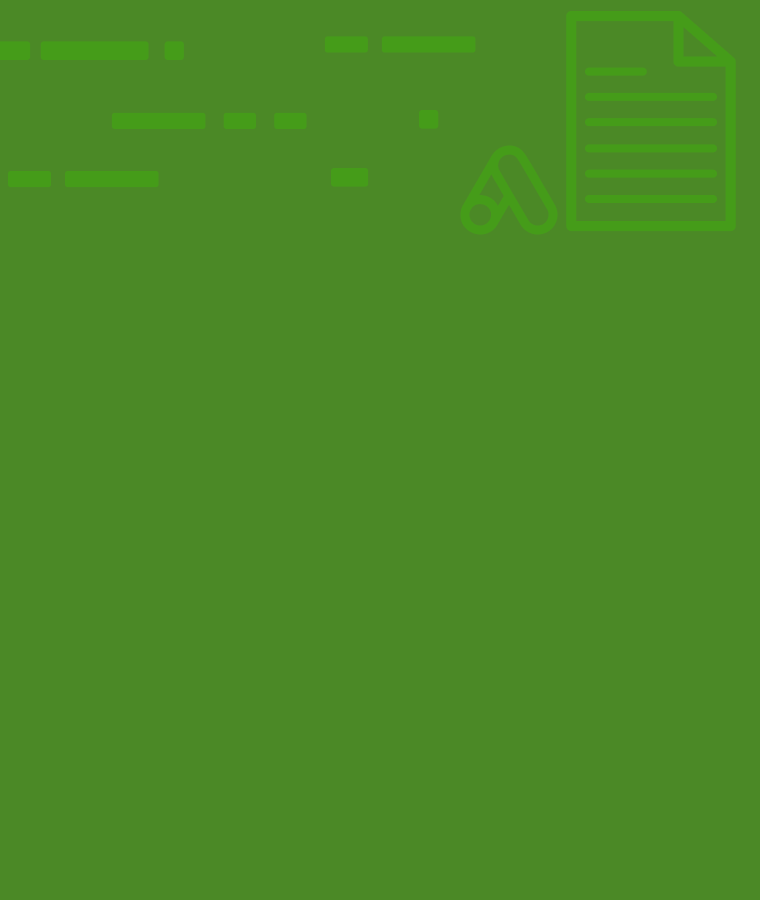
How to improve your Google Ads campaigns using scripts
As a marketer or paid media specialist, you know the importance of efficiently managing your Google Ads campaigns. But manually handling every aspect of your campaigns can be time-consuming and tedious. That’s where scripts come in.
Scripts are like your personal assistant for Google Ads. They can automate tasks, generate reports, and even make changes to your campaigns based on data. Think of it like having a robot do your chores, but instead of cleaning your house, it’s optimizing your ads.
In this post, we’ll cover what scripts are, why JavaScript is important for creating scripts, and how to create your own scripts for Google Ads. We’ll also provide examples of scripts for different tasks and tips for customizing them for your specific needs.
So let’s dive in and learn how to make your Google Ads campaigns more efficient with the power of scripts.
What are scripts in Google Ads?
Scripts are snippets of code that you can write and run within your Google Ads account. They use JavaScript, a popular programming language, to interact with your account data and perform actions based on your instructions.
Scripts can help you automate tasks that would otherwise require manual work or external tools. For example, you can use scripts to:
- Pause or enable campaigns, ad groups, or keywords based on performance or budget
- Adjust bids or budgets based on external factors, such as weather, stock, or competitors
- Generate custom reports or alerts based on your metrics and goals
- Apply labels or rules to your campaigns, ad groups, or keywords based on criteria
- And much more!
Scripts can save you time, money, and hassle by taking care of the repetitive or complex tasks that you don’t want to do yourself. They can also help you optimize your campaigns and improve your results by making data-driven decisions.
Sounds awesome, right? But how do you write scripts for Google Ads? And what do you need to know about JavaScript? Don’t worry, we’ll cover that in the next sections. But first, let’s do a quick quiz to check your understanding of scripts.
But… What is exactly JavaScript?
