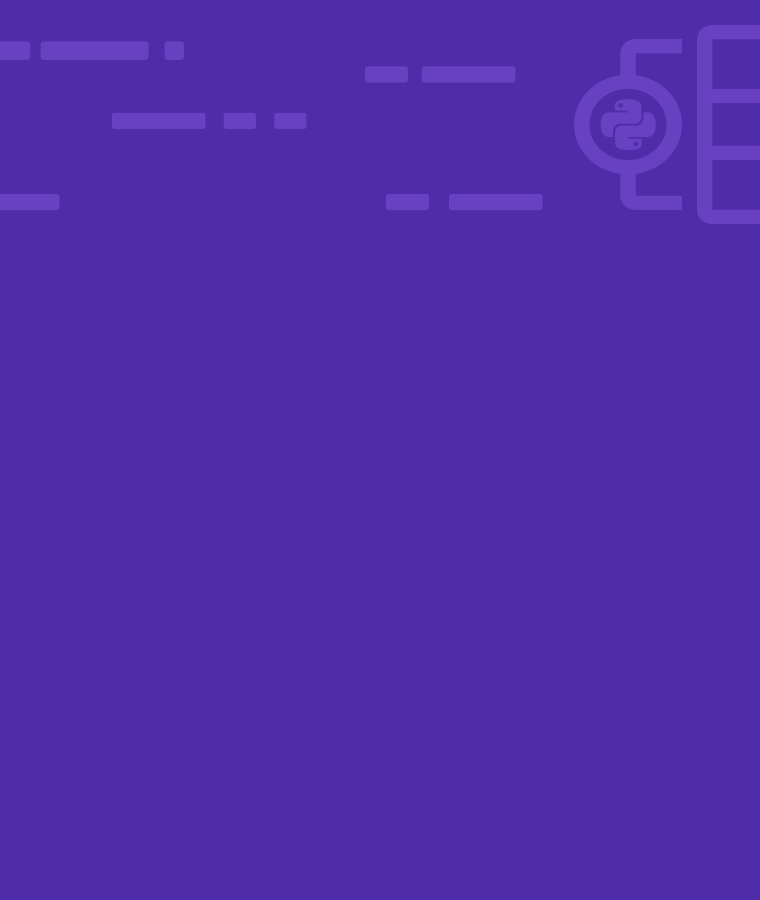
In-depth Guide to Python Data Types
Introduction
A python variable is a container that stores data whose value can be retrieved or manipulated when needed. The data stored can be of different types. Those types can be letters, numbers, symbols and so on. Python data types are the forms data can be stored in a variable. The main data types are:
- Numbers
- Strings
- Boolean
- Lists
- Tuples
- Sets
- Dictionaries
Numbers: these are numeric entries. They can be integers, floating numbers, and complex numbers. Integers are whole numbers with no decimal values; floating numbers are numbers with decimal points “.” Complex numbers are numbers with two parts; real and imaginary numbers.
Examples
sam_age = 15 # Integer print(sam_age) # >> 15 # stores a whole number "15" let's say the age of Sam; the result is 15 # =================================================================== average_score = 15.68 # Floating number print(average_score) # >> 15.68 # stores a decimal number "15.68". The result is 15.68. # =================================================================== number = 4 + 3j # complex number print(number) # >> (4+3j) # stores a complex number. The result is 4 + 3j.
Type notation
We use the type function to check if a number is an integer or a float.
Examples
sam_age = 15 print(type(sam_age)) # >> <class 'int'>
- In the above code, I put the variable name “sam_age” in the type method, type(sam_age) and printed the result.
- <int> means integer, the value stored in the variable name “sam_age” is a whole number.
average_score = 15.68 print(type(average_score)) # >> <class 'float'>
- In the above code, I put the variable name “average_score” in the type method, type(average_score) and printed the result.
- <float> means floating number; the value stored in the variable name “average_score” is a decimal number.
number = 4 + 3j print(type(number)) # >> <class 'complex'>
- In the above code, I put the variable name “number” in the type method, type(number) and printed the result.
- <complex> means complex number; the value stored in the variable name “number” is a complex number. It has two parts: the whole number and the number with “j”.
Things to note
- A floating number has a decimal point [.]
- When an integer(a whole number) is divided by another integer(a whole number), the result is a floating number.
