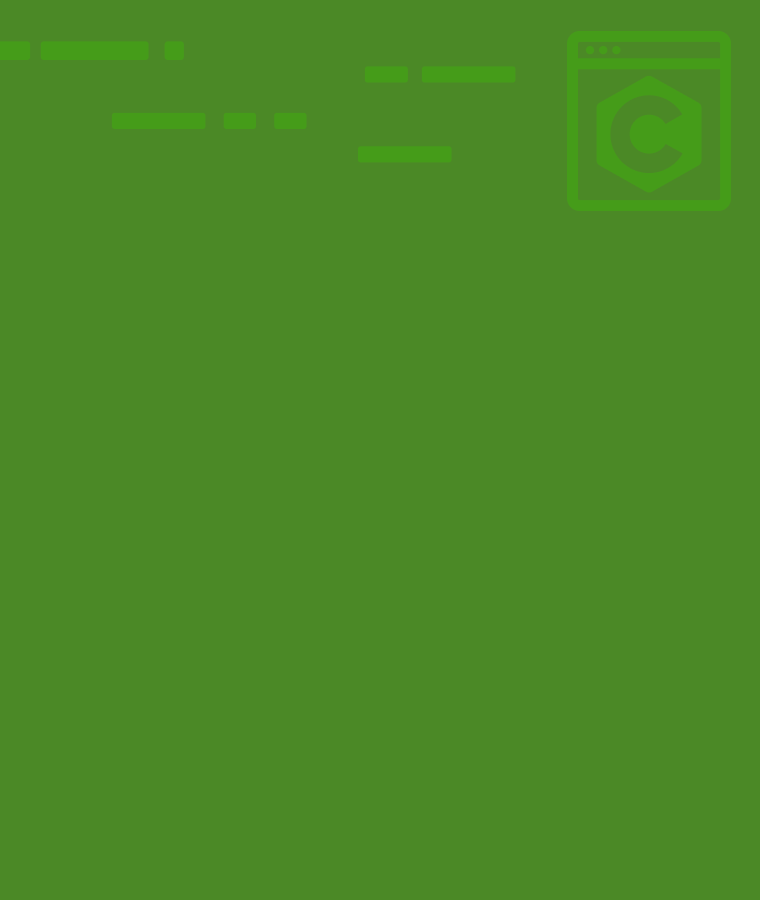
A Guide From Scratch to Learn C
Are you wondering whether learning C is a good decision or not? Don’t think twice, you are on the right track to becoming an expert programmer.
Despite the predominance of modern programming languages, C still maintains its importance in the tech world. C powers the technologies we use daily and will continue to remain active for a long time. Be it operating systems (Windows, Mac, Linux, or mobiles) or embedded systems like the cash register, temperature sensors, and vending machines, all are mostly written in C.
According to the TIOBE index 2022, C ranks 2nd on the list of most popular programming languages in the world.
Dennis Ritchie developed C between 1969 and 1973 to run applications on the UNIX operating system. C gained popularity during the 1980s with compilers for almost all modern operating systems.
C is the fundamental programming language. It’s recommended for aspiring programmers to learn C as their first programming language. C helps you understand the operating system’s inner workings that drives programming. These programming basics make learning advanced programming languages painless.
This article discusses why you should learn C before other languages. It also explains the basics of the language to get you started with C programming.
Why Learn C Language?
Learning C is foundational for programmers to learn advanced programming languages. Why? Because:
- It helps you understand the underlying architecture of operating systems.
- Other languages have the same concepts and features as C. These similar programming basics help you learn other languages, such as Java and C++.
- You can find resources and courses online to learn C.
- Beginner programmers can get support from C programming experts via communities and forums.
- You can get started with C language using a web-based C Compiler. You can write and compile codes in your browser without installing any programming software.
Besides these learning benefits, the C language also offers the following primary advantages.
- It is easier to test and debug programs written in C because of its module-based structure.
- Due to its universal nature, C can interface with other languages.
- You can contribute to open-source projects.
- C is a low-level programming language. It offers low-level access to memory and efficient use of the processor and memory.
- C language offers fast execution and high performance as it can directly communicate with the hardware.
- Applications and technologies such as the Internet of Things (IoT), operating systems, application development, embedded systems, and building databases mostly run on C.
Thus, people who first learn C can grasp modern programming languages.
How to Get Started With C Programming Language?
You’ll need to set up your system to write and execute your code in C. There are two ways to achieve this purpose.
- A Text Editor and Compiler
A classical way to build and execute C programs is to install a text editor and a compiler on your system. A text editor lets you write and edit your code; you need a compiler to run and execute the program. Sublime Text, Notepad++, and Visual Studio Code are some of the popular text editors.
There are different compilers available according to your operating system. The most popular ones are Borland Turbo C, Tiny C Compiler, and Portable C Compiler.
- An Integrated Development Environment
A more robust tool to get started with C programming setup is an IDE. Feature-rich software contains a source code editor, debugger, and compiler. The programmer can write, edit, and execute the code in a single program.
