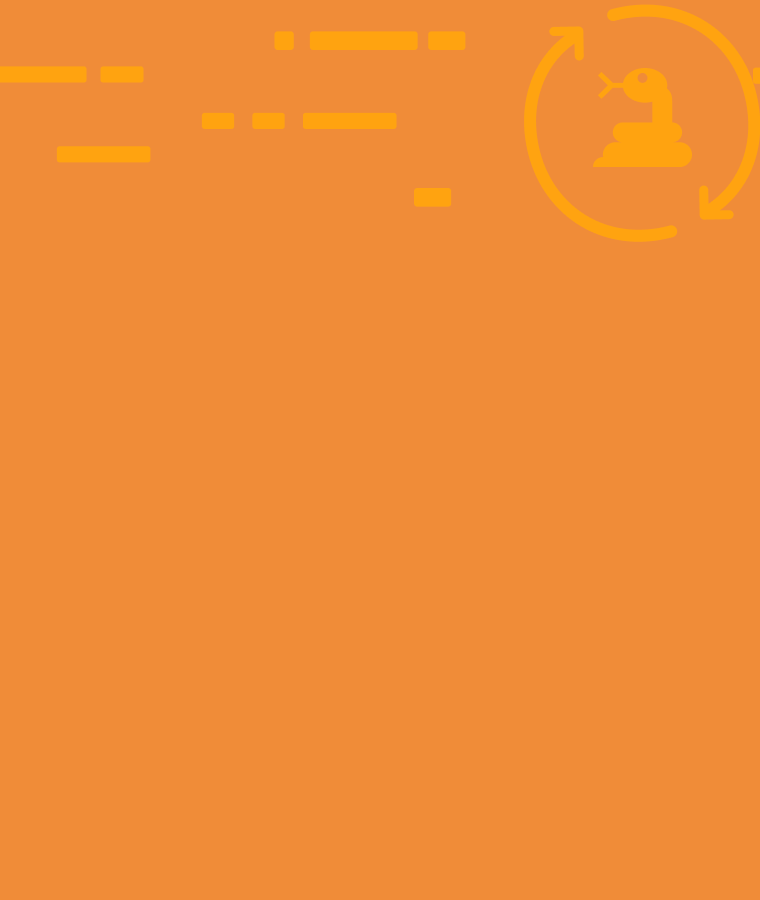
Python For Loop
Many times, we need to repeat certain processes on a specific task. The repetition of these processes is called loops. They are very important in programming to execute tasks effectively.
We ask the process to repeat the same operations on different values for a specific task to be solved.. In python, we can make use of “for” loops when we want to repeat some operations on different values in a program. A python for loop accesses values from a data sequence (called iterables because they are the elements we work with). This sequence of data can be a string, tuple or list.
Syntax Of a For Loop
We start with the “for” keyword, then a < variable_name> that stores the values accessed by the loop, then the “in” keyword to access the actual data we are getting values from <sequence_data(iterables)> and end the statement with a colon “:”.
After that, we pass in the “statements” the instructions we want the loop to perform.
for <variable_name> in <sequence_data(iterables)> : statements
Note: the indentation is important when writing statements in the code block . If you are making use of some code editors they may indent it automatically otherwise, you click on the “tab” key once from the start of the line.

- The python “for” loop is an iterator that goes through items in a data sequence, it picks an item and checks if that is the last or only item in the sequence.
- If it is not the only item in the data sequence, it repeats the same process till it gets to the last item in the data sequence and exits the loop.
“For loops” can loop over iterators like lists, tuples, string, range, and even generators. Now that we know the format of the “for” loop, we look at some examples working with three data sequences; strings, lists and tuples.
Example I – Looping through a string.
- First, we have the variable that stores the string we want to loop through(iterables)
- Second, we type in the “for” keyword, then assign a variable that will store the accessed values from the string
- Then type the “in” keyword and the name of the variable that stores the string, followed by a colon “:”
word = "firsttry" for char in word:
In the above code block; <char> is the name of the variable that stores the assessed value from the loop, while <word> is the name of the variable that we want to loop through. The statement will be anything we pass on the next line, let’s print the variable <char> to see the result.
word = "firsttry" for char in word: print(char) # result # f # i # r # s # t # t # r # y
In the above code block, we printed <char> and all the values from the strings were printed out. Till all the letters in the string were printed, the loop did not stop. Now, let’s try to add the letter “a” to each of the values from the string.
word = "firsttry" for char in word: print(char + "a") # result # fa # ia # ra # sa # ta # ta # ra # ya
It ran pretty smooth and added “a” to each values
Example II – with List
- First, we have the variable that stores the list we want to loop through(iterables)
- Second, we type in the “for” keyword, then assign a variable that will store the assessed values from the list.