Example
total_eggs = 12 num_of_people_toshare = 6 num_one_person_gets = total_eggs / num_of_people_toshare print(num_one_person_gets) # >> 2.0
- In the above code, I created three variable names, the first “total_eggs” stores the number of eggs, the second “num_of_people_toshare” stores the number of people to share the eggs and the last “num_one_person_gets” stores the amount each person gets.
- The result is <2.0>, a floating number has a decimal point, and the value is a floating number. We can also check the <type> to see if it is an integer or float.
print(type(num_one_person_gets)) # >> <class 'float'>
The type is <float>
Strings: are values wrapped in quotation marks. They begin with a quotation mark(“) and end with a quotation mark (“). The quotation marks can be single(‘ ‘), double(“ “) or triple(‘’’ ‘”)The values can be numbers(“28”), letters(“bcfh”), symbols(“@#${}[]”) or everything together(“234hdfijfi*&&&$][\”).
Examples
# Create a variable name, then put the text in single or double quotes; 'text' or "text", then print out the variable name name = "Sofia" # a word print(name) # >> Sofia # ======================================================================= sentence = "I love programming a lot" # a sentence print(sentence) # >> I love programming a lot # ======================================================================= age = "14" # integer print(age) >> 14 rating = 10.00 # floating number print(rating) # >> 10.00 # ======================================================================= special_symbols = "!@#$%^&*()_+}{[]" # symbols print(special_symbols) # >> !@#$%^&*()_+}{[] # ======================================================================= # number and symbol print("1 + 1") # >> 1 + 1 print("2 * 3") # >> 2 * 3 print("8 = 4") # >> 8 = 4
The numbers are not added together or multiplied in the above code because the expression starts and ends with a quotation mark. Exactly how the values are in the quotation marks is how it is printed in the console.
email_address = "johndoe23456@gmail.com" # all characters together print(email_address) # >> johndoe23456@gmail.com print("]um@]i i8 @\/\/es0/\/\e") # >> ]um@]i i8 @\/\/es0/\/\e
We can join strings and words to form a sentence using the addition sign (+); this process is known as concatenation.
Example:
name = "Sofia" age = "14" print(name + " is " + age + " years old") # >> Sofia is 14 years old
- In the above code, the number has quotation marks around it; therefore, it is a string. We only add strings.
Let’s try with an integer and see the result.
name = "Sofia" age = 14 print(name + " is " + age + " years old") # >> print(name + " is " + age + " years old") # TypeError: can only concatenate str (not "int") to str
The above result shows that strings cannot be added to integers(whole numbers).
Type notation
We can check what type a variable or a statement is; any item wrapped in quotation marks has the type <str> meaning string.
name = "Sofia" print(type(name)) # >> <class 'str'> age = "14" print(type(age)) # >> <class 'str'>
Boolean: expressions with the keyword “True” or “False”. It is used to check if a statement is right or wrong.
Examples
num_boys_greater_num_girls = False print(num_boys_greater_num_girls) # >> False dogs_are_friendly = True print(dogs_are_friendly) # >> True odd_number = 3 even_number = 2 print(odd_number > even_number) # >> True total_score = 100 student_score = 20 * 3 print(total_score < student_score) # >> False
Type Notation
A boolean has a type notation of <bool>, it has statements with either (True) or (False). The format is : type(variable_name) or type(statement).
num_boys_greater_num_girls = False print(type(num_boys_greater_num_girls)) # >> <class 'bool'> # In the above code block, a variable stores the value (False) and using the type method, it is <bool> meaning boolean. # ======================================================================= total_score = 100 student_score = 20 * 3 print(total_score < student_score) # >> False print(type(total_score < student_score)) # >> <class 'bool'> # 100 is greater than 60, so the above statement shows that it is (False), checking the type; it is <bool> meaning boolean.
Lists: consists of elements(they can be strings, numbers, boolean, list) in a square bracket and are separated by commas. A list starts with a square bracket “[“ and ends with a square bracket “]”.
