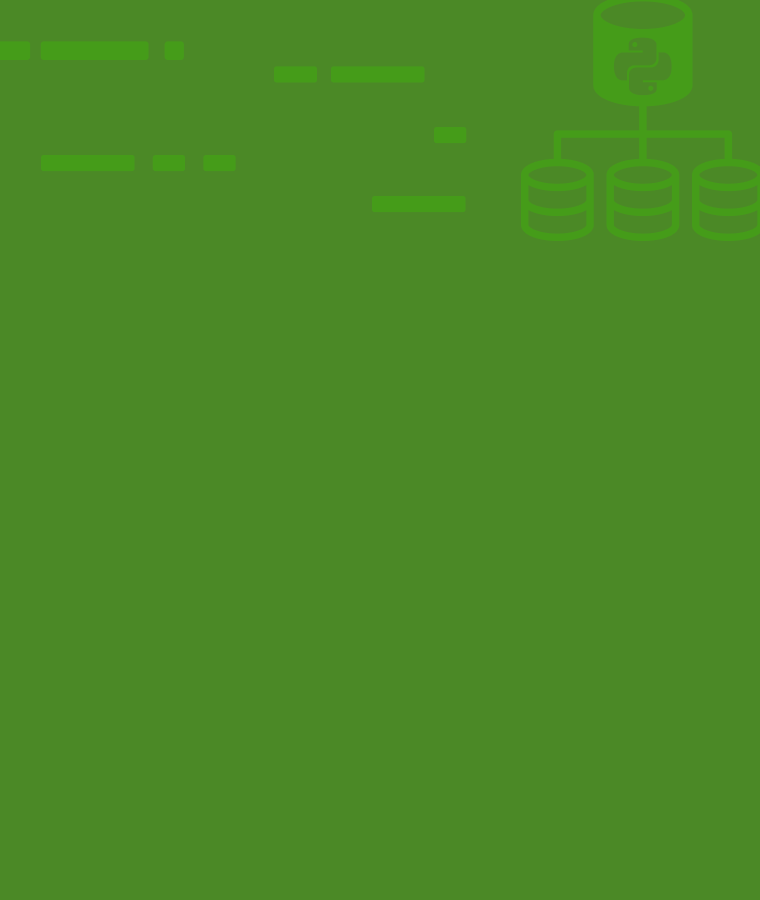
Ultimate Guide to Python Data Structures
Python is a popular, open-source, and free high-level programming language. It was created by Guido van Rossum and first released in 1991. This article aims to discuss user-defined and built-in data structures in Python. So without further ado, let’s begin.
According to a Statista survey conducted in the year 2021, Python was the third most used programming language among developers worldwide. The popularity of the Programming Language (PYPL) index ranks Python as the most popular programming language as of Mar 2022.
Developers around the world use this language in web development, data science, artificial intelligence, software development, and prototyping.
Python support built-in as well as user-defined data structures. In this article, we will discuss different types of Python data structures.
Why do we use Data Structures?
Data structures are specific ways to organize and store data for efficient access and processing.
All data structures contain data values, the relation between the stored values, and in some cases, functions applicable to the data.
The following rationale highlights the importance of using data structures.
- Data structures are the building blocks of software programs and applications.
- Many efficient algorithms are based on data structures. They enable the handling and managing of vast amounts of data, such as integrated database collections.
- Data structures are also the determining factor of your code’s responsiveness and runtime.
Some of the use cases of data structures are listed below;
- Data storage
- Resource and service management
- Data exchange
- Data sorting
- Data indexing
- Data search
- Data scalability
Types of Data Structures in Python
Built-in Data Structures
These data structures are integrated within the Python language, offering ease and speed to programmers in finding solutions.
- List
Lists are built-in Python data structures that can store heterogenous data sequentially. Every element in the list can be accessed by an address called index.
Python lists allow data access in forward (positive indexing) and reverse direction
(Negative indexing). Positive indexing fetches data from the start till the end, and negative indexing fetches data from last to first. Lists can be modified by adding new elements, updating, and deleting existing elements.
The following code snippet creates a heterogeneous Python list with integer and string data elements.
myList = [1000, 30, "apple", "oranges"] print(myList)
Output:
[1000, 30, apple, oranges]
clear(), copy(), extend(), index(), pop(), reverse() and sort() are some of the list functions available in Python.
- Tuple
Tuples are immutable python data structures similar to lists except for the feature that the elements in the tuple cannot change in any way. Tuples do not offer the extensive functionality that a list class provides; thus are used in scenarios where data does not need alterations.
myTuple = ("January", "February", "March", "April", "May", "June") prunt(myTuple)
Output:
'January', 'February', 'March', 'April', 'May', 'June'
- Dictionary
Dictionary stores data in key-value pairs. Best suited in cases where data is continuously mutating. Keys are the names logically denoting the elements they are associated with, called values. Keys are immutable, but the values can be either mutable or immutable. Key-value pairs in a dictionary are not organized in any specific manner. Data is accessed based on unique keys.
myDictionary1 = {1: "Java", 2: "PHP", 3: "Python", 4: "SQL"} myDictionary2 = {"First": "Java", "Second": "PHP", "Third": "Python", "Fourth": "SQL"} print(myDictionary1) print(myDictionary2)
Output:
{1: "Java", 2: "PHP", 3: "Python", 4: "SQL"} {"First": "Java", "Second": "PHP", "Third": "Python", "Fourth": "SQL"}
- Set
Sets are a collection of unique unordered elements. Elements are not indexed in sets; either an element is present in the set or not. Sets in Python data structures are similar to arithmetic sets with the same operations. An element is added only once in the set, even if it repeats. Sets are used in scenarios where uniqueness is preferred to repetition.
mySet = {"Monday", "Tuesday", "Wednesday", "Thursday", "Friday", "Saturday", "Monday"} print(mySet)
Output:
{'Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday', 'Saturday', 'Monday'}
clear(), pop(), copy(), difference(), discard(), intersection(), issubset(), issuperset() are some of the set functions.