Visual Studio, Codeblocks, AWS Cloud9, Eclipse, and Zend Studio are some of the most common IDEs. Web-based IDEs are also available with limited functionality. Yet, these are good enough to start learning the C language.
Learning the Fundamentals of C Programming Language
C is a static language, meaning the type of a variable is known at compile time. In other words, the types of each variable must be known before executing the source code. Let’s look at some of the core concepts of C language, such as data types, functions, conditionals, etc.
Data Type in C Language
C uses variables to store data values. Different types of variables are available in C. To create a variable, you need to specify its data type.
Syntax
type VariableName = value;
There are five major categories of C data types; primary, derived, enumerated, void, and bool. The following are the basic data types used in the C language:
- Primary Data Types
Primary data types or primitive data types store arithmetic data values. A primary data type tells the size and type of information a variable stores. These are further classified into the following data types.
- Integer – Integer data types store whole numbers such as 8, 90, 874, and 1322. The “int” keyword refers to integer data type.
- Floating Point – Floating point data type stores all the decimal values (real numbers). The ‘’float” keyword refers to the floating point data type.
- Double – Double data type stores data values up to 10 digits after the decimal. It refers to large numeric values that are not stored in integer and floating point data types. “double” keyword refers to the floating point data types.
- Character – Character stores letters of the ASCII chart and single alphabets such as ‘a’, ‘b’ , ‘c’ etc. The “char” keyword refers to the character data type.
- Derived Data Types
These are primary data types with added functionalities. They represent multiple values in a program. Following are the types of derived data types.
- Array – Arrays are an ordered and finite sequence of data having the same data type under a single name. Square brackets “[]” create an array.
- Pointer – Pointers are variables that hold the memory addresses of other variables. The “*” operator creates a pointer that refers to data types of the same kind.
- Structure – Structures are collections of data of different data types. “struct” keyword creates a structure followed by its name.
- Function – A function is a set of statements contained in a code block. Functions help in using the same set of statements many times in a code. Once defined, they run upon call. “main()” and “printf()” are examples of predefined functions.
Functions in C Language
As mentioned above, functions are a block of code that runs upon call. A function must be declared to tell the compiler its parameters and return type. You can call a function anywhere in the program.
Defining a function refers to the actual statements a compiler executes upon a function call. Upon execution, a function returns a value in your specified type. Below is the syntax to create a function.
Syntax
void FunctionName() { // code to be executed }
There are two types of functions in C.
- Standard Library Functions
These are built-in functions in C programming and are defined in the header files. printf(), scanf(), gets(), puts(), ceil(), floor() are all examples of library functions.
- User-defined Functions
Users can create functions as per their needs, known as user-defined functions. They are generally used to divide large codes into small parts for ease of understanding.
Conditionals – If Statements in C Language
C language offers conditional statements that make decisions based on the conditions. C has two conditional statements as follows;
- If statements – The specified condition is executed first. If the condition is true the code block in the body gets executed.
Syntax
if (condition) instruction;
- If-else statements – It is an extended form of an If statement. In this case, when a test exception is executed, if the condition is true, it executes the true block of the program. Otherwise, the false set of statements executes.
Syntax
if (condition) { True set of statements } Else { False set of statements } Statements;
Operators – To make these decisions, there are six relational operators available in C.
- Less than: a < b
- Less than or equal to: a <= b
- Greater than: a <= b
- Greater than or equal to: a >= b
- Equal to a == b
- Not Equal to: a != b
Loops in C Language
Loops allow execution of the same set of statements many times. The repetition of instructions continues until the specified condition is met. Loops have two parts: a control statement and a body. The initialization statement executes once. Next, the test statement executes. If it evaluates as false, the loop terminates otherwise statements inside the body are executed. After this test statement updates and executes again. There are three types of loops in C:
- for loop
- while loop
- do…while loop
Syntax of for Loop
for (initialization statement; test statement; update statement) { // loop body // statements inside the loop body }
Execute Your First Program in C Language
Below is a basic program written in C that prints your name. It includes a library <stdio.h> to work with the input and output function printf(). main() is the function that executes whatever you write inside the {} curly brackets. printf() is another function used for output. It displays the text on the screen, in our case ‘‘Sololearn’’.
Program to Display Your Name
#include <stdio.h> int main() { printf("Sololearn"); return 0; }
Output
Sololearn
Conclusion – How to Learn C With Sololearn
Climbing and running compliments a mountaineer in training for a summit. In the same way, learning C complements a programmer in mastering advanced programming languages.
The C language basics covered in this article set the stage for beginners to start their programming careers. ‘Introduction to C’ (a beginner-level course on C language) is a great resource by Sololearn.
If you are looking to dive deeper into the C language, explore ‘C Intermediate. It’s an intermediate-level course on C. With Sololearn’s global community and courses designed by experts, you will never feel overwhelmed in your C learning journey.