We’ve been talking about scripts for a while now, but where does the concept of “script” come from? The answer is JavaScript, a popular programming language that is used to create scripts for Google Ads.
JavaScript is a lightweight, interpreted, object-oriented language that is commonly used to create dynamic and interactive web pages. It is also used to create scripts for Google Ads, which can interact with your account data and perform actions based on your instructions.
JavaScript is important for creating scripts for Google Ads because it allows you to automate tasks, generate reports, and make changes to your campaigns based on data. Without JavaScript, you would have to manually handle every aspect of your campaigns, which can be time-consuming and tedious.
In addition to being important for creating scripts, JavaScript is also a versatile and powerful language that is used in a wide range of applications. It is one of the core technologies of the web, along with HTML and CSS, and is supported by all major web browsers.
While you don’t need to be an expert in JavaScript to create scripts for Google Ads, it is helpful to have a basic understanding of the language. Some of the basic concepts of JavaScript include:
- Variables: used to store data
- Functions: used to perform specific tasks
- Loops: used to repeat a set of instructions
- Conditional statements: used to make decisions based on conditions
- Arrays: used to store multiple values in a single variable
- Objects: used to store data and functions in a structured way
If you want to learn more about the basic concepts of JavaScript, you can also take a look at the article X basic concepts of JavaScript for beginners.
How to create scripts into Google Ads?
Now that you know what scripts are and why they are useful for Google Ads, let’s dive into the step-by-step process of creating scripts. (Note: We will add screenshots to illustrate each step.)
Step 1: Access the Scripts Page
To create a script, first log in to your Google Ads account and navigate to the “Scripts” page. You can find it under the “Bulk Actions” menu on the left-hand side of the page.
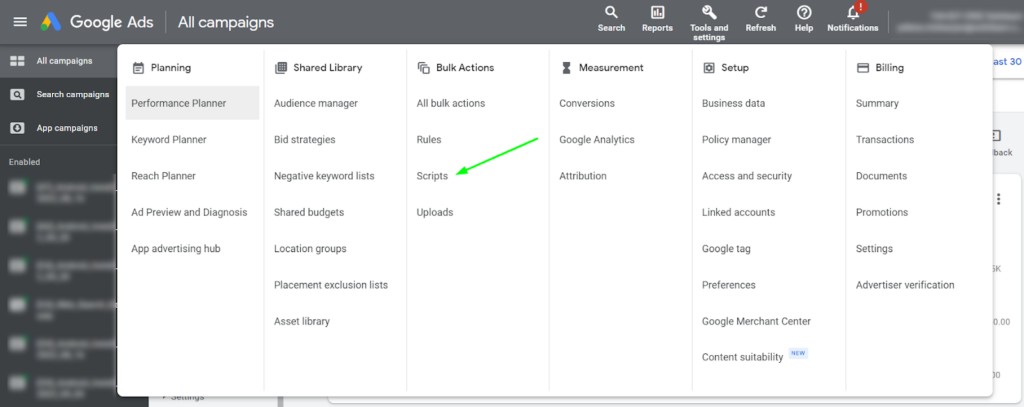
Step 2: Create a New Script
On the Scripts page, click on the “+ New Script” button to create a new script. This will open a blank script editor where you can write your code.