- Then type the “in” keyword and the name of the variable that stores the list, followed by a colon “:”
fruit_basket = ["orange", "apple", "banana","grapes"] for fruits in fruit_basket: print(fruits) # Result # orange # apple # banana # grapes
In the above code block; <fruits> is the name of the variable that stores the assessed value from the loop while <fruit_basket> is the name of the variable that we want to loop through. The statement will be anything we pass on the next line, and we can see the results printed.
Let’s say we want to print a statement that says “i love <fruits>”.
fruit_basket = ["orange", "apple", "banana","grapes"] for fruits in fruit_basket: print("I love " + fruits) # Result # I love orange # I love apple # I love banana # I love grapes
In the above code, we can see the phrase “I love” is repeated the number of times the fruits exist in the fruit basket. No fruit was repeated twice.
Lists containing numbers
list_of_numbers = [1, 4, 6, 8, 9] for numbers in list_of_numbers: print(numbers) # Result # 1 # 4 # 6 # 8 # 9
Let’s say we want each number to be multiplied by 2.
list_of_numbers = [1, 4, 6, 8, 9] for numbers in list_of_numbers: print(2 * numbers) # Result # 2 # 8 # 12 # 16 # 18
We see the results have been multiplied by 2.
Example III – with tuples
- First, we have the variable that stores the tuple we want to loop through(iterables)
- Second, we type in the “for” keyword, then assign a variable that will store the assessed values from the tuple.
- Then type the “in” keyword and the name of the variable that stores the tuple, followed by a colon “:”
color_box = ("Red", "Yellow", "Blue", "Purple") for colors in color_box: print(colors) # Result # Red # Yellow # Blue # Purple
In the above code block; <colors> is the name of the variable that stores the assessed value from the loop while <color_box> is the name of the variable that we want to loop through. The statement will be anything we pass on the next line, and we can see the results printed.
Let’s say we want to print a statement that says “<colors> is very pretty”.
color_box = ("Red", "Yellow", "Blue", "Purple") for colors in color_box: print(colors + " is very pretty ") # Result # Red is very pretty # Yellow is very pretty # Blue is very pretty # Purple is very pretty
We can see in the above examples that all items are accessed in the loops and you can pass in any statement to modify its results.
The range() function
The range() function is another method of looping through items in a data sequence. It returns a range of numbers using a starting value(optional) and a stopping value. A step value is optional, but the default is 1.
Format
<range(stop)> <range(start, stop)> <range(start, stop, step)>
- start: specifies the number the range starts from.
- stop: specifies the end of the range. This value would not be included in the range.
- step: this value specifies the increment in the numbers.
Example:
We are to find a range of 5 numbers; we can do this in two ways;
The first way is giving a start value of 0 and a stop value of 5. It becomes;
range(0,5), the numbers are; 0, 1, 2, 3, 4.
It stops at 4 because, in the range, the last number is not included, but when you count the numbers, they are 5 in total.
The second way is passing in 5 in the range, the start value is assumed to be 0. It becomes;
range(5), and the numbers are: 0, 1, 2, 3, 4
If we are to increase each number by 2, that is the step value. It becomes;
range(0,5,2), the numbers are 0, 2,4
Implementing range in for loop
“For” loop and the range() function goes hand in hand. The process needs to be repeated to get the numbers from a specific range.
Syntax
for <variable_name> in range(stop): statement Or for <variable_name> in range(start,stop): statement Or for <variable_name> in range(start, stop, step): statement
Examples
Let’s find the range of 5 numbers.
for num in range(5): print(num) # Result # 0 # 1 # 2 # 3 # 4
We can see that the numbers started from 0 and ended at 4.
Let’s add a starting range value
for num in range(1, 10): print(num) # Result # 1 # 2 # 3 # 4 # 5 # 6 # 7 # 8 # 9
We see in the above code, the start value is 1, the stop value is 10 but the last number is 9. The range of the values is 1 – 9
Let’s add a step value
for num in range(1, 10, 2): print(num) # Result# 1# 3# 5# 7# 9
In the above code block, the step value is 2, the numbers printed are increased by 2 every time.
