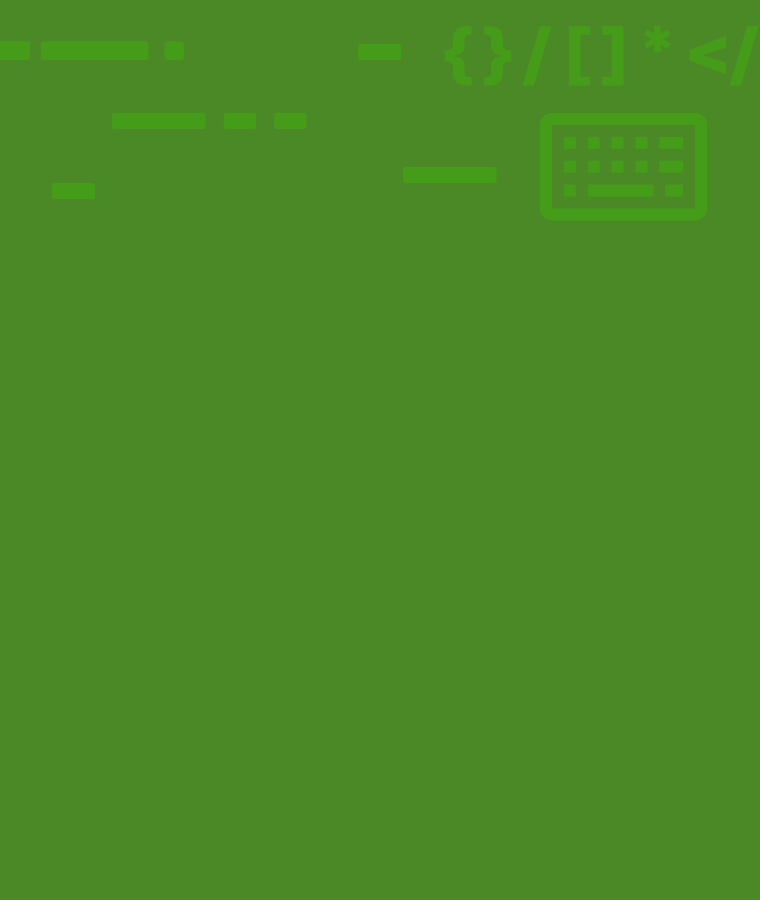
How Do You Write Your Own Programming Language?
Why would you ever write your own programming language? And how do you write your own programming language?
While it’s not the easiest thing in the world to do, if you’re an aspiring programmer, this might be something you might want to consider.
Why Is There A Need To Write Your Own Programming Language?
Making a new programming language’s interpreter is incredibly gratifying. It deepens your grasp of essential software concepts and how computers function. Although programming language interpreters might be confusing for new programmers, you can still write decent software without worrying about how the languages and the related rudimentary tools function.
Writing a programming language requires knowledge of the entire computing stack.. It revolves around applications of:
- Graph theory
- Category theory and type systems
- Complexity analysis
- More topics in computing mathematics
Writing and studying programming languages can help you learn more about computers’ mathematical and mechanical foundations. Writing your own programming language will make you a better developer, even if your daily work seldom involves language tools or compiler writing.
Even better, the language you create can grow as you’re growing as a developer. Even after the first version is “finalized,” a programming language can be expanded, optimized, and enhanced. With careful initial implementation, you might gradually add additional keywords, features, optimizations, and tools to your language, interpreter, or compiler. It’s simple to transform a programming language into a long-term creative project.
How To Write A Programming Language?
So, how do you create your own programming language? First, let’s start with what you need to consider when creating your own language, from design considerations to implementation and extension.
Design Considerations
Design a language first, then an interpreter or compiler. Meanwhile, the most significant thing you can do for yourself is study concepts from various programming languages. Don’t simply restrict yourself to modern languages such as Rust and Python; look back into computer history. Ideas from computing and mathematics may be recreated and integrated into novel ways.
This phase is spent defining syntax in most current OOP-like languages, including Python, Ruby, and Swift. Making a programming language in C++ is also included in the given list. But it would be best to keep in mind that it’s the designing of the language’s semantics that you need to focus on the most. It’s the stage where the “feel” of the language gets developed, and where you learn the most. Semantics are more complicated to modify than syntax.
Here are some questions to consider while considering language semantics and ergonomics:
- Which categories does your language belong to?
- Is it verified when the program is compiled?
- Does it do the necessary conversions between types on its own automatically?
- In your programming language, what is the highest-level organizing unit of programs?
