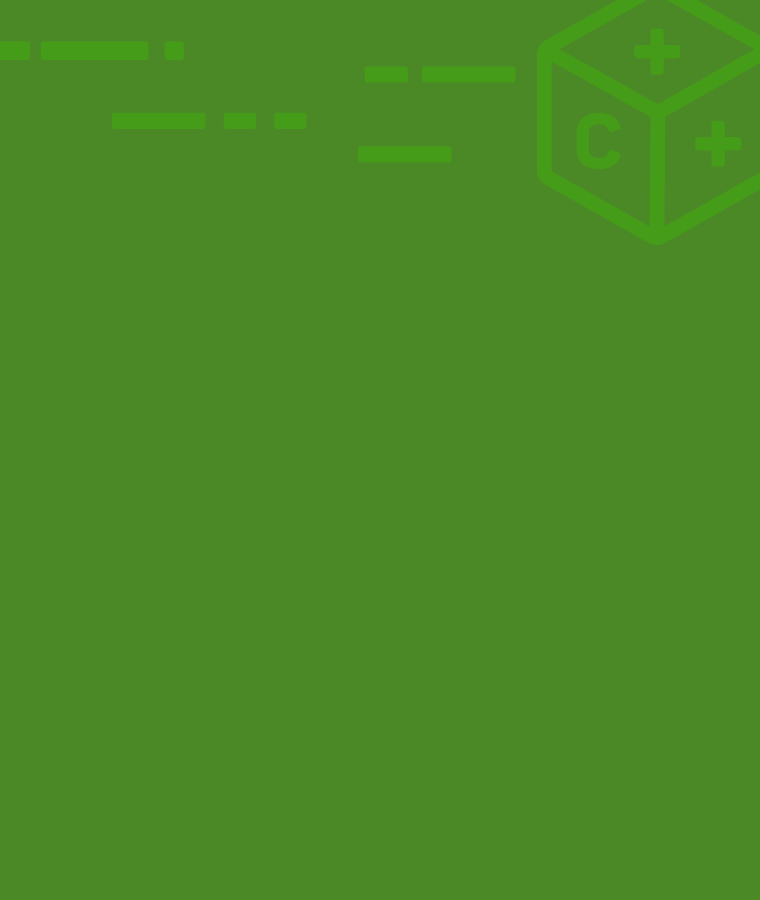
Introduction to C++ Array
Let’s understand with an example — suppose we want to store 5 students’ marks for some calculation. Marks are integer types, so we can declare variables something like this.
int st1, st2, st3, st4, st5
If we declare it like above, it’ll be very difficult for us to manipulate such data.
What if students are increasing, then it is very difficult to declare numerous variables and keep track of them.
To overcome this kind of situation, we have arrays. So now we can define the Arrays — used to store multiple values in a single variable of the same type, instead of declaring separate variables for each value. Or we can say it’s a collection of the same type of data in a single variable.
To declare an array:
- Define the variable type (array type)
- Specify the name of the array followed by square brackets
- Specify the number of elements it should store in between square brackets
For example:
- int marks[10]; (int type)
- string names[20]; (string type)
- double prices[15]; (double type)
- char letters[5]; (char type)
Initializing the Array
Initializing the Array during the declaration
Initializing the array during declaration means with the time of declaration array we initialize the array elements or values. How can we do that:
int marks[5] = { 80, 78, 92, 97, 86 };
Here, we place the value of 5 students marks in an array at the time of declaration.
Assigning the values after the declaration
Sometimes we declare the array without initializing elements like this.
int marks[5];
But for initializing the array, we place the value to the index number.
- marks[0] = 80;
- marks[1] = 78;
- marks[2] = 92;
- marks[3] = 97;
- marks[4] = 86;
Note: 0,1,2,3,4 are the index numbers that start from 0.
Few things to remember about array:
- The array indices start with 0. Meaning arr[0] is the first element stored at index 0.
- If the size of an array is N, the last element is stored at index (N-1). In this example, marks[4] is the last element.
- Elements of an array have consecutive addresses. For example, suppose the starting address of marks[0] is 8484. Then, the address of the next element marks[1] will be 8488, the address of marks[2] will be 8492, and so on.
- Here, the size of each element is increased by 4. This is because the size of int is 4 bytes.
- If we forget to declare the size of an array during initialisation then compiler automatically assigns the size of an array.
Let’s discuss about types of array in C++
Basically, there are two types of array in C++:
- One dimensional array
- Multi dimensional array
One dimensional array — in this type of array, the elements are stored in a single dimension or we can say single row.
Example: int bikes[20]
Multi dimensional array — in this type of array, the elements are stored in multi dimensions row and column wise. Two indices describe each element, the first index represents a row, and the second index represents a column.
Example: int cars[10][20], int rooms[5][10][15]
Manipulating the Array
Accessing the Elements of an Array
Each element in an array is associated with an index number. We can access the elements of an array by using those indices.