User-defined Data Structures
Programmers can create their own data structures in Python, known as user-defined data structures. User-defined data structures are supported by Python to reflect the same functionality as that of structures not supported by Python. Following are widely implemented types of user-defined Python data structures.
- Stack
Stacks are the linear data structures based on LIFO (Last In First Out) or FILO (First In Last Out). Data entered last would be accessed first in the case of LIFO, whereby in FILO, data entered first would be accessed last.
Stacks are based on array structures. Elements are added and removed from a single point in the stacks. You can access the elements from one point called the Top. It is also an indicator of the current position of the stack.
myStack = ["blue", "black", "orange"] print(myStack)
Output:
[blue, black, orange]
append(), pop(), empty(), size(), and top() are some of the operations performed on stacks.
Stacks are most often used in applications like recursive programming, reversing words, and undo mechanisms in word editors.
- Queue
A queue is another linear data structure operating on FIFO (First In First Out). Data entered first in the queue is accessed first. Just like a queue of passengers at an airport. Operations are performed from both ends of the queue, known as front-back or head-tail. Queues are built using array structures. Adding (En-queue), deleting (De-queue), and accessing elements are some of the functions you can perform on queues.
Queues are used as network buffers to manage and control traffic congestion on an app. Additionally, it’s also utilized in running operating systems to schedule processes.
myQueue = ["winter", "summer", "autumn", "spring"] print(myQueue)
Output:
['winter', 'summer', 'autumn', 'spring']
- Linked List
Linked lists are linear data structures with an ordered collection of elements in the form of nodes. Linked lists use pointers to connect their elements. Nodes have two fields called Data and Next. Data contains the stored value and Next is the reference pointer to the next node in the list.
The first node of the list is called the head. Any iteration through a linked list begins from the head node. Tail is the last node having its pointer referencing Null. Image viewing, music player applications, and similar media programs employ these data structures.
- Tree
A tree is a nonlinear data structure storing data with a hierarchy. It usually starts from the top to the branches at the bottom. Several levels in the tree structure denote the depth of data.
All data originates from the top node, called root. The nodes at the bottom are called leaf nodes. A node that precedes is known as the parent, and the node that follows is called the child for obvious reasons. Trees are used to store data connected non-linearly but have a hierarchy such as HTML pages that use trees to differentiate which tag comes under which block.
- Hashmap
Hashmaps are indexed nonlinear data structures operating on the hash function to generate keys and match with their value. The hash function evaluates the index and links the key to its value in the bucket. Keys are unique and immutable. Hash mapping allows quick access to elements, and its efficiency depends on the hashing function.
- Graph
Another nonlinear user-defined data structure is graph storing data as a collection of nodes. Graphs use a pair of vertices (data points) and edges (links). Each edge connects a pair of vertices. Graphs represent networks and are synonymous with real-world maps; therefore, find applications in Google Maps, Uber, telephone, and social networks providing solutions to real-life problems.
Python Data Structure Operation
Following is the list of operations performed on Python data structures to manipulate data.
- Insertion
Insertion is the operation to add elements to a data structure. Insertion can be unsuccessful in cases when the data structure is full, leaving no space for further addition. This condition is known as Overflow.
This operation applies to all data structures and increases the size of the data structure. The addition of an element in the queue is done with the enQueue() function, and in the stack, it is done via push() functions. Linked lists and arrays allow the addition of an element at any location, while stacks and queues only insert elements at a specific end. Elements in the graph and tree can be added randomly.
- Deletion
The deletion operation removes an existing element from the given data structure and applies to all data structures. Underflow is the condition where deletion operation is implemented on a data structure with no elements. This operation reduced the size of the data structure it is implemented in.
In queues, it is implemented with deQueue() function and in stacks pop() function performs this operation. Linked lists and arrays allow deletion of an element at any location, while stacks and queues only insert elements at a specific end. Elements in the graph and tree can be deleted randomly.
- Searching
As the name suggests, this operation is used to find a particular element stored in the data structure. It gives the location of a data element in the data structure. A key value is needed to implement the search operation. This key value is used to match the stored values, and upon successful matching, the location of the value is returned. Otherwise, the null location is received.
- Sorting
This operation arranges the elements of data structure either in ascending or descending order in the case of numerical data and alphabetically.
- Merging
Merging combines the data elements of two data structures into a single data structure. It appends the elements of one data structure after the elements of another data structure with the same structure. The resulting data structure may be sorted or not. Merge operation can also combine the elements of two data structures with different structures.
- Traversing
Applicable to all data structures, traversing is the operation of going through all the elements available in a data structure once. This operation is implemented by a pointer that indicates the current element in a data structure.
Wrapping Up Python Data Structures Guide
Now that you have a comprehensive background of the data structures usability and details, you can hop aboard our course “Python for Beginners“. Here, you can start building your skills set in Python language by solving real-life problems. The best part? You don’t need to have prior experience with programming languages.