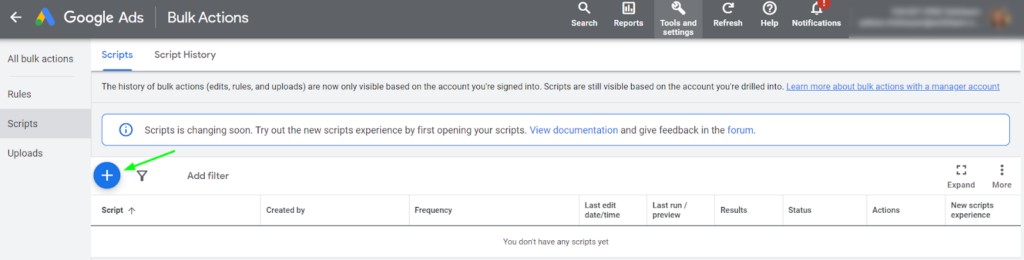
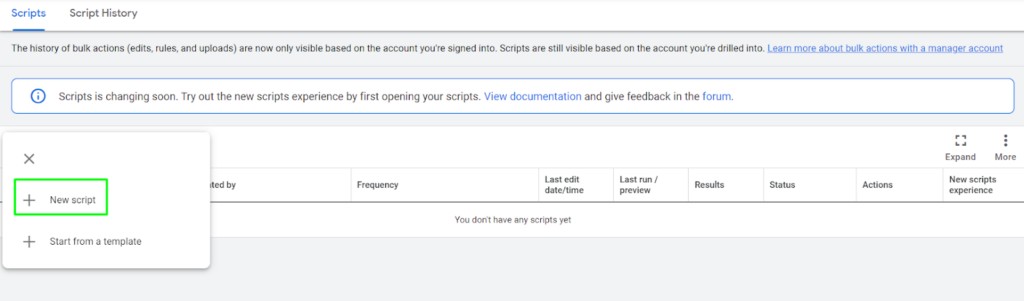
Step 3: Write Your Script
In the script editor, write your script using JavaScript. You can use the basic concepts of JavaScript, such as variables, functions, loops, and conditional statements, to interact with your account data and perform actions based on your instructions.
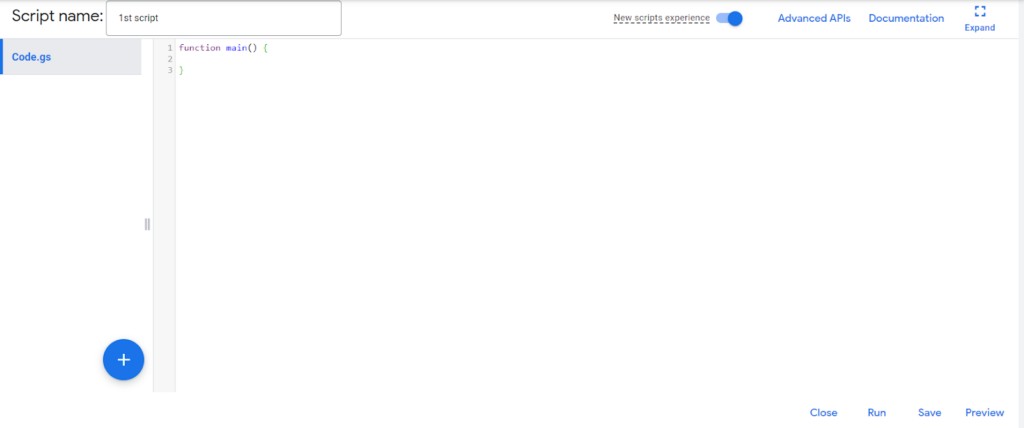
Step 4: Preview and Test Your Script
Before you run your script, it’s important to preview and test it to make sure it works as intended. Click on the “Preview” button to see how your script will interact with your account data. You can also use the “Logs” tab to check for any errors or issues with your script.
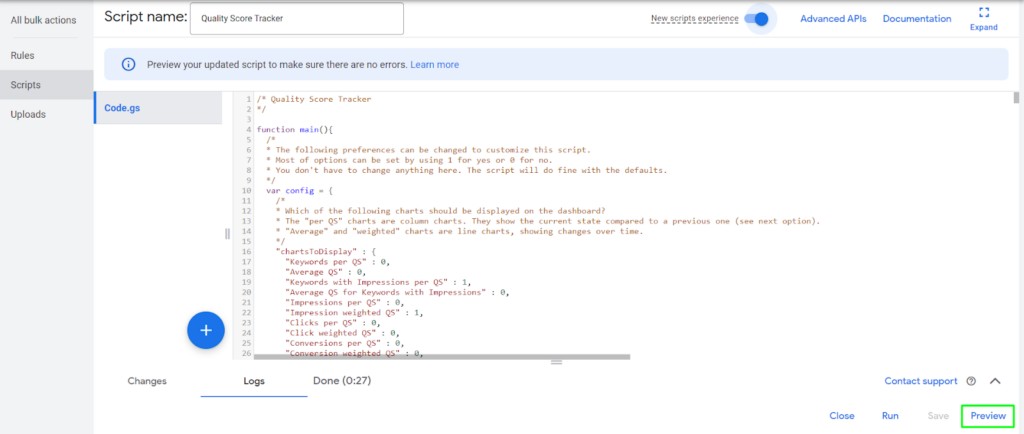

Step 5: Save and Run Your Script
Once you are satisfied with your script, click on the “Save” button to save your changes. Then, click on the “Run” button to run your script and apply the changes to your account.
