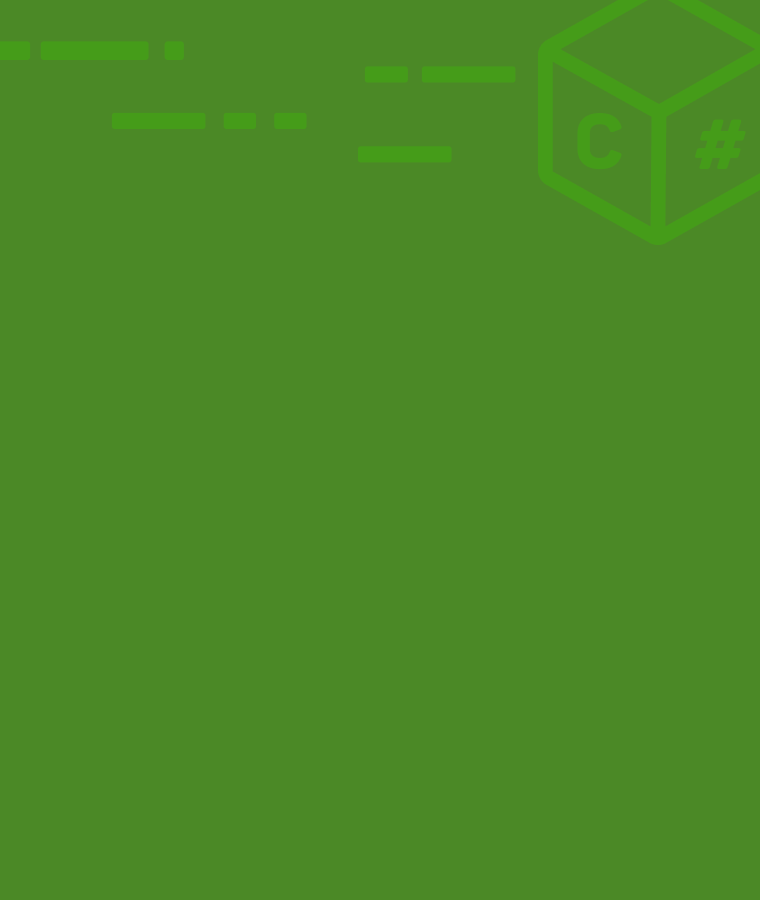
C# Array: What Are They & How to Use Them?
C# is amongst the top 5 programming languages according to the ‘Popularity of Programming Language index.’ You can find its use in diverse applications such as mobile applications, game development, and enterprise software.
C#, pronounced as ‘See Sharp’, is an object-oriented, general-purpose language. It runs on the most loved software development framework .NET, by Microsoft. Developers enjoy learning this multi-paradigm programming language. It is readable, fast, cross-platform, and focused on developer productivity. Moreover, it is high-in-demand with an active & vibrant community of developeC# arrayrs.
C# arrays are the next thing to learn after establishing C# language fundamentals related to data types, variables, and methods. This article explains the basics of C# arrays. And also discusses various array manipulation techniques.
What is Array in C#?
An array in C# is a type of data structure. It stores a sequential collection of elements with a fixed size.
An array is a collection of the same type of variables. Data items stored in an array are called array elements. Array stores these elements at contiguous memory locations. The number of elements in an array specifies its length. The first element corresponds to the lowest address. And the last element corresponds to the highest address of a particular array. C# arrays are different from C/C++ arrays.
How to Initialize Array in C#?
Array initialization is the process of assigning or storing values in an array. To initialize an array, you first need to declare it. You can declare an array by using an array name preceded by the data type and square bracket.
Syntax:
Data Type[] Arrayname
Once declared, you can initialize the array in the memory. The below-given statement declares and initializes an integer-type array. It can store ten integer values, as mentioned in the square bracket.
int[] Array = new int[10];
The following code snippet shows how to instantiate a C# integer array with predetermined values.
int[] Array = new int[4] { 23, 45, 78, 34 }
The statement given above initializes an array named “Array” with values 23, 45, 78, 34. The length of the array is 4.
Difference Between a List and Array
While both lists and arrays store variables; they are different. Lists in C# are a general collection of data items. Their memory is random and dynamic. It resizes whenever you add or remove elements from it. A list can contain heterogeneous data elements.
But, an array in C# always has a fixed size because array memory is static. It stores a fixed number of items of the same data types. Array memory is continuous and thus does not need memory tracking.
What is a C# String Array?
A string is a sequence of Unicode characters. And a string array is a collection of character arrays (in other words, an array of strings). You cannot change the string elements. C# string arrays can store only a fixed number of strings. It can be single and multidimensional.
You can specify the size of the array while declaring it or later. Use the following syntax to declare a string array in C# without specifying the size.
Syntax:
String[] < your variable name > // declaration of string array named “Arr” using keyword “string”string [] Arr;
To declare an array of strings with size, use the following syntax:
Syntax:
String / string[] < your variable name >= new String / string[provided size] // declaration of string array named “Arr” with size “3” using class object “String”String [] Arr = new String[3];
You can also declare and initialize an array with some strings in it, as shown below:
Syntax:
string[] Array_1, Array_2;
In the statement given above declares two string arrays named ‘Array_1’ and ‘Array_2’. You can also initialize these arrays using the following syntax:
Syntax:
Array_1 = new string[3] { "item1", "item2", "item3" }; Array_2 = new string[4] { "item1", "item2", "item3", "item4" };
Note: If you initialize an array without specifying its size, the code will give a compile time error. Thus, the size of an array is required while initializing it.