Iterating By Sequence Index
Apart from looping through lists and tuples, we can access elements using the indexing from the range() function.
Indexing = variable_name[index_number]
Example
fruit_basket = ["orange", "apple", "banana", "grapes"] print(fruit_basket[0]) # orange print(fruit_basket[1]) # apple print(fruit_basket[2]) # banana print(fruit_basket[3]) # grapes color_box = ("Red", "Yellow", "Blue", "Purple") print(color_box[0]) # Red print(color_box[1]) # Yellow print(color_box[2]) # Blue print(color_box[3]) # Purple
Instead of entering the numbers manually, we can set a range. Lists and tuples have an element at 0, which will be our start value, and our stop value will be the length of our list.
Format
for variable_name in range(0, len(list)):
Or
for variable_name in range(len(list)): # recall that start value by default is 0 using this format
Let’s print the length of the list to know the stop value
fruit_basket = ["orange", "apple", "banana","grapes"] print(len(fruit_basket)) # 4
The output is 4, therefore, our stop value is 4. When working with range, the last number is not added. The loop starts at 0 and ends at 3.
fruit_basket = ["orange", "apple", "banana", "grapes"] count_of_fruits = len(fruit_basket) for fruit_index in range(count_of_fruits): print(fruit_index) # Result # 0 # 1 # 2 # 3
In the above code block, the numbers are printed out. To access the elements, we make use of indexing <actual_variable_container[variable_name_storing_numbers]>. In this code sample, the format is;
<fruit_basket[fruit_index]>
We have;
fruit_basket = ["orange", "apple", "banana", "grapes"] count_of_fruits = len(fruit_basket) for fruit_index in range(count_of_fruits): print(fruit_basket[fruit_index]) # Result # orange # apple # banana # grapes
The same process applies to tuples,
color_box = ("Red", "Yellow", "Blue", "Purple") count_of_colors = len(color_box) for color_index in range(count_of_colors): print(color_box[color_index]) # Result # Red # Yellow # Blue # Purple
I can also set a start value for the list or tuple.
color_box = ("Red", "Yellow", "Blue", "Purple") count_of_colors = len(color_box) for color_index in range(1, count_of_colors): print(color_box[color_index]) # Result # Yellow # Blue # Purple
The start value starts from index 1 and ends at index 3.
Using conditional statements in For loop
In for loops, we can have conditional statements in the code block.
Format
for <variable_name> in <sequence_data(iterables)> : conditional statement: print()
In the format above, we see that the conditional statement is indented under the for loop and ended with a colon “:” and the print statement is indented under the the conditional statement.
The print has to be inside the code block of the conditional statement because if the condition is true , it returns the output, if it is not it does not return any output.
What happens if you put the print() statement outside the indent of the conditional statement?
for <variable_name> in <sequence_data(iterables)> : conditional statement: print()
Or
for <variable_name> in <sequence_data(iterables)> : conditional statement: print()
If the print() statement is put on the same indent as the for loop or same indent as the conditional statement, it throws an error saying there is an issue with the indentation.
Examples
Exercise I: in a list containing numbers print the numbers less than 5.
list_of_numbers = [1, 4, 6, 8, 9] for numbers in list_of_numbers: if numbers > 5: print(numbers) # Result # 6 # 8 # 9
In the above code block, we see that the condition is to check if any number is greater than 5, after that we indented the print statement under the conditional statement “if” and the output is returned when the condition is true.
If the print statement is brought outside the if statement( on the same indent as the if statement) or on the same indent as the for keyword, it throws an error.
list_of_numbers = [1, 4, 6, 8, 9] for numbers in list_of_numbers: if numbers > 5: print(numbers) # Result # print(numbers) # ^ # IndentationError: expected an indented block
Or
list_of_numbers = [1, 4, 6, 8, 9] for numbers in list_of_numbers: if numbers > 5: print(numbers) # Result # print(numbers) # ^ # IndentationError: expected an indented block
II : print out fruits that have the letter “e” in their names.
fruit_basket = ["orange", "apple", "banana","grapes"] for fruits in fruit_basket: if "e" in fruits: print(fruits) # Result # orange # apple # grapes
In the fruit basket, we see 4 fruits but in the result, we see 3 fruits. The conditional statement checks if the letter “e” is in each name of the fruit. “Banana” is not printed because there is no letter “e” in the word.