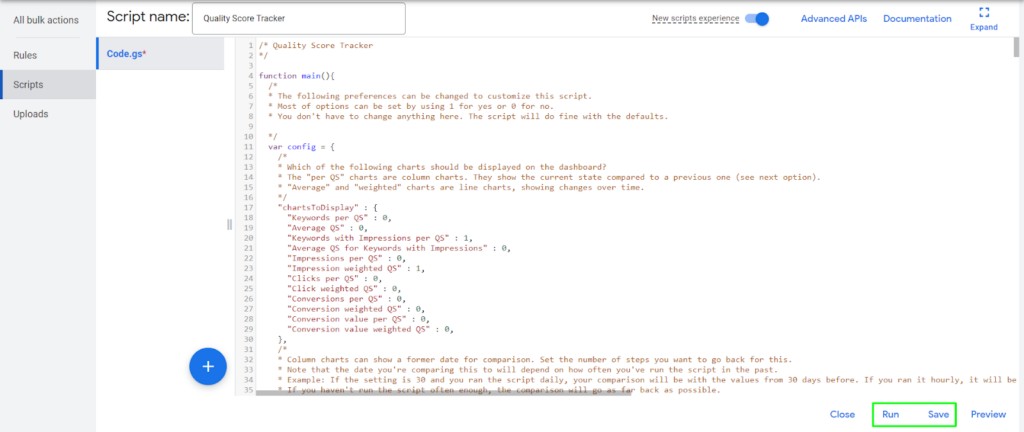
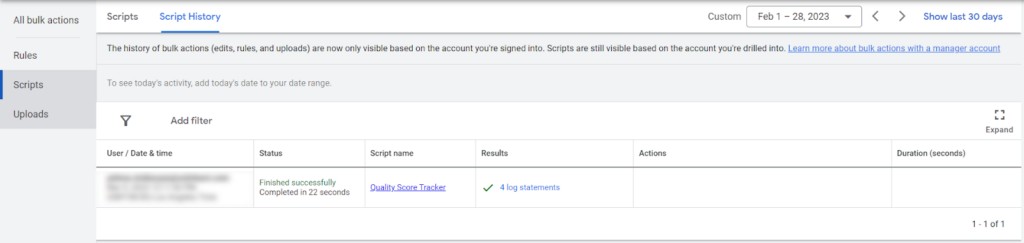
Tips and best practices for writing effective scripts in Google Ads
As you start creating your own scripts for Google Ads, it’s important to keep in mind some best practices that can help you create effective and efficient scripts. These best practices can help you stay organized, automate your scripts, and monitor their performance. Here are some of the key best practices to keep in mind:
- Start with a clear goal in mind: Know what you want to achieve with your script and write your code accordingly.
- Keep it simple: Avoid overcomplicating your script with unnecessary code or features.
- Use comments: Add comments to your code to explain what each section does and make it easier to understand.
- Test and debug: Always preview and test your script before running it to avoid errors or unexpected results.
- Use a naming convention: Give your script a descriptive and consistent name to make it easier to find and manage.
- Schedule your script: Set up a schedule for your script to run automatically at regular intervals.
- Monitor your script: Keep an eye on your script’s performance and make adjustments as needed.
Some useful Google Ads scripts for your campaigns
In this section, we will explore three examples of Google Ads scripts that can be useful for marketers. These scripts address potential needs of a marketer and provide a starting point for developing customized scripts that meet specific business goals.
While these scripts demonstrate some of the capabilities of Google Ads scripting, there are many other ways that scripts can be used to automate and optimize advertising campaigns. If you’re interested in creating your own scripts, it’s recommended to start with an Introduction to JavaScript course or a Javascript Intermediate course if you already know the basics.