Like: cout<<arr[0];
This will print the first element of an array.
How to print whole Array
For printing all elements of an array, we’ll use loops to print the elements of an array.
There are many ways to print the whole array elements. Here we’re using a for-each loop to print elements.
int arr[3] = { 8, 7, 9 }; for (int i: arr) { cout << i << " "; } //Output: 8 7 9
How to check if the index number goes to out of range
Let’s suppose we’ve an array of size 10. So what if someone wants to access the value at index 12. So here we need to first check the range of the index whether it’s in range or not.
int arr[] = { 1, 2, 3, 4, 5 }; int sizeOfArray = sizeof(arr) / sizeof(int); int index = 20; if (index < 0 || index > sizeOfArray) { count << "index out of range"; }
Array operations in C++
- Traversal
- Insertion
- Search
- Updation
- Deletion
Let’s discuss one-by-one
1: Traversal — Traversal in an array means printing the elements of the array one by one. Means we’re accessing the elements of the array. This can done using a simple code:
int main() { int arr[3] = { 1, 3, 2 }; for (int i = 0; i < 3; i++) { cout << arr[i] << endl; } return 0; }
2: Insertion —
Insertion of element in the end of the Array
Now we want to add some extra values to an existing array. In the above array we want to add 6 and 7. How to do this?
int arr[] = { 1, 2, 3, 4, 5 }; // Here last index is 4 so we've to add extra value to next indexarr[5] = 6; arr[6] = 7; // We can add more values to an array
Insertion of element at the specific Index
- First get the element to be inserted, let say elem
- Then get the position at which this element is to be inserted, let say position
- Then shift the array elements from this position to one position forward, and do this for all the other elements next to position.
- Insert the element elem now at the position position.
int arr[] = { 1, 2, 3, 4, 5 }; int i; int sizeOfArray = sizeof(arr) / sizeof(int); int position = 3, elem = 8; cout << sizeOfArray << endl; for (i = sizeOfArray; i >= position; i--) arr[i] = arr[i - 1]; arr[i] = elem; sizeOfArray++; for (int j; j < sizeOfArray; j++) { cout << arr[j]; }
In the above code we want to add element 8 at index number 3. Let’s understand this code in detail.
Here,
arr[0] = 1;
arr[1] = 2;
arr[2] = 3;
arr[3] = 4;
arr[4] = 5;
The size of the array is 5.
Inside the first for loop:
- i = 5
- Now the condition, i >= position or 5 >= 3 evaluates to be true, therefore program flow goes inside the loop
- Inside the loop, arr[i-1] or arr[5-1] or arr[4] or 5 gets initialised to arr[i] or arr[5]. So arr[5] = 5.
- Now the program flow goes to the update part of the for loop decrements the value of i. So i=4, and evaluates the condition again
- That is the condition i >= position or 4>=3 evaluates to be true again, therefore program flow again goes inside the loop
- This process continues, until the condition of the for loop evaluates to be false. Before its condition evaluated to be false, here are the new values of arr[]:
arr[5] = 5;
arr[4] = 4;
- Now 4 element is placed on the 4th index that was placed on the 3rd index.
- Now the 3rd index is free to placed new element.
Check if an element is in Array
3: Search — Now we’re going to search a specific element in the array. For that we need to ask the user to enter the element that you want to search in the array. Then traverse the whole array and check the entered value is matched with any element in the array. Let’s understand with an example.
int main() { int arr[3] = { 25, 76, 63 }; int userInput; cin >> userInput; for (int i: arr) { if (i == userInput) { cout << "Found"; } else { cout << "Not found"; break; } } return 0; }
How to change or update the elements
4: Updation — Updating refers to updating an existing array element at a particular index value to a new element.
int main() { int arr[4] = { 10, 20, 30, 50 }; int pos = 4; int newElem = 40; cout << "Original array :" << endl; for (int i = 0; i < 4; i++) { cout << arr[i] << endl; } arr[pos - 1] = newElem; cout << "after updating the array :" << endl; for (int i = 0; i < 4; i++) { cout << arr[i] << endl; } return 0; }
5: Deletion — Deletion operation is used to delete an element from the array. A simple program to perform deletion in an array is shown below.
int main() { int arr[] = { 1, 2, 3, 4 }; int pos = 2, size = 4; int j = pos; while (j < size) { arr[j - 1] = arr[j]; j = j + 1; } size = size - 1; for (int i = 0; i < size; i++) { cout << arr[i] << endl; } return 0; }
What are Empty Arrays?
Empty arrays means those arrays have no elements. How can we declare empty arrays? There are some ways to declare empty arrays.
For example:
int arr[10]; int arr[10] = {}; int arr[50] = { 0 };
Creating the Array using loop
By using the loop, we’ll create the arrays and initialise the array with user input.