- Modules, packages, or libraries are familiar names for this in programming languages, but other languages, like Rust, have developed their unique terminology for this notion.
- How does the programming language handle unusual circumstances, such as when a file is missing, when there is a problem accessing the network, or when there is a divide-by-zero error?
- Do you handle errors in the same manner as exceptions that propagate up the call stack, or do you see errors in the same way as values, as in C/Go?
- Does the language you’re using do optimization for tail recursion? Or do you have built-in control-flow tools for looping, such as while loops and for loops?
- How much functionality do you want to embed directly into the language, unlike providing it via a standardized library?
- Let us take an example of C, which does not have any knowledge of strings; instead, the C standard library is responsible for providing string semantics.
- The majority of high-level languages have maps and lists as core components of the language, but the majority of low-level languages do not.
- What are the mental processes behind memory allocation in your language?
- Memory is automatically allocated as required; is that correct?
- Is the developer going to have to create code in order to do this?
- Is there a rubbish collection service?
Implementation Considerations
The next step is to “test-drive” what you have designed for the operation and feel of a language. It determines how it will feel to use programs that have been created in that language.
Test-Drive Phase
During this step, you should save a text document in which you may experiment with the syntax of the new language by building tiny programs using that language. You may try to implement little algorithms and data structures such as recursive mathematical functions. Some of the functions are sorting algorithms and minor web servers by writing notes and questions for yourself in the comments section of the code. Don’t move on until most of the syntax and semantics queries have been answered.
Now it’s time to test your hypothesis about the fundamental concepts behind the language design. You may produce some sample code to confirm that the ideas work well together during this step. Suppose you have built a programming language based on functional programming and asynchronous and event-driven concurrency. Then sample code will work for you. If you’re wrong, you should revise your assumptions or experiment with language design. It better reflects your knowledge.
This part of the design process is called the straw-man design phase. It’s a simple way to see if the language you’ve developed in your head will work for the applications you want to write.
Interpreter/Compiler Phase
At their most fundamental level, interpreters and compilers are layers of minor modifications. They turn your source code, a string of text, into some form that your machine can execute. Building such a system requires picking practical intermediate formats for transformations to operate with. You may need to use similar formats for runtime data structures to encapsulate your language’s values and functions in an active program.
Honestly, every language might, in theory, be compiled or interpreted. However, in most cases, one of these two options makes more sense for a particular language than the other. Programmers with more experience will inform you that interpreting is typically the choice that provides greater flexibility, whereas compiling results in superior performance.
Extensions Of Your Project
Even after a first rollout, you may add more complicated features, concepts, and optimizations using a toy programming language. If you want to expand your project, consider this:
Type Systems With More Advanced Features
Studying type systems may arouse interest in category theory and adaptations of beautiful data structures with sophisticated type systems in languages like Haskell or Elm. Swift, TypeScript, or Rust may be an excellent place to start if you come from Java, JavaScript, or Python.
Optimizations
What are the best techniques for speeding up your interpreter or compiler? What exactly does it mean to be faster in parsing and compiling the code and producing faster code? There is no shortage of past knowledge and literature on compiler performance in the wild.
Adding C Foreign Function Interface (FFI)
Interoperability amongst your language and another, often C binaries, is provided via an FFI. C FFIs are a fantastic way to learn about low-level aspects of how programs are built into application programs and about the formats and code creation of executable files.
Just-In-Time Compilation
Just-in-time (JIT) compilers are a hybrid type of compiler that creates compiled machine code on the fly. E.g., some of the most popular and quickest language runtimes, such as Chrome’s V8 and LuaJIT for Lua, are neither vanilla interpreters nor complete compilers. JITs can often make better tradeoffs between efficiency and runtime dynamism in the language than simple compilers or interpreters. But this comes at the expense of increased complexity in the compiler.
External Tools For Writing Your Own Programming Language
A renowned parsing library known as Bison is one example of the many out-of-the-box solutions for programmers developing their language. Other examples include LLVM, which refers to the collection of compiler tools. To determine which tools are most suited for the language you have selected, you need to research and check some developer forums.
Be Smart, Creative, and Work Gradually
Does this sound like a lot of effort? Don’t give up on writing your own programming language! Especially if what you like about programming the most is the opportunity for creative expression.
Now, if you are a beginner, the most effective way to learn grow as a developer is to pick up the fundamentals of one or more programming languages. After that, you can work your way up to creating your own.
A good place to start is with our course on C++ or Java. Or better yet, take a look at our list of free courses and see what you’re most interested in!