String[] Array = new String[]; //this will give errorString [] Array = new String[5]; //this is fine
What is int Array?
Integers are whole numbers that can be positive or negative. An int array is a collection of integer values only. Declaration and initialization of an integer-type array are similar to a string array. Below is the syntax for declaring an integer array:
Syntax:
Data Type[] < Array Name >
Below is an example to declare and initialize an int array.
// Declaration of array int[] numarr1, numarr2; // Initializing the array numarr1 = new int[3] { 2, 4, 6 }; numarr2 = new int[4] { -1, 25, -12, 100 };
How to Perform C# Array Manipulation?
Array manipulation is the act of performing various tasks on the array elements. You can add, remove, access, and transform the array elements using manipulation methods. Let’s explore some of the basic C# array manipulation techniques.
Access the Values of an Array
You can use the index value to access the items in the array. An index is the location reference of any array element. Array indexes start from 0. The square bracket contains the index number, followed by the array name.
Syntax:
<Array Name> [ <Index Number> ]
Example:
The following code snippet declares and initializes a string array with 4 elements named “colors”. The first element of the array is at index ‘0’.
string[] colors = { "Red", "Green", "Blue", "Gray" }; Console.WriteLine("==== Printing Strings in Array colors ===="); Console.WriteLine(colors[0]); Console.WriteLine(colors[1]); Console.WriteLine(colors[2]); Console.WriteLine(colors[3]);
To access different elements of the array pass the index value in the square bracket followed by the array name. Use index ‘0’ to read the first element of the array “Red.” Incrementing the index value will give the next item in the array.
The following output is obtained using the C# Compiler. C# Compiler is a user-friendly online compiler from Sololearn that allows users to write and run Java code on the web browser.
Output:
==== Printing Strings in Array colors ==== Red Green Blue Gray
Change an Array Element
The elements in the array are not immutable. In other words, you can change array items.
Example:
Below, we have declared an integer-type array with the name “num”. Suppose we want to change the value at the first and third index.
int[] num = { 2, 4, 6, 8 };
To change the first item, assign the value ‘-5’ to the array num at index ‘0’, as per the below statement.
num[0] = -5;
To change the third item, assign the product of two numbers to the array num at index ‘2’.
num[2] = 256 * 15;
Output the final array by printing the values on the console. The first and third array items will be modified.
Console.WriteLine("==== Printing Integers in Array num ===="); Console.WriteLine(num[0]); Console.WriteLine(num[1]); Console.WriteLine(num[2]); Console.WriteLine(num[3]);
Output:
==== Printing Integers in Array num ==== -5 4 3840 8
Print Out Array
Loops are used to print out a complete array. Loops perform iteration on an array from a starting index to the ending index.
Example:
int[] num = { 2, 4, 6, 8 }; for (int i = 0; i < num.Length; i++) { Console.Write($"| {vals[i]} |"); }
The variable “i” is initialized as 0 and indicates the index number. The variable “i” increments after each iteration. And the item at the index gets printed on the console. It will continue to increase to the highest value corresponding to the array length, which is 4 in our case.
We can achieve the same goal with a “foreach” loop as well, using the following code snippet.
foreach(var item in array) { Console.Write($"| {item} |"); }
Output:
| 2 || 4 || 6 || 8 |
Convert Array to String
You can convert an array of items to strings using the “string.Join” function. It concatenates the elements of an array using a parameter.
Example:
int[] num = { -13, 5, 255, -78 }; Console.WriteLine(string.Join(" === ", num));
Let’s consider an int array. The “string.Join” function will iterate through the array and concatenate the string using the “===” parameter.
Output:
-13 === 5 === 255 === -78
Check if an Element is in an Array
You can use the “Array.Exists” function to check if an item exists in the array or not, i. Let’s consider an example of a string array containing the fruit names – apple, banana, orange, and grapes.
Example:
string[] fruits = { "apple", "banana", "orange", "grapes" }; string searchElement = "orange"; bool exists = Array.Exists(fruits, element => element == searchElement); if (exists) { Console.WriteLine("Item found in array"); } else { Console.WriteLine("Item not found in array"); }
“Array.Exists” have a callback function that uses a temporary variable as a search element. In this case, the variable element is a parameter. It checks if the ‘searchElement’ is equal to the element in the fruit array or not.
If the values are equal, the function returns ‘true’; else, it returns ‘false.’
Output:
Item found in array
Conclusion – Become an Expert in C# with Sololearn
This article lays out the basics of C# arrays. It serves as a good starting point to learn about C# arrays and work with them using various manipulation techniques.
For programmers interested in mastering the C# language, Sololearn offers a detailed course, “Introduction to C#.” It is an excellent resource to build your basics and attain intermediate-level expertise in C#.
Moreover, with support from Sololearn’s global community and educational resources developed by experts, you can excel in your academics and programming career.