To fill an array with user input. We need to ask the user to enter the size of the array then the elements of an array. Let’s understand with an example.
int main() { int size; cin >> size; int arr[size]; for (int i = 0; i < size; i++) { int j; cin >> j; arr[i] = j; } for (int k: arr) { cout << k << endl; }
How to know the size or length of an array?
Basically, when we say the length of an array actually we refer to the total number of elements present in the array.
For example:
int books[8] = { 1, 2, 3, 4, 5, 6, 7, 8 };
Here we can count the total number of elements in the array. But what if there are many more elements?
We’re going to learn about the various ways to find the array length.
Ways to find Length of an Array
- Counting element-by-element
- begin() and end() function
- sizeof() function
- Pointers.
Let’s understand one by one:
Counting element-by-element
In this method we’ll traverse all the elements of an array and simultaneously we’ll count the elements. Let’s understand with an example.
int main() { int count; int arr[] = { 1, 2, 3, 4, 5, 6, 7, 8 }; for (int i: arr) { cout << i << endl; count++; } cout << "The length of the array is: " << count; return 0; }
Using begin() and end() function
We can also calculate the length of an array using the standard library’s begin() and end() functions. See below code.
int main() { int count; int arr[] = { 1, 2, 3, 4, 5, 6, 7, 8 }; count = end(arr) - begin(arr); cout << "The length of the array is: " << count; return 0; }
By using sizeof() operator
The sizeof() operator returns the size of the passed variable or data in bytes. But for finding total numbers of elements in an array we divide the whole bytes with the size of data type of an array. See below example.
int main() { int arr[] = { 10, 20, 30, 40, 50, 60, 70, 80 }; int count = sizeof(arr) / sizeof(int); cout << "The length of the array is: " << count; return 0; }
Find the array length using pointer
By using pointer
We can also calculate the length of an array by using a pointer. See example:
int main() { int arr[] = { 8, 6, 9, 5, 3, 5 }; int count = * ( & arr + 1) - arr; cout << "The length of the array is: " << count; return 0; }
Sometimes we need to check the array whether it’s full or not?
Full array means all the elements are filled with equal to the array size. For example an array size is 20 then it’ll be called full array when the total number of elements are 20.
So how to check with code?
int main() { int arr[2] = { 1, 2 }; int count = 0; int size = sizeof(arr) / sizeof(int); for (int i: arr) { if (arr[i] != 0) count++; } if (count == size) cout << "Array is full"; else cout << "Array isn't full"; return 0; }
Array in Classes or Array of objects
When a class is defined, only the specification for the object is defined; no memory or storage is allocated. To use the data and access functions defined in the class, you need to create objects of class type. Let’s have a look at the code.
class emp { public: int id; string name; void get() { cin >> id >> name; } void show() { cout << id << " " << name << endl; } }; int main() { emp e[2]; for (int j = 0; j < 2; j++) { e[j].get(); } for (int j = 0; j < 2; j++) { e[j].show(); } return 0; }
Here we created an emp class with a public member ( id type of int, name type of string ) two member functions: get() for getting employee details and show() for printing the details of employees.
Next we created an array of type class in the main function.
Passing Array to a function
Just like normal variables, Arrays can also be passed to a function as an argument of a function, but in C++ whenever we pass an array as a function argument then it is always treated as a pointer by a function. It means a function argument can never be an array – they’re always converted to the pointers.
Basically there are three ways to pass an array to a function argument.
- Formal parameter as a sized array
- Formal parameter as a unsized array
- Formal parameter as a pointers
Let’s understand one-by-one:
1. Formal parameter as a sized array —
#include<iostream>using namespace std;int sum(int []); int main(){ int arr[3] = {2, 3, 4}; cout<<sum(arr); return 0;} int sum(int array[3]) { // here we pass the array with size int total =0; for(int i=0;i<3;i++){ total += array[i]; } return total;}
Even though the parameter array is declared as an int array of 3 elements, the compiler automatically converts it to an int pointer like this int *array. This is necessary because no parameter can actually receive an entire array. A pointer to an array gets passed when an array is passed to the function.
2. Formal parameter as a unsized array —
#include<iostream>using namespace std;int sum(int [],int);int main(){int arr[3] = {2, 3, 4}; int size = 3; cout<<sum(arr,size); // we pass the array as well size of the array as an argument return 0;} int sum(int array[3], int n) { int total = 0; for (int i = 0; i < n; i++) { total += array[i]; } return total; }
In this method, we pass the array with the size of an array as an argument.
3 Formal parameter as a pointer —
#include<iostream>using namespace std;int sum(int [],int);int main(){ int arr[3] = {2, 3, 4}; int size = 3; cout<<sum(arr,size); // we pass the array as well size of the array as an argument return 0;} int sum(int * array, int n) { int total = 0; for (int i = 0; i < n; i++) { total += array[i]; } return total; }
Here we’re not using square brackets because the function argument can’t be an array – they’re always converted to the pointers. So here we directly used pointers.