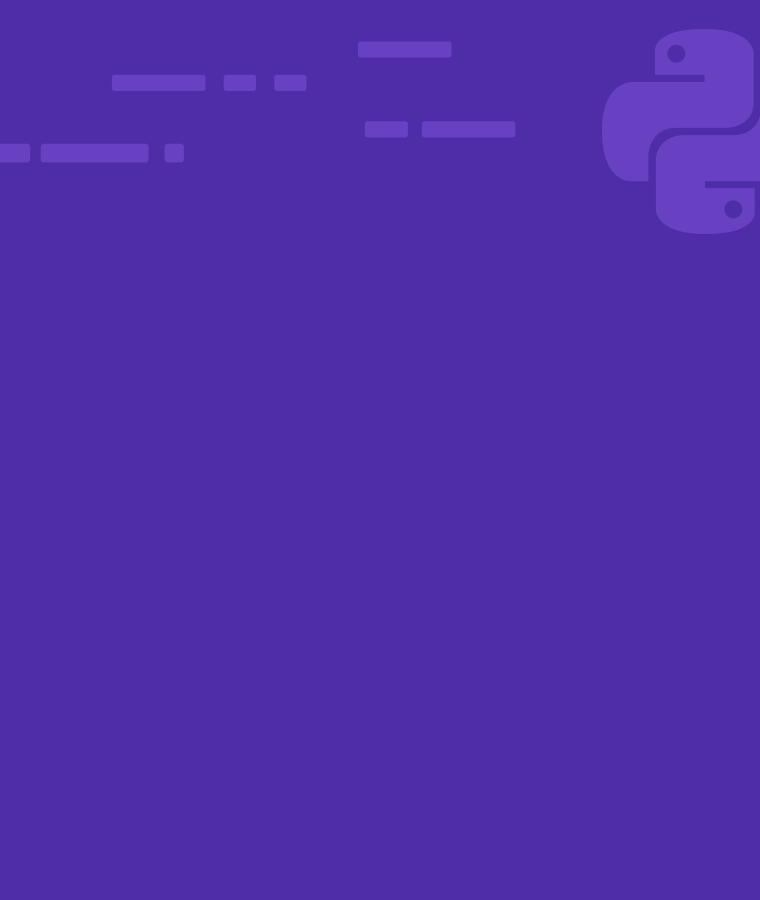
Floating numbers in Python
Data has different types, it can be stored in. The main types are numbers, strings, list, tuples, set and dictionaries. In this article, we will focus on floating numbers, one of the form numbers that can be stored.
Floating numbers have numbers with a whole part and a fractional part separated by a decimal point(.) These numbers are not integers.
wholepart.fractionalpart
How to obtain floating numbers
By storing a number with a decimal point in a variable and printing it out.
float_number = 13.45 print(float_number) # >> 13.45
We can also check the type the variable belongs to using the type notation “type()”.
float_number = 13.45 print(type(float_number)) # >> <class 'float'>
By dividing integers
maths_score = 12 science_score = 20 english_score = 30 mean_score = maths_score + science_score + english_score / 3 print(mean_score) # >> 42.0 print(type(mean_score)) # >> <class 'float'>
We can see in the code block above that the output is <42.0>, the whole part is 42 and the fractional part is 0.
By using the inbuilt float() method
float_num = float() print(float_num) # >> 0.0 print(type(float_num)) # >> <class 'float'>
Converting other data types to Float Using the inbuilt method float().
Data types like integers, strings and boolean can be converted to floats.
Example I – with integers
Integers are whole numbers, examples; 13, 40, 15, 60
integer_number = 13to_float_num = float(integer_number)print("This is a floating number" , to_float_num) # >> 13.0
In the above code block, the result is “13.0”, <13> is the whole part, <0> is the fractional part, and it is separated by a decimal point<.>. Let’s check the types of the variables
integer_number = 13 to_float_num = float(integer_number) print("Type for the variable - integer_number: ") print(type(integer_number)) # >> Type for the variable - integer_number: # >> <class 'int'> print("Type for the variable - to_float_num: ") print(type(to_float_num)) # >> Type for the variable - to_float_num: # >> <class 'float'>
Example II – with strings
Strings that contain numbers (“40”, “40.345”) and strings that contain some characters like: “infinity”, “inf”, “Nan” and “nan” are converted to floats apart from these examples of strings, the rest throw an error message.
- Strings with numbers
string_num = "30" to_float = float(string_num) print(to_float) # >> 30.0 print(type(to_float)) # <class 'float'> string_num1 = "50.555555" to_float1 = float(string_num1) print(to_float1) # >> 50.555555 print(type(to_float1)) # <class 'float'>
- Strings with characters; “infinity”,”inf” and “nan”
When the characters “infinity” or “inf” no matter the case of the letters(lower or upper), the output is <inf>
string_text = "infinity" string_text_alt = "inf" to_float = float(string_text) to_float_alt = float(string_text_alt) print(to_float) # >> inf print(to_float_alt) # >> inf print(type(to_float)) # <class 'float'> print(type(to_float_alt)) # <class 'float'>
When the characters “NAN” or “nan” no matter the case of the letters(lower or upper), the output is <nan>
string_text1 = "nan" string_text_alt1 = "NAN" to_float1 = float(string_text1) to_float_alt1 = float(string_text_alt1) print(to_float1) # >> nan print(to_float_alt1) # >> nan print(type(to_float1)) # <class 'float'> print(type(to_float_alt1)) # <class 'float'>
Other strings with non-numeric characters would not be converted to float
string_text = "Float number" to_float = float(string_text) print(to_float) # >> could not convert string to float: 'Float number'
Example III – with booleans
Booleans have two statement: <True> or <False>. <True> when converted to float the output is <1.0> while <False> becomes <0.0>
bool_true = True bool_false = False to_float = float(bool_true) to_float1 = float(bool_false) print(to_float) # >> 1.0 print(to_float1) # >> 0.0 print(type(to_float)) # <class 'float'> print(type(to_float1)) # <class 'float'>
Note: “True” is not the same as <True> the first is a string and it will throw and error, same as “False” and <False>
Example IV – from the input() method
This method is a substitute for numbers , infinity and nan in strings, any other from these strings will throw an error.
float_value = float(input("Enter a number or infinity or nan to convert to float: ")) print(float_value) # >> 30.908 print(type(float_value)) # >> <class 'float'>
Arithmetic Operations
Floating numbers can perform all four main operations; addition, subtraction, multiplication and division.
- Addition
float_num_1 = 20.34 float_num_2 = 2.0 add_float = float_num_1 + float_num_2 print(add_float) # >> 22.34
- Subtraction
float_num_1 = 20.34 float_num_2 = 2.0 sub_float = float_num_1 - float_num_2 print(sub_float) # >> 18.34
- Multiplication
float_num_1 = 20.34 float_num_2 = 2.0 multiply_float = float_num_1 * float_num_2 print(multiply_float) # >> 40.68
- Division
float_num_1 = 20.34 float_num_2 = 2.0 divide_float = float_num_1 / float_num_2 print(divide_float) # >> 10.17
Conclusion
Floating numbers are an integral part of real-world computation. In finance for money, in engineering for precision control, and in various fields for different purposes. Therefore, It is important to understand how they work and how they can be used. To know more about floats in the python programming language, check here.