Format
variable_name = [string1, string2, string3] variable_name = [number1, number2, number3] variable_name = [boolean1, boolean2, boolean3] variable_name = [string1, number1, string2, number2, boolean1, boolean2] variable_name = [[number1, string1], [number2, string2]]
Examples
student_names = ["Jenny", "Bless", "Gab"] # with strings print(student_names) # >> ['Jenny', 'Bless', 'Gab'] # ======================================================================= student_ages = [15, 17, 13] # with integers print(student_ages) # >> [15, 17, 13] # ======================================================================= student_scores = [10.40, 20.00, 16.04] # with floating numbers print(student_scores) # >> [10.4, 20.0, 16.04] # ======================================================================= student_responses = [True, False, False] # with boolean print(student_responses) # >> [True, False, False] # ======================================================================= number_of_colors_present = ["Red", 4, "Yellow", 3, "Green", 5] print(number_of_colors_present) # >> ['Red', 4 , 'Yellow', 3, 'Green', 5] student_result = [ ["Maths", 10.40], ["English", 20.00], ["Physics", 16.04], ] # with lists print(student_result) # >> ['Maths', 10.4], ['English', 20.0], ['Physics', 16.04]]
Type Notation
A list has a type notation of <list>, it has statements with square brackets “[]”. The format is : type(variable_name) or type(statement).
# type(statement) print(type(["Bingo", "14 years", "1.54meters", "Orange fur"])) # >> <class 'list'> # type(variable_name) late_comers = [["Shane", 10.40], ["Fab", 10.30]] print(type(late_comers)) # >> <class 'list'>
Tuples: consist of elements(like strings and numbers, boolean ) stored in brackets and separated by commas. They start with a bracket “(” and end with a closing bracket “)”.
Format
variable_name = (string1, string2, string3) variable_name = (number1, number2, number3) variable_name = (boolean1, boolean2, boolean3) variable_name = (number1, string1, number2, string2, boolean1) variable_name = ((number1, string1), (number2, string2), (boolean1, string3))
Examples
student_names = ("Jenny", "Bless", "Gab") # with strings print(student_names) # >> ('Jenny', 'Bless', 'Gab') # ======================================================================= student_ages = (15, 17, 13) # with integers print(student_ages) # >> (15, 17, 13) # ======================================================================= student_scores = (10.40, 20.00, 16.04) # with floating numbers print(student_scores) # >> (10.4, 20.0, 16.04) # ======================================================================= tuts = ((12, 12), (13, 14), (14, 14)) print(tuts) # ((12, 12), (13, 14), (14, 14)) student_info = ("Jane", 13, "Blue eyes", "Brown pet", 1.83, True, 2, 14.8) # with strings, integers, floating numbers and boolean print(student_info) # >> ('Jane', 13, 'Blue eyes', 'Brown pet', 1.83, True,2, 14.8)
Type Notation
Tuples have the type notation <tuple>, and their statements are wrapped in brackets “()”.
student_info = ("Jane", 13, "Blue eyes", "Brown pet", 1.83, True, 2, 14.8) print(type(student_info)) # >> <class 'tuple'> print(type((10, 20, 14.50, 20.10, 15))) # >> <class 'tuple'>
Sets: consist of elements(such as numbers, strings, tuples, booleans,lists) stored in curly braces ‘{}” and separated by commas. They start with a curly brace “{“ and end with the other “}”.
Format
variable_name = {string1, string2, string3} variable_name = {number1, number2, number3} variable_name = {string1, number1, boolean1, tuple1, string2, number2, list1, boolean2}
Examples
colors = {"red", "green", "yellow"} print(colors) # >> {'red', 'yellow', 'green'} temperature_in_celsius = {27, 30.6, 19} print(temperature_in_celsius) # >> {27, 19, 30.6} any_data = {"Peter Parker", ("Black spiderman", "Red spiderman"), False, 30.01, 15} print( any_data ) # >> {False, 'Peter Parker', ('Black spiderman', 'Red spiderman'), 30.01, 15}
- When sets are printed out, the result does not show the items in order they are stored in the variable
Type Notation
Sets have the type notation <sett>, and their statements are wrapped in curly braces “{}”.
any_data = {"Peter Parker", ("Black spiderman", "Red spiderman"), False, 30.01, 15} print(type(any_data)) # >> <class 'set'>
Dictionaries: a collection of items wrapped around by curly braces “{}”. Those items are referred to as key and value pairs. These key-value pairs can also be strings, numbers, lists, boolean, tuples and dictionaries.