Ad Performance Report Script
function main() { var report = AdsApp.report( 'SELECT CampaignName, AdGroupName, Headline, Impressions, Clicks, Conversions ' + 'FROM AD_PERFORMANCE_REPORT ' + 'WHERE Impressions > 0 ' + 'DURING LAST_7_DAYS'); var rows = report.rows(); while (rows.hasNext()) { var row = rows.next(); Logger.log(row['CampaignName'] + ' > ' + row['AdGroupName'] + ' > ' + row['Headline'] + ': ' + row['Impressions'] + ' impressions, ' + row['Clicks'] + ' clicks, ' + row['Conversions'] + ' conversions'); } }
What are we doing exactly in that snippet?
- The script retrieves data from the Ads platform related to the performance of ads.
- It selects data for the last 7 days and only for ads that have impressions.
- The data selected includes the name of the campaign, the name of the ad group, the headline of the ad, the number of impressions, clicks, and conversions.
- The retrieved data is logged using the Logger service, which creates a record that can be viewed later.
- The log includes the campaign name, ad group name, ad headline, number of impressions, clicks, and conversions.
Keyword Performance Monitor Script
function main() { var keywords = ['keyword1', 'keyword2', 'keyword3']; var threshold = 1.5; var email = 'example@example.com'; var report = AdsApp.report( 'SELECT CampaignName, AdGroupName, Criteria, Impressions, Clicks, Ctr, CostPerClick ' + 'FROM KEYWORDS_PERFORMANCE_REPORT ' + 'WHERE Criteria IN ["' + keywords.join('", "') + '"] ' + 'DURING LAST_7_DAYS'); var rows = report.rows(); while (rows.hasNext()) { var row = rows.next(); if (row['Ctr'] < threshold) { sendEmailAlert(email, row['CampaignName'], row['AdGroupName'], row['Criteria'], row['Ctr']); } } } function sendEmailAlert(email, campaign, adgroup, keyword, ctr) { var subject = 'Low CTR for keyword ' + keyword; var body = 'The keyword "' + keyword + '" in campaign "' + campaign + '", ad group "' + adgroup + '" has a CTR of ' + ctr + '.'; MailApp.sendEmail(email, subject, body); }
What are we doing exactly in that snippet?
- We are fetching performance data for a list of keywords from the KEYWORDS_PERFORMANCE_REPORT report for the past 7 days.
- We are checking if the click-through rate (CTR) for each keyword is less than a given threshold value (1.5% in this case).
- If the CTR is less than the threshold, we are sending an email alert to a specified email address with details about the keyword’s performance, including its campaign name, ad group name, and current CTR.
- We are using the MailApp service to send the email alert.
Ad Schedule Adjuster Script
function main() { var campaignIterator = AdsApp.campaigns().get(); while (campaignIterator.hasNext()) { var campaign = campaignIterator.next(); var report = campaign.targeting().hourOfDay().get(); var bidModifierList = []; while (report.hasNext()) { var row = report.next(); var hourOfDay = row.getTimeOfDay(); var conversions = row.getConversions(); if (conversions > 0) { var conversionRate = row.getConversionRate(); if (conversionRate > 1) { bidModifierList.push({ x: hourOfDay, y: 1.2 }); } else { bidModifierList.push({ x: hourOfDay, y: 0.8 }); } } } campaign.targeting().setBidModifier( "HOUR_OF_DAY", bidModifierList ); } }
What are we doing exactly in that snippet?
- We are iterating through all campaigns in the account and fetching a report of hourly performance for each campaign.
- For each hour of the day, we are checking if the conversion rate is greater than 0.1%.
- If the conversion rate is greater than 0.1%, we increase the bid modifier for that hour to 1.2, which will increase the bid for that hour by 20%.
- If the conversion rate is less than or equal to 0.1%, we decrease the bid modifier for that hour to 0.8, which will decrease the bid for that hour by 20%.
- Finally, we set the bid modifiers for the HOUR_OF_DAY targeting criterion for the campaign.