III: print out only numbers lesser than 6 in the range of 20 numbers.
for numbers in range(1,20): if numbers < 6: print(numbers) # Result # 1 # 2 # 3 # 4 # 5
We see in the result above that the numbers returned are less than 6.
Break Statement
Loops are repetitive and the process continues. When a break statement is introduced, the loop ends and the execution stops.
Format
for <variable_name> in <sequence_data(iterables)> : conditional statement: break print()
The “break” statement is indented under the conditional statement and the print statement is on the same indent as the conditional statement. The “break” tells the loop to end the operation and the result printed are all the values before the break statement is used.
Examples
I
for numbers in range(1,20): if numbers == 6: break print(numbers) # Result # 1 # 2 # 3 # 4 # 5
In the above code block, the condition says when the variable <numbers> is equal “==” to “6”, end the loop “break” and the remaining numbers are printed. The double equals to sign “==” is used to compare values on the lefthand side and righthand side. The result shows 1 to 5, those numbers are not equal to 6 that is why they are printed.
II
color_box = ("Red", "Yellow", "Blue", "Purple") for color in color_box: if color == "Blue": break print(color) # Result # Red # Yellow
In the above code block, the condition says when the variable <color> is equals to “Blue” at the end of the loop, the colors before “Blue” are printed.
Continue statement
When the “Continue” statement is used in the for loop, the loop skips that iteration if the statement is true. The continue statement is placed after the conditional statement. The results printed are values after the conditional statement.
Format
for <variable_name> in <sequence_data(iterables)> : conditional statement: continue print()
Example
I
for numbers in range(0,20): if numbers % 3 == 0: continue print(numbers) # Result # 1 # 2 # 4 # 5 # 7 # 8 # 10 # 11 # 13 # 14 # 16 # 17 # 19
In the above code block, the condition statement says when the variable <numbers> is divided by “3” and the remainder is 0 , the continue statement follows. Every time a number is divided by 3 and the remainder is 0, the number is skipped to the next.
II
fruit_basket = ["orange", "apple", "banana","grapes"] for fruits in fruit_basket: if "an" in fruits: continue print(fruits)
We can see that any fruit that has “an” in its spelling is skipped and the rest is printed.
Else in For Loop
e “else” statement es the exception in the loop. When the condition passed in the loop is false, the “else” statements handle it.
Format
for <variable_name> in <sequence_data(iterables)> : conditional statement: print() else: print()
Example
I
for numbers in range(1,10): if numbers % 3 == 0: print(numbers) else: print("Not divisible by 3") # Result # Not divisible by 3 # Not divisible by 3 # 3 # Not divisible by 3 # Not divisible by 3 # 6 # Not divisible by 3 # Not divisible by 3 # 9
In the code block above, the else statement tackles the result of the condition when it is false. Let’s take the value “1”; when divided by 3, it does not give a reminder of 0; therefore the condition is false and the else statement is checked. For values that are not divisible by 3, print “Not divisible by 3” instead of the number. That is why the numbers not divisible by 3 have the phrase printed in the console.
II
fruit_basket = ["orange", "apple", "banana","grapes"] for fruits in fruit_basket: if "an" in fruits: print(fruits) else: print("This is a fruit") # Result # orange # This is a fruit # banana # This is a fruit
In the code block, the condition says if the fruits have the letter “an” in their spelling they should be printed; if not(for the else statement), the phrase “This is a fruit” is printed instead.
Nested Loops
A Nested loop consists of another “for” loop to access elements in a data sequence.
Format
for <variable_name> in <sequence_data(iterables)>: for <new_variable_name> in <variable_name>: statement
Example
Result using one “for” loop.
fruit_basket = ["orange", "apple"] for fruits in fruit_basket: print("Fruit - " + fruits) # Result # Fruit - orange # Fruit - apple
Nested loop
fruit_basket = ["orange", "apple"] for fruits in fruit_basket: for letters in fruits: print("Letter - " + letters) # Result # Letter - o # Letter - r # Letter - a # Letter - n # Letter - g # Letter - e # Letter - a # Letter - p # Letter - p # Letter - l # Letter - e
In the code block, we see that the first time we looped through the items in the basket, the result was the fruit names. Now we loop through each fruit name with a new variable <letters> to get the new result.
Conclusion
Loops make the execution of tasks simpler and faster. You can play around with it to get familiar with it. Remember to practice to master the use of loops. To learn more about python for loops, check here.