Format
variable _name = {string1: string2, string3: string4} variable_name = {string1: number1, string2: number2 } variable_name = {string1: boolean1, string2: number1, string3: list1, string4: tuple1, string5: dictionary1}
Examples
student_data = {"001": "Singh", "002": "Alice"} # with strings print(student_data) # >> {'001': 'Singh', '002': 'Alice'} # ======================================================================= student_age = {"Bob": 12, "Jack": 14, "Milly": 16} # with strings and integers print(student_age) # >> {'Bob': 12, 'Jack': 14, 'Milly': 16} # ======================================================================= favorite_book = { "Title": "Storm and Silence", "Completed": True, "Number of Pages": 466, "Favorite Characters": ["Lily Lilton", "Captain Jack", "Rikkard Ambrose"], "Rating": 5.0, "Sequels": 3, "Years of each sequel": (2016, 2017, 2019), } print(favorite_book) # >> {'Title': 'Storm and Silence', 'Completed': True, 'Number of Pages': 466, 'Favorite Characters': ['Lily Lilton', 'Captain Jack', 'Rikkard Ambrose'], 'Rating': 5.0, 'Sequels': 3, 'Years of each sequel': (2016, 2017, 2019)}
Type Notation
Dictionaries have the type notation <dict>, and their statements have key-value pairs wrapped in curly braces “{}”.
student_age = {"Bob": 12, "Jack": 14, "Milly": 16} print(type(student_age)) # >> <class 'dict'>
Data type comparison
Strings and Numbers
When numbers are wrapped in quotation marks, they are referred to as strings and cannot perform mathematical operations because they function like text in the console.
Example
I – Addition
Strings
print("1" + "1") # >> 11 print("John" + "Wick") # >> JohnWick
When an addition sign (+) is in between two strings or more, they are brought together.
Numbers
print(1 + 1) # >> 2 print(5.06 + 4.98) # >> 10.04
- Adding up numbers gives us the sum.
II – Multiplication
- When strings are multiplied by a number, the output will be the string appearing as many times as the number multiplied by. In the code below, the string ‘abcd’ is multiplied by 5, and the result is ‘abcd’ appearing five times.
print("abcd" * 5) # >> abcdabcdabcdabcdabcd
The number <10> is wrapped in quotation marks, making it a string; the result is the value “10” appearing five times.
print("10" * 5) # >> 1010101010
In the code block below, <10> multiplies by 5, and the result is 50; the <10> is not wrapped in quotation marks; therefore, it is a number.
print(10 * 5) # >> 50 print(5.05 * 5) # >> 25.25
Strings cannot be subtracted or divided, but numbers perform those two operations.
Strings and Boolean
Statements that are wrapped in quotation marks (“”) are known as strings, while boolean has two statements; <True> or <False>.
“True” or “False” is not the same thing as True or False;
“True” or “False” is wrapped in quotation marks, which means it is a string, while <True> or <False> is not wrapped in quotation marks, meaning it is boolean.
response_one = "True" print(type(response_one)) # >> <class 'str'> response_two = True print(type(response_two)) # >> <class 'bool'>
Lists and tuples
Lists are denoted by square brackets “[]” and tuples are denoted by brackets “()”. They can both store strings,numbers and booleans.
t = ("Hello", 1, "love", "food", True, 12.3, "calories") l = ["Hello", 1, "love", "food", True, 12.3, "calories"] print(t) print(l)
Dictionaries and Sets
Dictionaries and sets have their elements wrapped in curly braces. The main difference is that in dictionaries there are keys and values while in sets justs the statements. Also sets do not store lists.
# sets anydata_one = {"red", 1.2, False, 40, ("Joker", "Hailey")} print(anydata_one) # >> False, 1.2, 40, ('Joker', 'Hailey'), 'red'} # ========================================================================================= # dictionaries anydata_two = { "sky_color": ["blue,white,grey"], "weather": ("stormy", "sunny", "rainy"), "Number of seasons": 4, "Snows all the time": False, } print( anydata_two ) # >> {'sky_color': ['blue,white,grey'], 'weather': ('stormy', 'sunny', 'rainy'), 'Number of seasons': 4, 'Snows all the time': False}
Conclusion
This was a lot to take in but getting to the bottom shows you made efforts to learn about the topic. Remember to take it one at a time and celebrate little wins. To know more about python data types, check here.